C#一个图标随机出现在几个不同的位置,随机移动,点击图标则显示图标的另一种形态,并加上音效和背景音乐
时间: 2024-03-16 22:48:13 浏览: 38
设计思路:
1. 在窗体中添加一个 PictureBox 控件,用来显示图标的图片。
2. 使用 Timer 控件来定时随机生成图标的位置,并且控制图标的移动。
3. 在 PictureBox 上添加 MouseClick 事件,当玩家点击图标时,判断是否击中,然后更新图标的图片。
4. 使用 SoundPlayer 控件来播放背景音乐和音效。
代码实现:
1. 在窗体中添加一个 PictureBox 控件,用来显示图标的图片。
```csharp
private PictureBox iconPicBox = new PictureBox();
```
2. 使用 Timer 控件来定时随机生成图标的位置,并且控制图标的移动。
```csharp
private Timer iconTimer = new Timer();
private int iconWidth = 80; // 图标的宽度
private int iconHeight = 80; // 图标的高度
private void IconTimer_Tick(object sender, EventArgs e)
{
// 随机生成图标的位置
Random random = new Random();
int x = random.Next(pictureBox1.Width - iconWidth);
int y = random.Next(pictureBox1.Height - iconHeight);
iconPicBox.Location = new Point(x, y);
// 随机生成图标的移动方向和速度
int dx = random.Next(-5, 6); // x 方向的速度
int dy = random.Next(-5, 6); // y 方向的速度
while (dx == 0 && dy == 0) // 防止速度为零
{
dx = random.Next(-5, 6);
dy = random.Next(-5, 6);
}
iconTimer.Tag = new Point(dx, dy); // 将速度保存在 Tag 属性中
}
private void IconTimer_Move(object sender, EventArgs e)
{
// 控制图标的移动
Point speed = (Point)iconTimer.Tag;
int x = iconPicBox.Location.X + speed.X;
int y = iconPicBox.Location.Y + speed.Y;
// 判断是否超出窗口边界
if (x < 0 || x > pictureBox1.Width - iconWidth)
{
speed.X = -speed.X;
x = iconPicBox.Location.X + speed.X;
}
if (y < 0 || y > pictureBox1.Height - iconHeight)
{
speed.Y = -speed.Y;
y = iconPicBox.Location.Y + speed.Y;
}
// 更新图标的位置
iconPicBox.Location = new Point(x, y);
}
```
3. 在 PictureBox 上添加 MouseClick 事件,当玩家点击图标时,判断是否击中,然后更新图标的图片。
```csharp
private SoundPlayer hitSoundPlayer = new SoundPlayer(Properties.Resources.hitSound);
private void IconPicBox_MouseClick(object sender, MouseEventArgs e)
{
// 判断是否击中图标
if (e.X < iconWidth && e.Y < iconHeight)
{
iconPicBox.Image = Properties.Resources.icon2; // 更新图标的图片
hitSoundPlayer.Play(); // 播放击中音效
}
}
```
4. 使用 SoundPlayer 控件来播放背景音乐和音效。
```csharp
private SoundPlayer bgSoundPlayer = new SoundPlayer(Properties.Resources.bgSound);
private void MainForm_Load(object sender, EventArgs e)
{
// 播放背景音乐
bgSoundPlayer.PlayLooping();
}
```
完整代码如下:
相关推荐
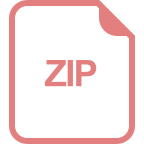
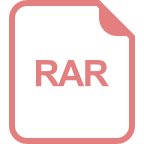
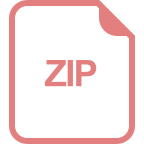














