购买地铁车票的规定如下: 乘1-4站,3元/位;乘5-9站,4元/位;乘9站以上,5元/位。输入人数、站数,输出应付款。 请用C语言编写程序实现
时间: 2023-09-03 11:27:28 浏览: 73
### 回答1:
以下是使用 C 语言编写的程序:
```c
#include <stdio.h>
int main() {
int people, stations, price;
printf("请输入乘车人数和站数:\n");
scanf("%d%d", &people, &stations);
if (stations <= 0) {
printf("站数不能小于等于 0!\n");
return 1;
}
else if (people <= 0) {
printf("人数不能小于等于 0!\n");
return 1;
}
else if (stations <= 4) {
price = people * 3;
}
else if (stations <= 9) {
price = people * 4;
}
else {
price = people * 5;
}
printf("应付款为:%d 元\n", price);
return 0;
}
```
程序运行结果示例:
```
请输入乘车人数和站数:
3 2
应付款为:9 元
```
### 回答2:
以下是使用C语言编写的实现程序:
```c
#include <stdio.h>
int main() {
int peopleCount, stationCount;
int totalPrice = 0;
printf("请输入人数和站数:");
scanf("%d %d", &peopleCount, &stationCount);
if (stationCount >= 1 && stationCount <= 4) {
totalPrice = peopleCount * 3;
} else if (stationCount >= 5 && stationCount <= 9) {
totalPrice = peopleCount * 4;
} else if (stationCount >= 10) {
totalPrice = peopleCount * 5;
} else {
printf("站数无效!");
return 0;
}
printf("应付款:%d元\n", totalPrice);
return 0;
}
```
运行程序时,用户需要输入人数和站数,程序将根据规定计算并输出应付款金额。如果输入的站数无效(小于1或大于9),程序将输出"站数无效!"。
希望可以帮到你!
### 回答3:
以下是用C语言编写的程序实现:
#include <stdio.h>
int main() {
int people, stations;
int price;
printf("请输入人数:");
scanf("%d", &people);
printf("请输入站数:");
scanf("%d", &stations);
if (stations >= 1 && stations <= 4) {
price = 3;
}
else if (stations >= 5 && stations <= 9) {
price = 4;
}
else if (stations > 9) {
price = 5;
}
else {
printf("输入站数有误!\n");
return 0;
}
int total_price = people * price;
printf("应付款:%d元\n", total_price);
return 0;
}
运行程序后,程序会要求输入人数和站数。根据所输入的站数不同,程序会计算出每位乘客应付的车票价格,并最终计算出应付款总额。最后输出总额。
相关推荐
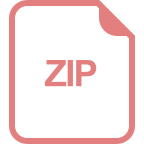
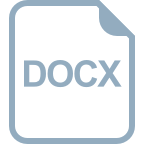
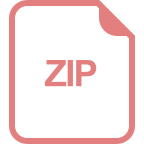








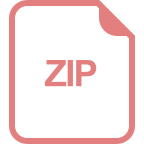
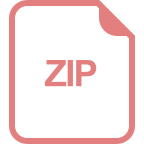
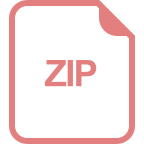
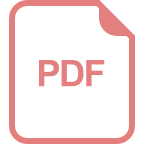
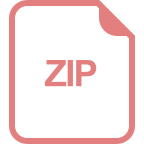
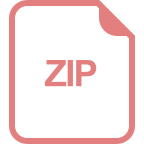
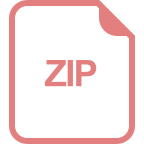