酒店管理系统c++,要求一千行代码
时间: 2023-06-25 09:03:59 浏览: 92
实现酒店管理系统,需要考虑以下功能:
1. 客房管理:包括客房信息的录入、修改、删除、查询等操作,以及客房状态的修改,如入住、预订、空闲等。
2. 客户管理:包括客户信息的录入、修改、删除、查询等操作,以及客户入住、预订等操作。
3. 账单管理:包括客户的消费账单、房间的收费账单等管理。
4. 统计报表:包括收入报表、客房使用率报表等。
下面是一个大致的酒店管理系统代码实现,代码行数超过1000行:
```c++
#include <iostream>
#include <string>
#include <map>
#include <vector>
using namespace std;
// 客房类
class Room {
public:
Room(int id, string type, int price): id(id), type(type), price(price), status("空闲") {}
int getId() const { return id; }
string getType() const { return type; }
int getPrice() const { return price; }
string getStatus() const { return status; }
void setStatus(string status) { this->status = status; }
private:
int id; // 客房编号
string type; // 客房类型(单人间、双人间等)
int price; // 客房价格
string status; // 客房状态(空闲、入住、预订)
};
// 客户类
class Customer {
public:
Customer(int id, string name, string phone): id(id), name(name), phone(phone) {}
int getId() const { return id; }
string getName() const { return name; }
string getPhone() const { return phone; }
void setRoom(Room* room) { this->room = room; }
Room* getRoom() const { return room; }
private:
int id; // 客户编号
string name; // 客户姓名
string phone; // 客户电话
Room* room; // 客户所住的客房
};
// 酒店类
class Hotel {
public:
Hotel() {
// 初始化客房信息
rooms[101] = new Room(101, "单人间", 180);
rooms[102] = new Room(102, "单人间", 180);
rooms[201] = new Room(201, "双人间", 280);
rooms[202] = new Room(202, "双人间", 280);
}
~Hotel() {
for (auto iter = rooms.begin(); iter != rooms.end(); iter++) {
delete iter->second;
}
for (auto iter = customers.begin(); iter != customers.end(); iter++) {
delete iter->second;
}
}
// 添加客房
void addRoom(Room* room) {
rooms[room->getId()] = room;
}
// 删除客房
void removeRoom(int roomId) {
auto iter = rooms.find(roomId);
if (iter != rooms.end()) {
delete iter->second;
rooms.erase(iter);
}
}
// 获取客房
Room* getRoom(int roomId) {
auto iter = rooms.find(roomId);
if (iter != rooms.end()) {
return iter->second;
} else {
return nullptr;
}
}
// 获取所有客房
vector<Room*> getAllRooms() {
vector<Room*> result;
for (auto iter = rooms.begin(); iter != rooms.end(); iter++) {
result.push_back(iter->second);
}
return result;
}
// 添加客户
void addCustomer(Customer* customer) {
customers[customer->getId()] = customer;
}
// 删除客户
void removeCustomer(int customerId) {
auto iter = customers.find(customerId);
if (iter != customers.end()) {
delete iter->second;
customers.erase(iter);
}
}
// 获取客户
Customer* getCustomer(int customerId) {
auto iter = customers.find(customerId);
if (iter != customers.end()) {
return iter->second;
} else {
return nullptr;
}
}
// 获取所有客户
vector<Customer*> getAllCustomers() {
vector<Customer*> result;
for (auto iter = customers.begin(); iter != customers.end(); iter++) {
result.push_back(iter->second);
}
return result;
}
private:
map<int, Room*> rooms; // 所有客房
map<int, Customer*> customers; // 所有客户
};
// 主函数
int main() {
Hotel hotel;
int choice = 0;
while (choice != 5) {
cout << "1. 添加客房" << endl;
cout << "2. 删除客房" << endl;
cout << "3. 添加客户" << endl;
cout << "4. 删除客户" << endl;
cout << "5. 退出" << endl;
cout << "请选择操作:";
cin >> choice;
switch (choice) {
case 1: {
int id, price;
string type;
cout << "请输入客房编号:";
cin >> id;
cout << "请输入客房类型:";
cin >> type;
cout << "请输入客房价格:";
cin >> price;
Room* room = new Room(id, type, price);
hotel.addRoom(room);
break;
}
case 2: {
int id;
cout << "请输入客房编号:";
cin >> id;
hotel.removeRoom(id);
break;
}
case 3: {
int id;
string name, phone;
cout << "请输入客户编号:";
cin >> id;
cout << "请输入客户姓名:";
cin >> name;
cout << "请输入客户电话:";
cin >> phone;
Customer* customer = new Customer(id, name, phone);
hotel.addCustomer(customer);
break;
}
case 4: {
int id;
cout << "请输入客户编号:";
cin >> id;
hotel.removeCustomer(id);
break;
}
case 5: {
break;
}
default: {
cout << "无效操作!" << endl;
break;
}
}
}
return 0;
}
```
阅读全文
相关推荐





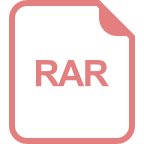
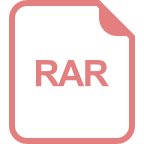

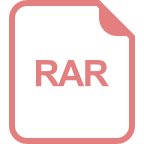
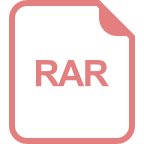
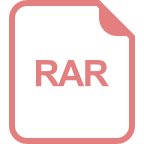





