设计一个程序,要求用函数msgget创建消息队列,从键盘输入的字符串添加到消息队列,然后应用函数msgrcv读取队列中的消息并在计算机屏幕上输出。程序先调用msgget函数创建、打开消息队列,接着调用msgsnd函数,把输入的字符串添加到消息队列中,然后调用msgrcv函数,读取消息队列中的消息并打印输出,最后调用msgctl函数,删除系统内核中的消息队列
时间: 2024-03-13 12:45:12 浏览: 84
好的,以下是可以实现上述功能的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define MAXSIZE 100
struct msgbuf {
long mtype;
char mtext[MAXSIZE];
};
int main()
{
int msgid;
key_t key;
struct msgbuf message;
// 生成key值
if ((key = ftok("msgqueue.txt", 'a')) == -1) {
perror("ftok");
exit(1);
}
// 创建消息队列
if ((msgid = msgget(key, 0644 | IPC_CREAT)) == -1) {
perror("msgget");
exit(1);
}
printf("Enter message: ");
fgets(message.mtext, MAXSIZE, stdin);
message.mtype = 1;
// 将消息添加到队列中
if (msgsnd(msgid, &message, MAXSIZE, 0) == -1) {
perror("msgsnd");
exit(1);
}
// 读取并输出队列中的消息
if (msgrcv(msgid, &message, MAXSIZE, 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
printf("Received message: %s", message.mtext);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(1);
}
return 0;
}
```
程序通过调用 `ftok` 函数生成一个唯一的 key 值,然后使用 `msgget` 函数创建或打开一个消息队列。接着,程序从键盘输入一个字符串,并将其添加到消息队列中,用 `msgrcv` 函数读取队列中的消息,并将其输出到计算机屏幕上。最后,程序使用 `msgctl` 函数来删除系统内核中的消息队列。
阅读全文
相关推荐
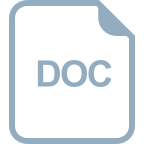
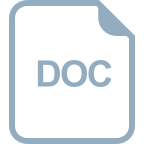
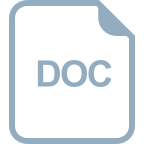

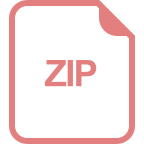
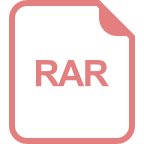
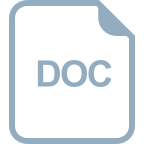
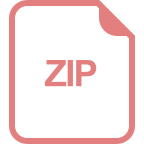
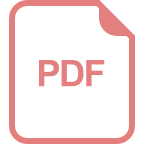
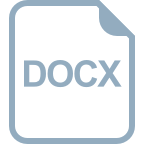
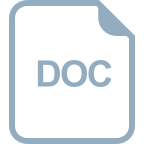
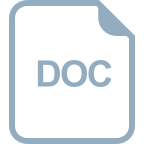
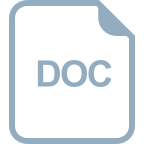
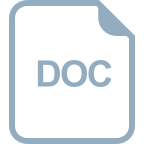
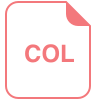
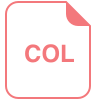
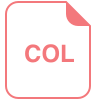
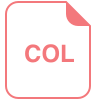
