用c++编写一个程序,使用系统调用msgget(), msgsnd(), msgrcv(), msgctl()实现消息的发送和接收,一个子进程发送10条消息,一个子进程接收10条消息。(MSGKEY取值75,消息的长度取1024)
时间: 2023-06-08 22:04:50 浏览: 209
好的,我能够理解您的要求。以下是使用系统调用msgget(), msgsnd(), msgrcv(), msgctl()实现消息的发送和接收的C程序,其中子进程1发送10条消息,子进程2接收10条消息, MSGKEY取值75,消息的长度取1024。
```c
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<errno.h>
#include<sys/types.h>
#include<sys/ipc.h>
#include<sys/msg.h>
#define MSGKEY 75 // 定义消息队列键值
#define MSGSIZE 1024 // 定义消息的长度
// 定义消息结构体
struct msgbuf {
long mtype; // 消息类型
char mtext[MSGSIZE]; // 消息文本
};
int main() {
int msgid, status;
pid_t pid;
struct msgbuf msg; // 定义消息结构体
// 创建消息队列
msgid = msgget(MSGKEY, 0666 | IPC_CREAT);
if (msgid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 创建子进程1
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
else if (pid == 0) { // 子进程1发送10条消息
int i;
for (i = 1; i <= 10; i++) {
sprintf(msg.mtext, "Message %d from process 1", i); // 设置消息文本
msg.mtype = 1; // 设置消息类型
printf("Sending message: %s\n", msg.mtext); // 打印消息文本
if (msgsnd(msgid, &msg, strlen(msg.mtext) + 1, 0) == -1) { // 发送消息
perror("msgsnd");
exit(EXIT_FAILURE);
}
}
exit(EXIT_SUCCESS); // 退出子进程1
}
else { // 父进程继续执行
// 创建子进程2
pid = fork();
if (pid == -1) {
perror("fork");
exit(EXIT_FAILURE);
}
else if (pid == 0) { // 子进程2接收10条消息
int i;
for (i = 1; i <= 10; i++) {
if (msgrcv(msgid, &msg, MSGSIZE, 1, 0) == -1) { // 接收消息
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("Received message: %s\n", msg.mtext); // 打印消息文本
}
exit(EXIT_SUCCESS); // 退出子进程2
}
else { // 父进程继续执行
// 等待子进程1和2退出
waitpid(pid, &status, 0);
waitpid(pid, &status, 0);
// 删除消息队列
if (msgctl(msgid, IPC_RMID, NULL) == -1) {
perror("msgctl");
exit(EXIT_FAILURE);
}
}
}
return 0;
}
```
请注意,这个程序只是一个简单的示例,具体实现方式可能会根据您的系统环境和需求略有不同。如果您遇到任何问题,请随时与我联系。
相关推荐
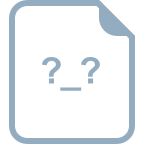
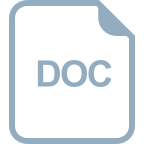
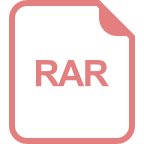
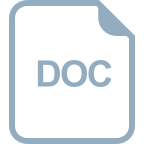
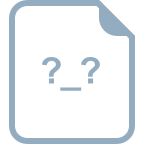











