C语音多项式的创建代码
时间: 2024-10-24 19:11:52 浏览: 7
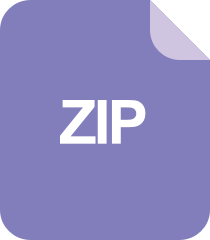
牛顿插值多项式C代码.zip
在C语言中,创建多项式通常涉及到结构体来存储系数和次数,以及函数来添加、减去、乘以等操作。这里是一个简单的例子,展示如何创建一个多项式结构体:
```c
#include <stdio.h>
// 定义多项式项的数据结构
typedef struct {
int coefficient; // 系数
int exponent; // 次数
} PolynomialTerm;
// 创建一个新的多项式项
PolynomialTerm create_term(int coefficient, int exponent) {
PolynomialTerm term;
term.coefficient = coefficient;
term.exponent = exponent;
return term;
}
// 多项式表示为数组,其中最后一个元素表示常数项(exponent为0)
typedef struct {
PolynomialTerm terms[10]; // 假设最多有10项
int size; // 当前项数
} Polynomial;
// 初始化多项式
void init_polynomial(Polynomial* poly) {
poly->size = 0;
}
// 添加新项到多项式
void add_term(Polynomial* poly, int coefficient, int exponent) {
if (poly->size < sizeof(poly->terms)) {
poly->terms[poly->size] = create_term(coefficient, exponent);
poly->size++;
} else {
printf("多项式已满,无法添加更多项\n");
}
}
// 打印多项式
void print_polynomial(const Polynomial* poly) {
for (int i = 0; i <= poly->size - 1; i++) {
if (poly->terms[i].exponent == 0)
printf("%d", poly->terms[i].coefficient); // 如果是常数项
else
printf("%dx^%d", poly->terms[i].coefficient, poly->terms[i].exponent);
if (i != poly->size - 1) printf("+"); // 非最后一项加"+"连接
}
}
int main() {
Polynomial poly;
init_polynomial(&poly);
// 示例:添加 x^2 + 3x + 4
add_term(&poly, 1, 2);
add_term(&poly, 3, 1);
add_term(&poly, 4, 0);
print_polynomial(&poly);
return 0;
}
```
阅读全文
相关推荐
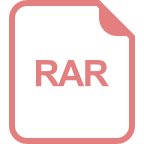
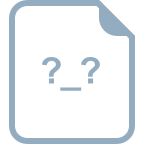
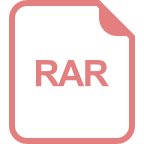
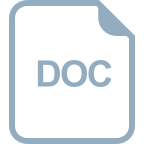
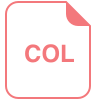
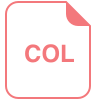
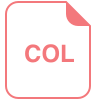

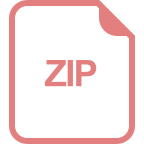