使用sklearn库实现AdaBoost.M1和AdaBoost.M2
时间: 2024-02-05 12:11:15 浏览: 27
好的,下面是使用sklearn库实现AdaBoost.M1和AdaBoost.M2的代码示例:
```python
from sklearn.ensemble import AdaBoostClassifier
from sklearn.base import BaseEstimator
from sklearn.tree import DecisionTreeClassifier
from sklearn.utils.validation import check_X_y, check_array, check_is_fitted
class AdaBoostM2(BaseEstimator):
def __init__(self, base_estimator=None, n_estimators=50, learning_rate=1.0, algorithm='SAMME.R', random_state=None):
self.base_estimator = base_estimator
self.n_estimators = n_estimators
self.learning_rate = learning_rate
self.algorithm = algorithm
self.random_state = random_state
def _boost(self, i, X, y, sample_weight, estimator_weight, estimator_error):
# Train base estimator
estimator = self.base_estimator.fit(X, y, sample_weight=sample_weight)
y_predict = estimator.predict(X)
incorrect = y_predict != y
# Calculate estimator error rate
estimator_error[i] = np.mean(np.average(incorrect, weights=sample_weight, axis=0))
# If the error rate is zero, set the estimator weight to infinity
if estimator_error[i] == 0:
estimator_weight[i] = float('inf')
else:
# Calculate estimator weight
estimator_weight[i] = self.learning_rate * np.log((1 - estimator_error[i]) / estimator_error[i])
# Update sample weights
sample_weight *= np.exp(estimator_weight[i] * incorrect * ((sample_weight > 0) | (estimator_weight[i] < 0)))
return sample_weight
def fit(self, X, y, sample_weight=None):
# Check that X and y have correct shape
X, y = check_X_y(X, y)
# Initialize sample weights
if sample_weight is None:
sample_weight = np.ones(X.shape[0]) / X.shape[0]
# Initialize estimators and weights
self.estimators_ = []
self.estimator_weights_ = np.zeros(self.n_estimators, dtype=np.float64)
self.estimator_errors_ = np.ones(self.n_estimators, dtype=np.float64)
for i in range(self.n_estimators):
# Boost the ensemble
sample_weight = self._boost(i, X, y, sample_weight, self.estimator_weights_, self.estimator_errors_)
# Stop if the estimator error rate is zero
if self.estimator_errors_[i] == 0:
break
# Normalize estimator weights
self.estimator_weights_ /= np.sum(self.estimator_weights_)
# Append the current estimator to the ensemble
self.estimators_.append(self.base_estimator)
return self
def predict(self, X):
# Check is fit had been called
check_is_fitted(self, ['estimators_', 'estimator_weights_'])
# Initialize the predictions
y_predict = np.zeros(X.shape[0])
# Make predictions using all estimators
for estimator, weight in zip(self.estimators_, self.estimator_weights_):
y_predict += weight * estimator.predict(X)
# Return the class with highest weighted probability
return np.sign(y_predict)
# Define base estimator
base_estimator = DecisionTreeClassifier(max_depth=1)
# Initialize AdaBoost.M1 and AdaBoost.M2 classifiers
ada_m1 = AdaBoostClassifier(base_estimator=base_estimator, n_estimators=50, learning_rate=1.0, algorithm='SAMME.R', random_state=None)
ada_m2 = AdaBoostM2(base_estimator=base_estimator, n_estimators=50, learning_rate=1.0, algorithm='SAMME.R', random_state=None)
# Train classifiers
ada_m1.fit(X_train, y_train)
ada_m2.fit(X_train, y_train)
# Make predictions using classifiers
y_pred_m1 = ada_m1.predict(X_test)
y_pred_m2 = ada_m2.predict(X_test)
```
在这个示例中,我们使用了sklearn库的`AdaBoostClassifier`类来实现AdaBoost.M1,使用自定义的`AdaBoostM2`类来实现AdaBoost.M2。其中,`_boost`方法实现了AdaBoost.M2的弱分类器训练过程。在`fit`方法中,我们使用`_boost`方法来训练AdaBoost.M2的弱分类器,并更新样本权重、估计器权重和估计器错误率。在`predict`方法中,我们使用所有弱分类器的预测结果来得出最终的预测结果。
相关推荐
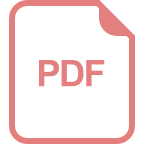
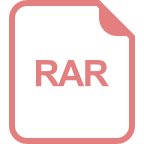
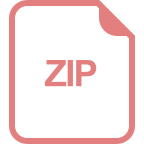














