写一个可以在微博抓取评论的代码
时间: 2024-03-31 13:31:58 浏览: 40
抓取微博评论需要使用微博开放平台的API,需要先进行注册和创建应用,获取相应的App Key和App Secret。以下是一个Python示例代码,可以获取指定微博的评论:
```python
import requests
import json
# 设置参数
access_token = '你的access_token'
weibo_id = '微博ID'
# 构造API请求
url = 'https://api.weibo.com/2/comments/show.json?access_token={}&id={}'.format(access_token, weibo_id)
# 发送API请求
response = requests.get(url)
# 解析API响应
if response.status_code == 200:
comments = json.loads(response.text)['comments']
for comment in comments:
print(comment['text'])
else:
print('API请求失败')
```
其中,`access_token`是通过OAuth2.0授权获取的访问令牌,`weibo_id`是需要抓取评论的微博的ID。该示例代码通过调用微博API获取指定微博的评论,并将评论内容打印到控制台中。
相关问题
python爬虫抓取微博评论数据的编程代码
在Python中抓取微博评论数据通常需要使用Selenium、Scrapy等库来模拟浏览器行为,因为微博这类网站可能会有反爬机制。以下是一个简单的使用Selenium的示例,展示如何获取某条微博的评论:
```python
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
# 创建Chrome驱动实例
driver = webdriver.Chrome()
def get_weibo_comments(url):
driver.get(url) # 访问微博页面
time.sleep(5) # 等待页面加载完成
# 查找评论区域的HTML元素,这通常是通过评论的父级节点id来定位
comment_divs = driver.find_elements(By.CSS_SELECTOR, '#comments > div')
for comment in comment_divs:
# 解析每个评论的内容
content = comment.find_element(By.CSS_SELECTOR, '.comment-content').text
# 获取用户信息
user_info = comment.find_element(By.CSS_SELECTOR, '.comment-user-name').text
print(f"评论内容: {content}")
print(f"用户信息: {user_info}\n")
# 使用实际的微博评论链接替换此处
weibo_url = "https://weibo.com/comment/show?id=XXX&rl=0"
get_weibo_comments(weibo_url)
# 关闭浏览器
driver.quit()
用python完成抓取微博文本的代码
以下是一个基本的Python代码示例,用于从微博抓取文本:
1. 首先,我们需要安装 `requests` 和 `beautifulsoup4` 库:
```
pip install requests
pip install beautifulsoup4
```
2. 然后,我们需要导入这些库:
```python
import requests
from bs4 import BeautifulSoup
```
3. 接下来,我们可以使用 `requests` 库来获取微博页面的 HTML 内容:
```python
url = 'https://weibo.com/u/1234567890'
response = requests.get(url)
html = response.content
```
4. 然后,我们可以使用 `beautifulsoup4` 库来解析 HTML 内容:
```python
soup = BeautifulSoup(html, 'html.parser')
```
5. 接下来,我们可以使用 `soup` 对象来查找微博文本:
```python
texts = []
for tag in soup.find_all('div', {'class': 'WB_text'}):
texts.append(tag.text)
```
6. 最后,我们可以打印或保存文本:
```python
for text in texts:
print(text)
```
完整代码示例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://weibo.com/u/1234567890'
response = requests.get(url)
html = response.content
soup = BeautifulSoup(html, 'html.parser')
texts = []
for tag in soup.find_all('div', {'class': 'WB_text'}):
texts.append(tag.text)
for text in texts:
print(text)
```
阅读全文
相关推荐
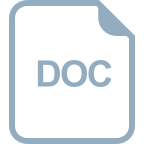
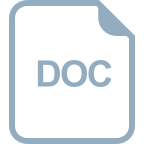
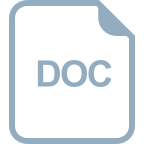
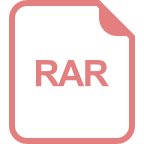
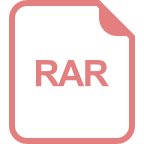
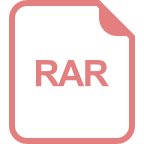
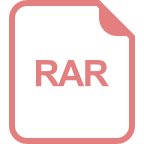
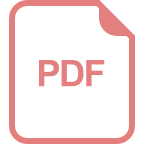
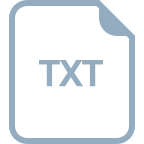
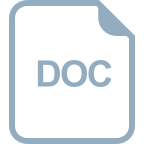





