converting 2nd argument `x' of dfitpack.curfit to C/Fortran array
时间: 2024-05-02 09:18:14 浏览: 20
As an AI language model, I do not have access to the specific code you are referring to. However, here is a general explanation of how to convert a Python list or NumPy array to a C/Fortran array.
In C, you can define an array using a pointer and allocating memory using the `malloc()` function. For example, to create a float array of size 10, you can use the following code:
```
float *my_array;
my_array = (float *) malloc(10 * sizeof(float));
```
In Fortran, you can define an array using the `dimension` attribute. For example, to create a real array of size 10, you can use the following code:
```
real :: my_array(10)
```
To convert a Python list or NumPy array to a C/Fortran array, you can use the `ctypes` library in Python. Here is an example of how to convert a NumPy array to a C array:
```
import numpy as np
import ctypes
# create a NumPy array
x = np.array([1.0, 2.0, 3.0, 4.0, 5.0])
# convert the NumPy array to a C array
x_c = x.ctypes.data_as(ctypes.POINTER(ctypes.c_double))
```
Here is an example of how to convert a NumPy array to a Fortran array:
```
import numpy as np
import ctypes
# create a NumPy array
x = np.array([1.0, 2.0, 3.0, 4.0, 5.0])
# convert the NumPy array to a Fortran array
x_fortran = np.asfortranarray(x)
x_fortran_pointer = ctypes.c_void_p(x_fortran.ctypes.data)
```
相关推荐
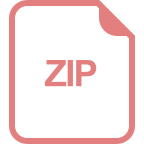
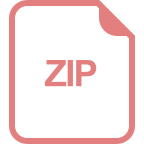














