写一个这样的代码,vue上传成功文件之后,通过前端发送请求,到后台,如何通过获取每一行表格的id来下载文件
时间: 2023-12-06 16:44:14 浏览: 106
假设你已经实现了前端文件上传并成功保存到后台服务器,接下来是前端获取后台返回的文件下载地址并实现点击下载。
前端代码:
```html
<template>
<div>
<table>
<thead>
<tr>
<th>ID</th>
<th>文件名</th>
<th>下载</th>
</tr>
</thead>
<tbody>
<tr v-for="item in tableData" :key="item.id">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>
<a :href="item.downloadUrl" download>下载</a>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [
{ id: 1, name: 'file1.txt', downloadUrl: '' },
{ id: 2, name: 'file2.txt', downloadUrl: '' },
{ id: 3, name: 'file3.txt', downloadUrl: '' },
],
};
},
methods: {
handleUploadSuccess(response, file) {
// 上传成功后处理逻辑
const item = this.tableData.find((item) => item.id === response.id);
item.downloadUrl = response.downloadUrl;
},
},
};
</script>
```
在上传成功的回调函数中,我们可以通过查找表格数据中对应的行,将下载链接赋值到对应的行数据中。这里只是模拟了一个本地数据,实际场景中需要根据后台返回的数据格式进行修改。
后端代码(Java SpringBoot):
```java
@RestController
public class FileController {
// 文件上传接口
@PostMapping("/upload")
public ResponseEntity<?> uploadFile(@RequestParam("file") MultipartFile file, @RequestParam("id") Long id) {
// 处理上传逻辑
// ...
// 返回下载链接
String downloadUrl = "http://localhost:8080/download?id=" + id;
Map<String, String> response = new HashMap<>();
response.put("downloadUrl", downloadUrl);
return ResponseEntity.ok(response);
}
// 文件下载接口
@GetMapping("/download")
public void downloadFile(@RequestParam("id") Long id, HttpServletResponse response) throws IOException {
// 根据id获取文件路径
String filePath = "path/to/file" + id + ".txt";
File file = new File(filePath);
// 设置响应头
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=" + file.getName());
response.setHeader("Content-Length", String.valueOf(file.length()));
// 拷贝文件流到响应输出流
InputStream inputStream = new FileInputStream(file);
OutputStream outputStream = response.getOutputStream();
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.flush();
inputStream.close();
outputStream.close();
}
}
```
在上传接口中,我们需要将上传的文件保存到服务器上,并返回下载链接。这里我们将下载链接设置为 `http://localhost:8080/download?id={id}` 的形式,其中 `{id}` 是上传时传入的文件 id。
在下载接口中,我们需要根据传入的 id 获取文件路径,设置响应头,然后将文件流拷贝到响应输出流中,以实现下载功能。
阅读全文
相关推荐
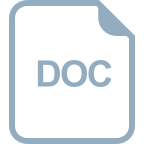
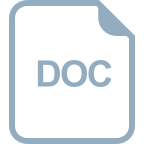
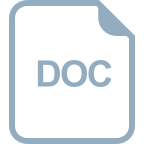

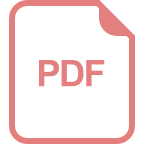
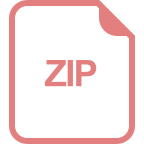
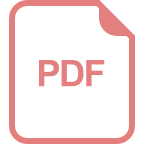
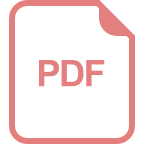
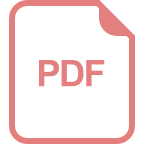
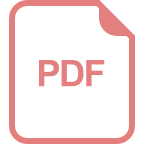
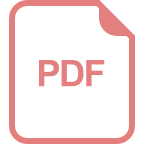
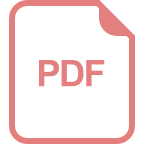
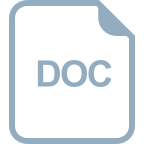
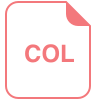
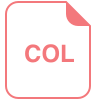
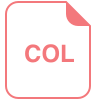
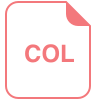
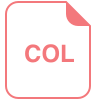
