①使用系统调用msgget ( ), msgsnd ( ), msgrev ( ), 及msgctl ( ) 编制一长度为1k的消息的发送和接收程序。 ②观察分析程序,说明控制消息队列系统调用msgctl ( ) 在此起什么作用?共享存储区的创建、附接和段接。 使用系统调用shmget( ),shmat( ),sgmdt( ),shmctl( ),编制一个与上述功能相同的程序。
时间: 2024-02-23 12:00:16 浏览: 136
好的,我会尽力回答你的问题。
1. 长度为1k的消息的发送和接收程序
下面是一个简单的实现,包括消息队列的创建、消息发送和接收、以及消息队列的删除。这里我们使用了系统调用msgget(), msgsnd(), msgrcv()和msgctl()。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#include <errno.h>
#define MSGSZ 1024 // 消息大小
// 消息结构体
typedef struct msgbuf {
long mtype;
char mtext[MSGSZ];
} message_buf;
int main() {
int msqid; // 消息队列ID
key_t key; // 消息队列键值
message_buf sbuf, rbuf; // 发送和接收消息缓冲区
size_t buflen; // 接收到的消息长度
int msgflg = IPC_CREAT | 0666; // IPC_CREAT表示如果消息队列不存在则创建它,0666表示权限
long int msgtype = 1; // 消息类型
// 生成key
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 创建消息队列
if ((msqid = msgget(key, msgflg)) < 0) {
perror("msgget");
exit(1);
}
printf("Message Queue created with ID: %d\n", msqid);
// 发送消息
sbuf.mtype = msgtype;
printf("Enter a message to send: ");
fgets(sbuf.mtext, MSGSZ, stdin);
if (msgsnd(msqid, &sbuf, strlen(sbuf.mtext)+1, IPC_NOWAIT) < 0) {
printf("%d, %d\n", msqid, sbuf.mtype);
perror("msgsnd");
exit(1);
}
printf("Message sent: %s\n", sbuf.mtext);
// 接收消息
if ((buflen = msgrcv(msqid, &rbuf, MSGSZ, msgtype, 0)) < 0) {
perror("msgrcv");
exit(1);
}
printf("Message received: %s\n", rbuf.mtext);
// 删除消息队列
if (msgctl(msqid, IPC_RMID, NULL) < 0) {
perror("msgctl");
exit(1);
}
printf("Message Queue deleted\n");
return 0;
}
```
2. msgctl()的作用
msgctl()函数用于对消息队列进行控制操作,包括获取/设置消息队列属性、删除消息队列等。在上述程序中,我们使用msgctl()函数删除了消息队列。
3. 共享存储区的创建、附接和段接程序
下面是一个简单的实现,包括共享存储区的创建、附接、段接和删除。这里我们使用了系统调用shmget(), shmat(), shmdt()和shmctl()。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/shm.h>
#include <sys/ipc.h>
#include <errno.h>
#define SHMSZ 1024 // 共享内存大小
int main() {
int shmid; // 共享内存ID
key_t key; // 共享内存键值
char *shm, *s; // 共享内存指针和写入数据的指针
int shmflg = IPC_CREAT | 0666; // IPC_CREAT表示如果共享内存不存在则创建它,0666表示权限
// 生成key
if ((key = ftok(".", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 创建共享内存
if ((shmid = shmget(key, SHMSZ, shmflg)) < 0) {
perror("shmget");
exit(1);
}
printf("Shared Memory created with ID: %d\n", shmid);
// 附接共享内存
if ((shm = shmat(shmid, NULL, 0)) == (char *) -1) {
perror("shmat");
exit(1);
}
printf("Memory attached at %p\n", shm);
// 写入数据
s = shm;
printf("Enter a message to write: ");
fgets(s, SHMSZ, stdin);
// 段接共享内存
if (shmdt(shm) < 0) {
perror("shmdt");
exit(1);
}
printf("Memory detached\n");
// 删除共享内存
if (shmctl(shmid, IPC_RMID, NULL) < 0) {
perror("shmctl");
exit(1);
}
printf("Shared Memory deleted\n");
return 0;
}
```
希望这些代码能帮助你解决问题。如果还有其他问题或疑问,请随时提出。
阅读全文
相关推荐
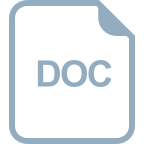
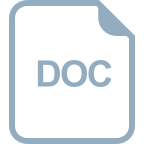
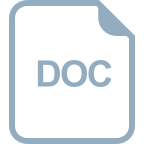
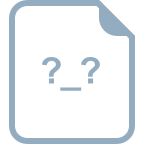








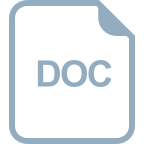
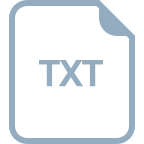
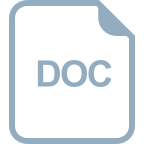
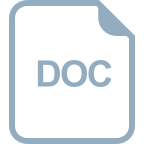
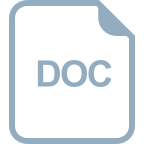
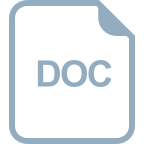