请写一个链表排序的C语言程序
时间: 2023-08-30 17:04:06 浏览: 65
以下是链表排序的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void insert(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
void bubbleSort(struct Node* head_ref) {
struct Node* i;
struct Node* j;
int swapped;
for (i = head_ref; i != NULL; i = i->next) {
swapped = 0;
for (j = i->next; j != NULL; j = j->next) {
if (i->data > j->data) {
swap(&(i->data), &(j->data));
swapped = 1;
}
}
if (swapped == 0)
break;
}
}
int main() {
struct Node* head = NULL;
insert(&head, 5);
insert(&head, 20);
insert(&head, 4);
insert(&head, 3);
insert(&head, 30);
printf("Linked List before sorting: \n");
printList(head);
bubbleSort(head);
printf("Linked List after sorting: \n");
printList(head);
return 0;
}
```
该程序使用冒泡排序算法对链表进行排序。在 `insert` 函数中,我们将新节点插入到链表的头部。在 `bubbleSort` 函数中,我们使用两个 `for` 循环来比较和交换节点的数据。最后,我们在 `main` 函数中创建一个链表,并调用 `bubbleSort` 函数来对其进行排序。
相关推荐
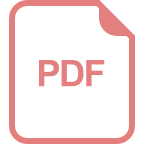














