java 下拉框选项从数据库
时间: 2023-08-19 09:03:50 浏览: 388
实现下拉框选项从数据库获取的步骤如下:
1. 建立数据库连接:使用 JDBC API 建立数据库连接。
2. 查询数据库:使用 SQL 语句从数据库中获取下拉框选项的数据。
3. 将查询结果封装成列表:将查询结果封装成 Java 对象列表。
4. 将列表填充到下拉框中:使用 Java Swing 的 JComboBox 组件将列表填充到下拉框中。
以下是一个简单的示例代码:
```java
import javax.swing.*;
import java.sql.*;
import java.util.*;
public class ComboBoxFromDatabase extends JFrame {
private JComboBox<String> comboBox;
public ComboBoxFromDatabase() {
super("ComboBox from Database");
// Create database connection
try (Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "root", "password")) {
// Create SQL query
String sql = "SELECT name FROM mytable";
// Execute query and get result set
try (Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(sql)) {
// Create list to store results
List<String> options = new ArrayList<>();
// Loop through result set and add names to list
while (resultSet.next()) {
options.add(resultSet.getString("name"));
}
// Create combo box and add options
comboBox = new JComboBox<>(options.toArray(new String[0]));
getContentPane().add(comboBox);
}
} catch (SQLException e) {
e.printStackTrace();
}
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 200);
setVisible(true);
}
public static void main(String[] args) {
new ComboBoxFromDatabase();
}
}
```
在此示例中,我们使用了 MySQL 数据库,并且从名为 mytable 的表中获取了名字的列表。您需要将数据库连接字符串、用户名和密码更改为您自己的设置,并使用适合您的 SQL 语句从数据库中获取下拉框选项的数据。
阅读全文
相关推荐
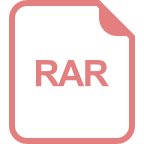




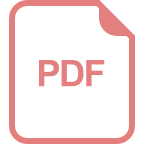
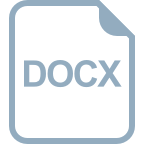
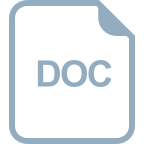
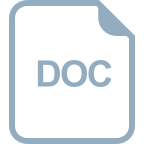






