1064, 'pattern:yyyy-MM-dd HH:mm:ss msg:Invalid format: "0
时间: 2023-10-02 21:10:39 浏览: 53
The error message indicates that there is an issue with the format of the input string "0 ". The expected format is "yyyy-MM-dd HH:mm:ss". It seems that the input string does not conform to this format, hence the "Invalid format" error. It is possible that there is a missing or extra character in the input string, or the input string is not in the correct format. More information about the input string and the expected format is needed to determine the exact cause of the error.
相关问题
qt把字符串转换成"yyyy-MM-dd hh:mm:ss"
在 Qt 中,你可以使用 QDateTime 类来将字符串转换为指定格式的日期和时间。对于将字符串转换为 "yyyy-MM-dd hh:mm:ss" 格式的日期和时间,你可以按照以下方式操作:
```cpp
#include <QCoreApplication>
#include <QDateTime>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QString dateTimeString = "2022-01-01 12:34:56";
QDateTime dateTime = QDateTime::fromString(dateTimeString, "yyyy-MM-dd hh:mm:ss");
if (dateTime.isValid()) {
QString formattedDateTime = dateTime.toString("yyyy-MM-dd hh:mm:ss");
qDebug() << "Formatted DateTime:" << formattedDateTime;
} else {
qDebug() << "Invalid DateTime";
}
return a.exec();
}
```
在上面的示例中,首先使用 `fromString` 函数将字符串 `dateTimeString` 解析为 QDateTime 对象。解析过程中,我们指定了输入字符串的格式为 "yyyy-MM-dd hh:mm:ss"。然后,我们检查 QDateTime 对象是否有效。如果有效,我们使用 `toString` 函数将日期和时间格式化为 "yyyy-MM-dd hh:mm:ss" 的字符串,并进行输出。如果无效,则输出 "Invalid DateTime"。
请注意,在使用该代码之前,确保已经包含了相关的 Qt 模块和头文件。
@JsonDeserialize(using = DateDeserializer.class) 能动态转换yyyy-MM-dd HH:mm:ss或者yyyy-MM-dd吗?
是的,可以使用自定义的`DateDeserializer`类来实现动态转换不同格式的日期时间字符串。在`DateDeserializer`类中,您可以根据输入的日期时间字符串的格式,使用不同的日期时间格式化器来解析日期时间,并将其转换为`Date`对象。例如,您可以按照以下方式实现`DateDeserializer`类:
```java
public class DateDeserializer extends JsonDeserializer<Date> {
@Override
public Date deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException, JsonProcessingException {
String dateString = jsonParser.getText();
SimpleDateFormat[] formats = new SimpleDateFormat[] {
new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"),
new SimpleDateFormat("yyyy-MM-dd")
};
for (SimpleDateFormat format : formats) {
try {
return format.parse(dateString);
} catch (ParseException e) {
// ignore and try next format
}
}
throw new IllegalArgumentException("Invalid date format: " + dateString);
}
}
```
在上面的示例中,我们定义了两种日期时间格式:`yyyy-MM-dd HH:mm:ss`和`yyyy-MM-dd`。我们使用一个循环来尝试每种格式,直到找到可以解析日期时间字符串的格式为止。如果没有找到,则抛出一个异常。然后,您可以将`@JsonDeserialize`注解与`Date`类型的属性一起使用,以指定要使用的自定义反序列化器,例如:
```java
public class MyObject {
@JsonDeserialize(using = DateDeserializer.class)
private Date date;
// other fields and methods
}
```
这样,在反序列化JSON字符串时,Jackson将使用`DateDeserializer`类来解析并转换日期时间字符串。
相关推荐
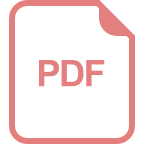
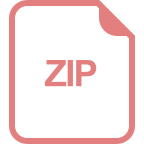
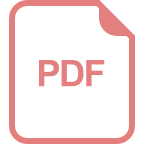












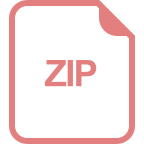