import os import pandas as pd import time while True: start = time.perf_counter() def get_txt_files(folder_path): files = [] try: for root, dirs, filenames in os.walk(folder_path): for filename in filenames: if filename.endswith(".txt"): files.append(os.path.abspath(os.path.join(root, filename))) except Exception as e: print("Error: {}".format(e)) return files def combine_txt_files(txt_files): content = "" try: for txt_file in txt_files: with open(txt_file, "r") as f: content += f.read() except Exception as e: print("Error: {}".format(e)) return content def write_combined_content_to_file(content, file_path): try: with open(file_path, "w") as f: f.write(content) except Exception as e: print("Error: {}".format(e)) def txt_to_excel(txt_file, excel_file): try: df = pd.read_csv(txt_file, delimiter="\t", header=None) df.to_excel(excel_file, index=False, header=None) except Exception as e: print("Error: {}".format(e)) if __name__=="__main__": folder_path="Z:\看板v2" txt_files=get_txt_files(folder_path) combined_content=combine_txt_files(txt_files) combined_file_path="D:\\測試log\\TXT合1并.txt" write_combined_content_to_file(combined_content,combined_file_path) excel_file_path="D:\\測試log\\123.xlsx" txt_to_excel(combined_file_path,excel_file_path) end = time.perf_counter() print('運行時間為:{}秒'.format(end-start)) time.sleep(30)
时间: 2024-02-14 11:32:49 浏览: 24
这是一段 Python 代码,主要功能是将指定文件夹中的所有 .txt 文件合并为一个文件,并将合并后的内容转换为 Excel 文件。
首先使用 `os.walk` 函数获取指定文件夹中所有的 .txt 文件路径,然后使用 `open` 函数读取每个文件的内容,并将所有内容合并为一个字符串。接着使用 `open` 函数将合并后的字符串写入到指定路径的文件中。最后使用 Pandas 库中的 `read_csv` 函数读取合并后的文本文件,并使用 `to_excel` 函数将其转换为 Excel 文件。
代码中使用了一个死循环,每隔 30 秒就会执行一次上述操作。遇到任何异常都会输出错误信息。最后会输出每次操作的运行时间。
相关问题
import os import pandas as pd from openpyxl import Workbook folder_path = r'D:/拆分后的工作簿' new_path = r'D:\拆分后的工作簿\新增資料夾\workbook.xlsx' files = os.listdir(folder_path) wb = Workbook() for file in files: if file.endswith('.xlsx'): file_path = os.path.join(folder_path, file) sheet_name = os.path.splitext(file)[0] df = pd.read_excel(file_path, sheet_name=None) for sheet in df: df[sheet].to_excel(wb, sheet_name=sheet_name)
这段代码看起来没有问题,不过你最后没有保存工作簿。你需要将以下代码添加到末尾:
```python
wb.save(new_path)
```
这将工作簿保存到指定的路径下。完整代码如下:
```python
import os
import pandas as pd
from openpyxl import Workbook
folder_path = r'D:/拆分后的工作簿'
new_path = r'D:\拆分后的工作簿\新增資料夾\workbook.xlsx'
files = os.listdir(folder_path)
wb = Workbook()
for file in files:
if file.endswith('.xlsx'):
file_path = os.path.join(folder_path, file)
sheet_name = os.path.splitext(file)[0]
df = pd.read_excel(file_path, sheet_name=None)
for sheet in df:
df[sheet].to_excel(wb, sheet_name=sheet_name)
wb.save(new_path)
```
希望这次能够帮到你!
import pandas as pd , data=pd.read_csv()
import pandas as pd是Python中导入pandas库的常用语句,它将pandas库导入并使用别名pd来简化代码书写。
data=pd.read_csv()是pandas库中的一个函数,用于读取CSV文件并将其转换为DataFrame对象。CSV文件是一种常见的以逗号分隔值的文件格式,用于存储表格数据。
在使用data=pd.read_csv()时,你需要在括号中指定CSV文件的路径或URL,并将读取到的数据赋值给变量data。这样就可以通过data来访问和操作读取到的数据了。
相关推荐
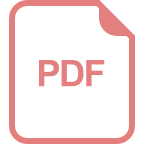
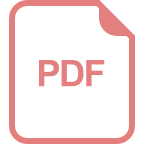













