用c语言编写一段程序,使其用消息缓冲队列来实现 client 进程和 server 进程之间的通信。server 进程先建立一个关键字为 SVKEY(如 75)的消息队列,然后等待接收类型为 REQ(如1)的消息;在收到请求消息后,它便显示字符串“serving for client”和接收到的 client进程的进程标识数,表示正在为 client 进程服务:然后再向 client 程发送一应答消息,该消息的类型是 client进程的进程标识数,而正文则是 server 进程自己的标识数。client程向消息队列发送类型为REQ的消息(消息的正文为自己的进程标识数)以取得server进程的服务,并等待 server程发来的应答,然后显示字符串“receivereply from%和接收到的server 进程的标识数。
时间: 2024-02-01 14:13:06 浏览: 24
以下是用消息缓冲队列实现 client 进程和 server 进程之间通信的 C 语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <unistd.h>
#define SVKEY 75 // 消息队列关键字
#define REQ 1 // 请求消息类型
// 定义消息结构体
typedef struct message {
long mtype;
int pid;
} Message;
int main() {
int msqid;
pid_t pid = getpid();
Message req_msg, reply_msg;
// 创建或获取消息队列
msqid = msgget(SVKEY, IPC_CREAT | 0666);
if (msqid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 发送请求消息
req_msg.mtype = REQ;
req_msg.pid = pid;
if (msgsnd(msqid, &req_msg, sizeof(int), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
printf("Sent request message from client %d.\n", pid);
// 接收应答消息
if (msgrcv(msqid, &reply_msg, sizeof(int), pid, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("Received reply message from server %d.\n", reply_msg.mtype);
return 0;
}
```
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <unistd.h>
#define SVKEY 75 // 消息队列关键字
#define REQ 1 // 请求消息类型
// 定义消息结构体
typedef struct message {
long mtype;
int pid;
} Message;
int main() {
int msqid;
pid_t pid = getpid();
Message req_msg, reply_msg;
// 创建或获取消息队列
msqid = msgget(SVKEY, IPC_CREAT | 0666);
if (msqid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
// 接收请求消息
if (msgrcv(msqid, &req_msg, sizeof(int), REQ, 0) == -1) {
perror("msgrcv");
exit(EXIT_FAILURE);
}
printf("Received request message from client %d.\n", req_msg.pid);
// 发送应答消息
reply_msg.mtype = req_msg.pid;
reply_msg.pid = pid;
if (msgsnd(msqid, &reply_msg, sizeof(int), 0) == -1) {
perror("msgsnd");
exit(EXIT_FAILURE);
}
printf("Sent reply message from server %d.\n", pid);
return 0;
}
```
在这个例子中,client 进程向消息队列发送类型为 REQ 的消息,消息的正文为自己的进程标识数。server 进程先建立关键字为 SVKEY 的消息队列,然后等待接收类型为 REQ 的消息。在收到请求消息后,它会显示字符串“serving for client”和接收到的 client 进程的进程标识数,表示正在为 client 进程服务。然后再向 client 进程发送一应答消息,该消息的类型是 client 进程的进程标识数,而正文则是 server 进程自己的标识数。client 进程等待 server 进程发来的应答,然后显示字符串“received reply from”和接收到的 server 进程的标识数。
注意:在 Linux 中,进程间通信的方式有很多种,消息缓冲队列只是其中一种。此外,这个例子只是演示了如何使用消息缓冲队列实现进程间通信,具体应用时需要根据实际情况进行修改和完善。
相关推荐
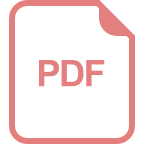














