请用Python编写一个程序:分别输入某一天的日期,包括年份,月份以及日。输出给定日期的第二天的日期。如,输入日期为2023 03 17,那么输出为2023 03 18。 >输入样例1:2024 02 28 >输出样例1:2024 02 29 >输入样例2:2024 02 29 >输出样例2:20240301
时间: 2024-02-01 11:16:07 浏览: 79
可以使用 Python 内置的 `datetime` 模块来完成日期的计算。以下是代码实现:
```python
from datetime import datetime, timedelta
# 读入日期
year, month, day = map(int, input().split())
# 构造 datetime 对象
date = datetime(year=year, month=month, day=day)
# 计算第二天的日期
next_date = date + timedelta(days=1)
# 输出结果
print(next_date.strftime('%Y %m %d'))
```
在这个程序中,我们首先使用 `input()` 函数读入日期,然后使用 `datetime` 对象来表示这个日期。接着,使用 `timedelta` 类型来计算第二天的日期,最后使用 `strftime()` 函数将日期转换成字符串输出。
需要注意的是,代码中的日期格式化字符串 `'%Y %m %d'` 中的 `%Y` 表示年份(四位数),`%m` 表示月份(两位数),`%d` 表示日(两位数)。
相关问题
输出某年某月的天数。 输入格式: 第一行输入年 第二行输入月 输出格式: 输出某年某月是几天 输入样例1: 2022 2 输出样例1: 2022年2月是28天 输入样例2: 2021 9 输出样例2: 2021年9月是30天python
在Python中,你可以编写一个简单的函数来计算给定年份和月份的天数。这个函数通常会根据格里高利历(公历)来确定月份的天数,考虑到闰年的规则。下面是一个简单的示例:
```python
def days_in_month(year, month):
# 定义一个字典存储每个月的天数
month_days = {1: 31, 2: 28, 3: 31, 4: 30, 5: 31, 6: 30, 7: 31, 8: 31, 9: 30, 10: 31, 11: 30, 12: 31}
# 判断是否是闰年,如果是,则2月有29天
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
month_days[2] = 29
return month_days.get(month)
# 测试函数
year = int(input().strip())
month = int(input().strip())
print(f"{year}年{month}月是{days_in_month(year, month)}天")
```
请注意,上述代码假设用户输入的是有效的一年和一月。如果需要更复杂的错误处理(比如负数、非整数输入),你可以在函数开始处添加相应的检查。
输入某年某月某日,判断这一天是这一年的第几天(用选择结构)
在用选择结构(如if-else、switch等)来编写这样的程序时,你可以遵循以下步骤:
1. 首先,确定给定日期是否在闰年。判断规则是:能被4整除但不能被100整除的年份是闰年;或者能被400整除也是闰年。
2. 然后,处理每个月的天数差异。一月有31天,二月在平年有28天,在闰年则有29天。
3. 接下来,从1月1日开始计数,逐个月累加天数。对于给定的日期之前的所有月份,直接加上该月的天数;如果到了二月,则需要考虑闰年的情况。
4. 到了2月,如果是闰年,就加上29天,如果不是闰年,加上28天。
5. 最后,检查给定日期是不是这个月的最后一天,如果是,那么当前的天数就是当年的总天数。
这是一个基本的伪代码框架:
```python
def is_leap_year(year):
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
return True
else:
return False
def day_of_year(year, month, day):
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if is_leap_year(year) and month == 2:
days_in_month[1] = 29
else:
days_in_month[1] = 28
total_days = sum(days_in_month[:month - 1]) + day
return total_days
# 示例
year = 2023
month = 3
day = 6
current_day = day_of_year(year, month, day)
```
阅读全文
相关推荐






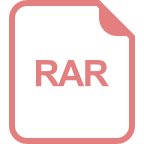
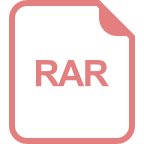
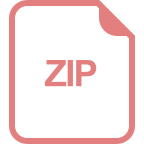
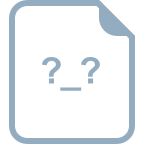
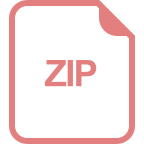
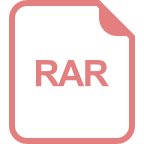
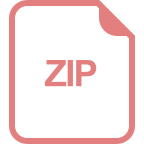