①使用系统调用msgget(),msgsnd(),msgrcv(),及msgctl()等编制一个长度为1k的消息的发送和接收程序。 ②观察程序,说明控制消息队列系统调用
时间: 2023-11-13 10:04:47 浏览: 90
以下是使用系统调用msgget(),msgsnd(),msgrcv(),及msgctl()等编制一个长度为1k的消息的发送和接收程序的示例代码:
发送程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
key_t key;
int msgid;
struct msgbuf msg;
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid < 0) {
perror("msgget");
exit(1);
}
msg.mtype = 1;
strcpy(msg.mtext, "Hello, World!");
if (msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0) < 0) {
perror("msgsnd");
exit(1);
}
printf("Message sent: %s\n", msg.mtext);
return 0;
}
```
接收程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main() {
key_t key;
int msgid;
struct msgbuf msg;
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid < 0) {
perror("msgget");
exit(1);
}
if (msgrcv(msgid, &msg, MSG_SIZE, 1, 0) < 0) {
perror("msgrcv");
exit(1);
}
printf("Message received: %s\n", msg.mtext);
if (msgctl(msgid, IPC_RMID, NULL) < 0) {
perror("msgctl");
exit(1);
}
return 0;
}
```
在发送程序中,首先使用ftok()函数生成一个IPC键值,然后使用msgget()函数创建一个消息队列。接着定义一个msgbuf结构体,其中mtype成员表示消息类型,mtext成员表示消息内容。将要发送的消息内容复制到msgbuf结构体中的mtext成员中,然后使用msgsnd()函数将消息发送到消息队列中。最后打印出发送的消息内容。
在接收程序中,首先使用ftok()函数生成一个IPC键值,然后使用msgget()函数创建一个消息队列。接着定义一个msgbuf结构体,然后使用msgrcv()函数从消息队列中接收消息。msgtype参数指定要接收的消息类型,这里设置为1。如果成功接收到消息,则打印出消息内容。最后使用msgctl()函数删除消息队列。
控制消息队列的系统调用包括msgget()、msgsnd()、msgrcv()和msgctl()。其中,msgget()用于创建或访问一个消息队列,msgsnd()用于将消息发送到消息队列中,msgrcv()用于从消息队列中接收消息,msgctl()用于控制消息队列。这些系统调用都是通过IPC键值来访问消息队列的。在使用这些系统调用时,需要注意设置正确的消息类型和消息长度,以确保消息能够正确发送和接收。
相关推荐
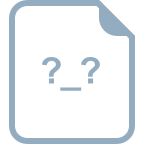
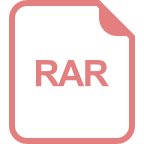














