本题要求编写程序计算某年某月某日是该年中的第几天。 输入格式: 输入在一行中按照格式“yyyy/mm/dd”(即“年/月/日”)给出日期。注意:闰年的判别条件是该年年份能被4整除但不能被100整除、或者能被400整除。闰年的2月有29天。
时间: 2024-10-01 19:11:02 浏览: 91
为了编写一个程序来计算给定日期是一年中的第几天,你可以使用以下步骤:
1. 首先,将输入的字符串分割成单独的部分,例如年份、月份和日期。这通常通过 `str.split()` 函数完成。
2. 确认输入的日期是否有效,检查月份范围(1到12)和日期范围(对于非闰年,2月最多28天;闰年2月最多29天)。
3. 对于闰年的判断,可以添加一个函数来检查年份是否满足闰年的规则(能被4整除但不能被100整除,或能被400整除)。
4. 接下来,根据普通年份和闰年的差异来计算总天数。比如前两个月的天数(1月通常是31天,如果是2月,则是28天或29天),然后逐月累加,直到目标月份。
5. 计算给定月份之前的天数,并加上当前月份的天数(注意要考虑特殊月份如二月的闰年额外的一天)。
6. 最后返回总天数。
下面是一个简单的Python示例代码实现这个功能:
```python
def is_leap_year(year):
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
return True
else:
return False
def day_of_year(date_str):
date_parts = date_str.split('/')
year = int(date_parts[0])
month = int(date_parts[1])
day = int(date_parts[2])
# 检查输入的有效性
if not (1 <= month <= 12) or not (1 <= day <= (is_leap_year(year) and 29 or 28)):
print("Invalid date.")
return None
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if is_leap_year(year):
days_in_month[1] = 29
total_days = sum(days_in_month[:month - 1]) + day
return total_days
# 测试代码
date_input = input()
result = day_of_year(date_input)
if result is not None:
print(f"The given date {date_input} is on the {result}th day of the year.")
```
阅读全文
相关推荐
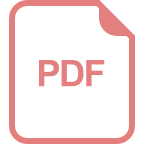
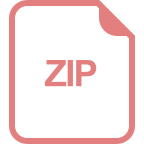
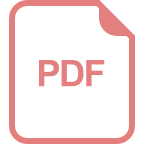








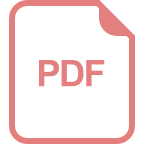
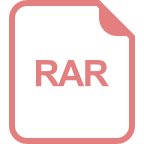
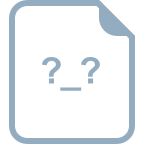
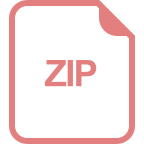
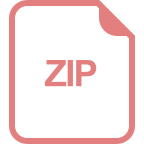