编写程序,使其用消息队列来实现client进程(client_3207623130.c)和server进程(server_3207623130.c)之间的通信。①server进程先建立一个关键字为75的消息队列,然后等待接收类型为1的消息(消息内容为:client进程的进程标识符和“client 学号”);在收到请求消息后,server进程便显示字符串“serving for client”和接收到client进程的进程标识符,表示正在为client进程服务。②然后server进程再向client进程发送应答消息,该消息的类型是client进程的进程标识符(消息内容为:“sever进程的标识符”和“server 学号”)。client进程等待server进程发来的消息(消息类型为client自己的进程标识符),然后显示字符串“receiver reply from”和接收到server进程的标识符。要求:利用消息缓冲队列进行进程间的通信,运用系统调用msgget()、msgsnd()、msgrcv()、msgctl()的功能和实现过程,请给出client_3207623130.c、server_3207623130.c代码,以及我在linux中输入的命令
时间: 2023-06-08 07:04:21 浏览: 170
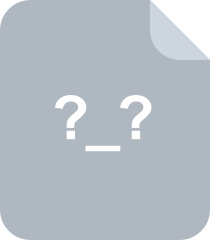
消息队列程序,进程间通信。
server_3207623130.c:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define CLIENT_MSG_TYPE 1
int main() {
int server_msgqid, client_msgqid;
key_t server_key = 75;
struct msgbuf {
long mtype;
char data[100];
} server_msgbuf, client_msgbuf;
//创建关键字为75的消息队列
server_msgqid = msgget(server_key, IPC_CREAT | 0666);
if (server_msgqid < 0) {
perror("msgget error: ");
exit(1);
}
printf("Server message queue created.\n");
while (1) {
//等待接收类型为1的消息
msgrcv(server_msgqid, &client_msgbuf, sizeof(client_msgbuf.data), CLIENT_MSG_TYPE, 0);
printf("Serving for client [PID=%ld]: %s\n", client_msgbuf.mtype, client_msgbuf.data);
//创建应答消息并发送给client
server_msgbuf.mtype = client_msgbuf.mtype;
sprintf(server_msgbuf.data, "Server [PID=%d] is serving for client", getpid());
client_msgqid = msgget(server_msgbuf.mtype, 0666 | IPC_CREAT); //创建client的消息队列
msgsnd(client_msgqid, &server_msgbuf, sizeof(server_msgbuf.data), 0);
}
return 0;
}
client_3207623130.c:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#define SERVER_MSG_TYPE 2
int main() {
int client_msgqid, server_msgqid;
key_t client_key;
struct msgbuf {
long mtype;
char data[100];
} client_msgbuf, server_msgbuf;
client_key = getpid();
//创建client的消息队列
client_msgqid = msgget(client_key, IPC_CREAT | 0666);
if (client_msgqid < 0) {
perror("msgget error: ");
exit(1);
}
printf("Client message queue created.\n");
while (1) {
//向server发送请求消息
server_msgqid = msgget(75, 0666 | IPC_CREAT); //获取server的消息队列
client_msgbuf.mtype = 1;
sprintf(client_msgbuf.data, "%ld--client_3207623130", getpid());
msgsnd(server_msgqid, &client_msgbuf, sizeof(client_msgbuf.data), 0);
//等待server回应
msgrcv(client_msgqid, &server_msgbuf, sizeof(server_msgbuf.data), getpid(), 0);
printf("Receiver reply from server [PID=%ld]: %s\n", server_msgbuf.mtype, server_msgbuf.data);
}
return 0;
}
Linux命令:
gcc -o server server_3207623130.c
gcc -o client client_3207623130.c
./server &
./client &
注意:在Linux中运行时需要终止进程,可以使用“kill -9 进程号”的命令。
阅读全文
相关推荐


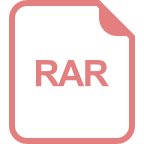

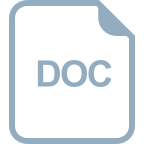
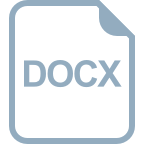
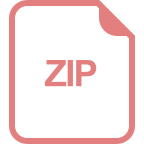
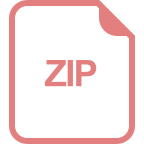
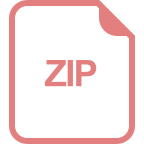
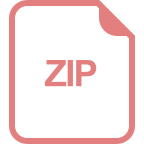
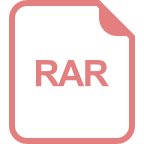
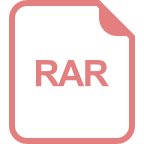
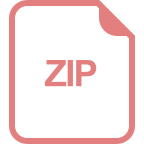
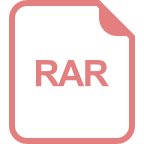
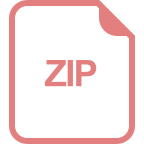
