解释下面的代码: for root, dirs, files in os.walk(folder_path): for file_name in files:
时间: 2024-05-22 20:15:00 浏览: 161
这段代码使用Python中的os模块中的walk函数,用于遍历指定文件夹及其子文件夹中的所有文件和文件夹。os.walk会返回3个值,分别是当前遍历到的文件夹路径root、当前文件夹下的目录列表dirs、当前文件夹下的文件列表files。这个for循环中,我们只需要遍历所有的文件,因此针对files列表进行遍历,对于其中的每个文件名file_name,我们可以进行进一步的操作。
相关问题
解释这段代码import os import multiprocessing as mp def count_letters(file_path, letter_counts): with open(file_path, 'r') as f: for line in f: for letter in line: if letter.isalpha(): letter_counts[letter.lower()] += 1 def process_folder(folder_path, letter_counts): for root, dirs, files in os.walk(folder_path): for name in files: if name.endswith('.txt'): file_path = os.path.join(root, name) count_letters(file_path, letter_counts) if __name__ == '__main__': folder_path = 'D:\\pachong\\并行\\blogs\\blogs' num_processes = mp.cpu_count() # 使用所有可用的 CPU 核心数 letter_counts = mp.Manager().dict({letter: 0 for letter in 'abcdefghijklmnopqrstuvwxyz'}) processes = [] for i in range(num_processes): p = mp.Process(target=process_folder, args=(folder_path, letter_counts)) p.start() processes.append(p) for p in processes: p.join() with open('letter_counts.txt', 'w') as f: for letter, count in letter_counts.items(): f.write(f'{letter}: {count}\n')
这段代码使用了 Python 的 multiprocessing 模块,实现了一个多进程的字母计数器。具体来说,代码首先定义了两个函数:
- count_letters(file_path, letter_counts):用于统计文件中每个字母出现的次数,其中 file_path 是文件路径,letter_counts 是一个字典,用于记录每个字母的出现次数。
- process_folder(folder_path, letter_counts):用于遍历目录中的所有文件,并对每个文件调用 count_letters 函数。
在主程序部分,代码首先获取了待处理的文件夹路径和可用的 CPU 核心数。然后,代码创建了一个字典 letter_counts,用于记录每个字母的出现次数,并使用 multiprocessing 模块的 Manager 类将其转换为共享字典,以便多个进程可以同时访问。接下来,代码创建了多个进程,并将它们分别赋予不同的任务。最后,代码等待所有进程完成后,将统计结果写入文件 letter_counts.txt。
需要注意的是,由于 multiprocessing 模块中的进程之间无法直接共享变量,因此这里使用了 Manager 类创建了一个共享字典。此外,为了避免多个进程同时写入同一个文件而导致的冲突,代码将文件写入操作放在了主进程中。
import os import pandas as pd import time while True: start = time.perf_counter() def get_txt_files(folder_path): files = [] try: for root, dirs, filenames in os.walk(folder_path): for filename in filenames: if filename.endswith(".txt"): files.append(os.path.abspath(os.path.join(root, filename))) except Exception as e: print("Error: {}".format(e)) return files def combine_txt_files(txt_files): content = "" try: for txt_file in txt_files: with open(txt_file, "r") as f: content += f.read() except Exception as e: print("Error: {}".format(e)) return content def write_combined_content_to_file(content, file_path): try: with open(file_path, "w") as f: f.write(content) except Exception as e: print("Error: {}".format(e)) def txt_to_excel(txt_file, excel_file): try: df = pd.read_csv(txt_file, delimiter="\t", header=None) df.to_excel(excel_file, index=False, header=None) except Exception as e: print("Error: {}".format(e)) if __name__=="__main__": folder_path="Z:\看板v2" txt_files=get_txt_files(folder_path) combined_content=combine_txt_files(txt_files) combined_file_path="D:\\測試log\\TXT合1并.txt" write_combined_content_to_file(combined_content,combined_file_path) excel_file_path="D:\\測試log\\123.xlsx" txt_to_excel(combined_file_path,excel_file_path) end = time.perf_counter() print('運行時間為:{}秒'.format(end-start)) time.sleep(30)
这是一段 Python 代码,主要功能是将指定文件夹中的所有 .txt 文件合并为一个文件,并将合并后的内容转换为 Excel 文件。
首先使用 `os.walk` 函数获取指定文件夹中所有的 .txt 文件路径,然后使用 `open` 函数读取每个文件的内容,并将所有内容合并为一个字符串。接着使用 `open` 函数将合并后的字符串写入到指定路径的文件中。最后使用 Pandas 库中的 `read_csv` 函数读取合并后的文本文件,并使用 `to_excel` 函数将其转换为 Excel 文件。
代码中使用了一个死循环,每隔 30 秒就会执行一次上述操作。遇到任何异常都会输出错误信息。最后会输出每次操作的运行时间。
阅读全文
相关推荐
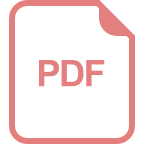
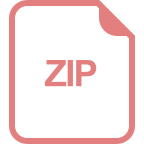
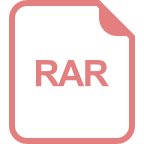









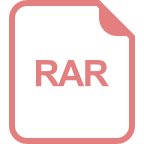
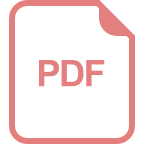
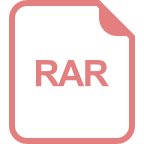