使用消息调用msgget()、msgsnd()、msggrev()、msgctrl()编制长度为1K的消息的发送和接收程序
时间: 2024-02-07 22:01:59 浏览: 60
以下是使用消息队列进行消息发送和接收的示例代码:
发送程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_SIZE 1024
typedef struct msgbuf {
long mtype;
char mtext[MAX_SIZE];
} MsgBuf;
int main() {
key_t key;
int msgid;
MsgBuf buf;
// 创建消息队列
key = ftok(".", 'a');
if ((msgid = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget error");
exit(1);
}
// 设置消息类型和内容
buf.mtype = 1;
strcpy(buf.mtext, "Hello, world!");
// 发送消息
if (msgsnd(msgid, &buf, strlen(buf.mtext) + 1, 0) == -1) {
perror("msgsnd error");
exit(1);
}
printf("Message sent: %s\n", buf.mtext);
return 0;
}
```
接收程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAX_SIZE 1024
typedef struct msgbuf {
long mtype;
char mtext[MAX_SIZE];
} MsgBuf;
int main() {
key_t key;
int msgid;
MsgBuf buf;
// 获取消息队列
key = ftok(".", 'a');
if ((msgid = msgget(key, IPC_CREAT | 0666)) == -1) {
perror("msgget error");
exit(1);
}
// 接收消息
if (msgrcv(msgid, &buf, MAX_SIZE, 1, 0) == -1) {
perror("msgrcv error");
exit(1);
}
printf("Message received: %s\n", buf.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl error");
exit(1);
}
return 0;
}
```
这个示例程序中,我们创建了一个消息队列,发送端设置了消息的类型为1,接收端接收类型为1的消息,并输出消息内容。注意,在使用完消息队列后,我们需要使用msgctl函数将其删除。
阅读全文
相关推荐
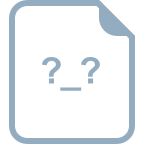
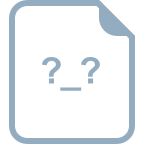




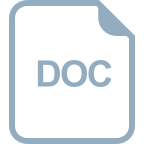
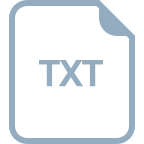
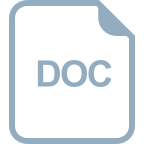
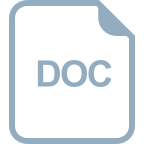
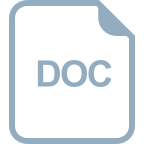
