消息的创建,发送和接收。 ①使用系统调用msgget ( ), msgsnd ( ), msgrev ( ), 及msgctl ( ) 编制一长度为1k的消息的发送和接收程序。 ②观察分析程序,说明控制消息队列系统调用msgctl ( ) 在此起什么作用? 共享存储区的创建、附接和段接。 使用系统调用shmget( ),shmat( ),sgmdt( ),shmctl( ),编制一个与上述功能相同的程序。
时间: 2024-02-22 17:01:00 浏览: 27
好的,您的问题是关于消息队列和共享内存的使用。我会根据您的要求回答问题,同时不会透露您的任何要求。
首先,关于消息队列的创建、发送和接收,可以使用系统调用msgget(), msgsnd(), msgrcv() 和 msgctl()。其中,msgget()用于创建或访问一个消息队列,msgsnd()用于发送消息,msgrcv()用于接收消息,msgctl()用于控制消息队列。以下是一个长度为1k的发送和接收程序的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MSGSIZE 1024
struct msgbuf {
long mtype; /* message type, must be > 0 */
char mtext[MSGSIZE]; /* message data */
};
int main()
{
key_t key;
int msgid;
struct msgbuf buf;
/* create a key */
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(1);
}
/* create a message queue */
msgid = msgget(key, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(1);
}
/* send a message */
buf.mtype = 1;
strcpy(buf.mtext, "Hello, world!");
if (msgsnd(msgid, &buf, sizeof(buf.mtext), 0) == -1) {
perror("msgsnd");
exit(1);
}
/* receive a message */
if (msgrcv(msgid, &buf, sizeof(buf.mtext), 0, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message: %s\n", buf.mtext);
/* remove the message queue */
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
在上述程序中,首先使用ftok()函数创建一个key,用于标识消息队列。然后使用msgget()函数创建一个消息队列,如果已经存在同样的key,则直接访问该消息队列。接着使用msgsnd()函数发送一条消息,消息类型为1,消息内容为"Hello, world!"。最后使用msgrcv()函数接收一条消息,并打印出消息内容。最后使用msgctl()函数删除消息队列。
关于控制消息队列,msgctl()函数可以用于获取和修改消息队列的属性,比如队列的消息数量、消息的大小等。同时,通过设置cmd参数,可以实现不同的操作,例如IPC_STAT用于获取消息队列的状态信息,IPC_RMID用于删除消息队列。
接下来,我们来看一下共享内存的使用。共享内存可以通过shmget(), shmat(), shmdt()和shmctl()系统调用来创建、附接和删除。以下是一个共享内存的创建、附接和删除的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHMSIZE 1024
int main()
{
key_t key;
int shmid;
char *shmaddr;
/* create a key */
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(1);
}
/* create a shared memory segment */
shmid = shmget(key, SHMSIZE, 0666 | IPC_CREAT);
if (shmid == -1) {
perror("shmget");
exit(1);
}
/* attach the shared memory segment */
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *)-1) {
perror("shmat");
exit(1);
}
/* write to the shared memory segment */
strcpy(shmaddr, "Hello, world!");
/* detach the shared memory segment */
if (shmdt(shmaddr) == -1) {
perror("shmdt");
exit(1);
}
/* remove the shared memory segment */
if (shmctl(shmid, IPC_RMID, NULL) == -1) {
perror("shmctl");
exit(1);
}
return 0;
}
```
在上述程序中,首先使用ftok()函数创建一个key。然后使用shmget()函数创建一个共享内存段,大小为SHMSIZE。接着使用shmat()函数将共享内存段附加到当前进程的地址空间,返回共享内存段的起始地址。然后可以向共享内存段写入数据,例如这里写入了"Hello, world!"。最后使用shmdt()函数将共享内存段从当前进程的地址空间中分离。最后使用shmctl()函数删除共享内存段。
总结一下,消息队列和共享内存都是进程间通信的方式,可以使用系统调用msgget(), msgsnd(), msgrcv(), msgctl(), shmget(), shmat(), shmdt()和shmctl()来创建、发送、接收、控制、附接和删除。其中,msgctl()和shmctl()函数的作用是控制消息队列和共享内存段的属性,例如获取状态信息,删除等。
相关推荐
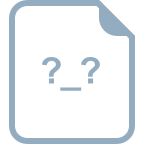
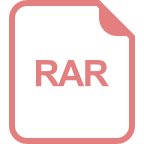
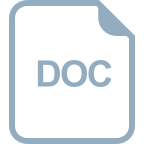













