使用C语言编写一段linux操作系统可以使用的优先级调度算法
时间: 2024-03-26 12:36:15 浏览: 55
好的,我可以提供一种常见的优先级调度算法,它叫做优先级抢占调度算法(Priority Preemptive Scheduling Algorithm),使用C语言实现也比较容易。
以下是一份示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESSES 100
struct process {
int pid; // 进程ID
int priority; // 优先级,越小越高
int burst_time; // 执行时间
int remaining_time; // 剩余执行时间
};
int num_processes;
struct process processes[MAX_PROCESSES];
// 比较函数,用于排序
int cmp(const void *a, const void *b) {
struct process *pa = (struct process *)a;
struct process *pb = (struct process *)b;
return pa->priority - pb->priority;
}
// 优先级抢占调度算法
void priority_preemptive_scheduling() {
int current_time = 0;
int completed = 0;
// 按照优先级排序
qsort(processes, num_processes, sizeof(struct process), cmp);
// 执行进程
while (completed < num_processes) {
int next_process = -1;
int highest_priority = MAX_PROCESSES + 1;
// 查找下一个要执行的进程
for (int i = 0; i < num_processes; i++) {
if (processes[i].remaining_time > 0 && processes[i].priority < highest_priority && processes[i].burst_time + current_time >= processes[next_process].burst_time + current_time) {
next_process = i;
highest_priority = processes[i].priority;
}
}
// 执行进程
printf("Time %d: Process %d\n", current_time, processes[next_process].pid);
processes[next_process].remaining_time--;
current_time++;
// 完成进程
if (processes[next_process].remaining_time == 0) {
completed++;
}
}
}
int main() {
// 输入进程信息
printf("Enter the number of processes: ");
scanf("%d", &num_processes);
for (int i = 0; i < num_processes; i++) {
printf("Enter the priority and burst time of process %d: ", i + 1);
scanf("%d%d", &processes[i].priority, &processes[i].burst_time);
processes[i].pid = i + 1;
processes[i].remaining_time = processes[i].burst_time;
}
// 执行调度算法
priority_preemptive_scheduling();
return 0;
}
```
以上代码实现了一个简单的优先级抢占调度算法,并且可以输入进程信息进行测试。在实际的操作系统中,还需要考虑更多的因素,如进程状态转换、进程间通信等。
阅读全文
相关推荐
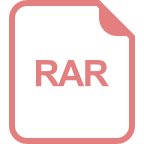















