怎么创建dll可以在c#和c++工程中都可以被调用,如何调用
时间: 2024-02-28 11:56:14 浏览: 19
要创建一个可以在 C# 和 C++ 工程中都可以被调用的 DLL,可以使用 C++ 语言编写 DLL,并将其包装在一个 C++/CLI 封装器中。这个封装器将 C++ DLL 中的函数和类包装成 .NET 对象,使其可以在 C# 中使用。
下面是创建和调用这种 DLL 的一些步骤:
1. 使用 C++ 编写 DLL,并导出需要在 C# 和 C++ 中使用的函数和类。这可以使用 __declspec(dllexport) 关键字来完成。
2. 创建一个 C++/CLI 封装器工程,并将 C++ DLL 添加到该工程中。在封装器工程中,可以使用 #include 指令包含 C++ DLL 的头文件,并使用 using namespace 指令导入需要的命名空间。
3. 在 C++/CLI 封装器中,将需要在 C# 中使用的函数和类包装成公共 .NET 类,并将其导出为 DLL。这可以使用 __declspec(dllexport) 和 public 关键字来完成。
4. 在 C# 中,使用 DllImport 属性和 extern 关键字声明需要调用的 C++/CLI 封装器中的函数和类。在 C# 中,可以像调用任何其他 .NET 对象一样调用这些函数和类。
下面是一个简单的示例,演示如何创建并调用一个可以在 C# 和 C++ 中使用的 DLL:
C++ DLL 文件 "MyDll.h":
```
__declspec(dllexport) int Add(int a, int b);
```
C++ DLL 文件 "MyDll.cpp":
```
int Add(int a, int b)
{
return a + b;
}
```
C++/CLI 封装器文件 "MyWrapper.h":
```
#pragma once
using namespace System;
namespace MyWrapper
{
public ref class MyDll
{
public:
static int Add(int a, int b);
};
}
```
C++/CLI 封装器文件 "MyWrapper.cpp":
```
#include "MyDll.h"
#include "MyWrapper.h"
namespace MyWrapper
{
int MyDll::Add(int a, int b)
{
return ::Add(a, b);
}
}
```
C# 文件 "Program.cs":
```
using System;
using System.Runtime.InteropServices;
using MyWrapper;
class Program
{
[DllImport("MyWrapper.dll")]
public static extern int Add(int a, int b);
static void Main(string[] args)
{
int result = Add(1, 2);
Console.WriteLine(result);
MyDll myDll = new MyDll();
int result2 = myDll.Add(3, 4);
Console.WriteLine(result2);
}
}
```
在上面的示例中,我们创建了一个 C++ DLL,其中包含了一个 Add 函数。然后,我们创建了一个 C++/CLI 封装器,将 Add 函数包装成了一个公共 .NET 类。最后,我们在 C# 中声明了一个 DllImport 函数,用于调用封装器中的 Add 函数,以及使用封装器类创建了一个对象并调用了其中的 Add 函数。
相关推荐
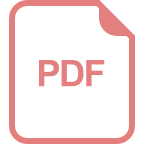














