java获取文件上传进度
时间: 2023-01-11 09:55:36 浏览: 102
To get the progress of a file upload in Java, you can use a `ProgressListener` that updates the progress of the upload as it is happening. Here's an example of how you might implement a `ProgressListener` using the Apache Commons FileUpload library:
```java
import org.apache.commons.fileupload.ProgressListener;
public class UploadProgressListener implements ProgressListener {
private long megaBytes = -1;
@Override
public void update(long pBytesRead, long pContentLength, int pItems) {
long mBytes = pBytesRead / 1000000;
if (megaBytes == mBytes) {
return;
}
megaBytes = mBytes;
System.out.println("We are currently reading item " + pItems);
if (pContentLength == -1) {
System.out.println("So far, " + pBytesRead + " bytes have been read.");
} else {
System.out.println("So far, " + pBytesRead + " of " + pContentLength
+ " bytes have been read.");
}
}
}
```
To use the `ProgressListener`, you will need to create an instance of it and pass it to the `FileUpload` object when you are parsing the request. Here's an example of how you might do this:
```java
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.FileUpload;
import org.apache.commons.fileupload.FileUploadBase;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
import javax.servlet.http.HttpServletRequest;
import java.util.List;
public class FileUploader {
public void handleFileUpload(HttpServletRequest request) throws Exception {
// Create a factory for disk-based file items
DiskFileItemFactory factory = new DiskFileItemFactory();
// Create a new file upload handler
ServletFileUpload upload = new ServletFileUpload(factory);
// Set progress listener
upload.setProgressListener(new UploadProgressListener());
// Parse the request
List<FileItem> items = upload.parseRequest(request);
// Process the uploaded items
for (FileItem item : items) {
if (!item.isFormField()) {
// It's a file upload
String fileName = item.getName();
// Process the uploaded file
} else {
// It's a regular form field
String fieldName = item.getFieldName();
String fieldValue = item.getString();
// Process the form field
}
}
}
}
```
I hope this helps! Let me know if you have any questions.
阅读全文
相关推荐
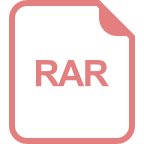



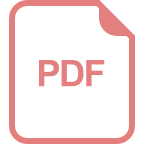
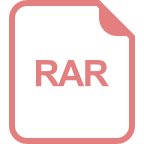
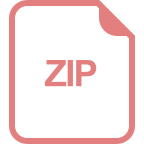
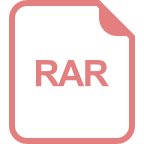
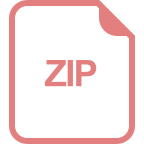
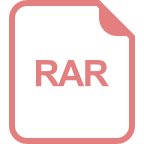
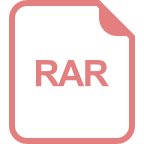
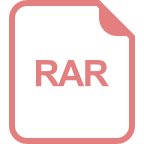
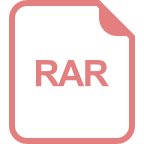
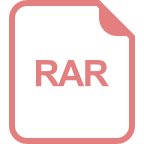
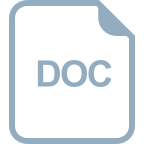
