用PYTHON 写一个类,可以进行MONGO数据库的增删改查,包含分页查询
时间: 2023-08-31 11:24:25 浏览: 64
### 回答1:
好的,这是一个例子,你可以根据自己的需要进行修改。
首先,你需要安装 pymongo 库,这是 Python 中操作 MongoDB 数据库的工具。你可以使用 pip 命令来安装:
```
pip install pymongo
```
然后,你可以使用以下代码创建一个 MongoDB 操作类:
```python
import pymongo
class MongoDB:
def __init__(self, host, port, db_name, collection_name):
self.client = pymongo.MongoClient(host, port)
self.db = self.client[db_name]
self.collection = self.db[collection_name]
def insert_one(self, document):
self.collection.insert_one(document)
def insert_many(self, documents):
self.collection.insert_many(documents)
def update_one(self, filter, update):
self.collection.update_one(filter, update)
def update_many(self, filter, update):
self.collection.update_many(filter, update)
def delete_one(self, filter):
self.collection.delete_one(filter)
def delete_many(self, filter):
self.collection.delete_many(filter)
def find_one(self, filter):
return self.collection.find_one(filter)
def find(self, filter):
return self.collection.find(filter)
```
这个类的用法如下:
```python
# 创建 MongoDB 对象
mongo = MongoDB('localhost', 27017, 'mydb', 'mycollection')
# 插入单个文档
document = {'name': 'John', 'age': 30}
mongo.insert_one(document)
# 插入多个文档
documents = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 35}]
mongo.insert_many(documents)
# 更新单个文档
filter = {'name': 'John'}
update = {'$set': {'age': 40}}
mongo.update_one(filter, update)
# 更新多个文档
filter = {'age': {'$lt': 30}}
update = {'$set': {'age': 30}}
mongo.update_many(filter, update)
# 删除单个文档
filter = {'name': 'John'
### 回答2:
在Python中,可以使用pymongo库来进行MongoDB数据库的增删改查操作。以下是一个使用Python编写的类,可以实现对MongoDB数据库的增删改查,并包含分页查询的功能。
```python
from pymongo import MongoClient
class MongoDB:
def __init__(self, host='localhost', port=27017, db_name='test'):
self.client = MongoClient(host, port)
self.db = self.client[db_name]
def insert(self, collection_name, data):
collection = self.db[collection_name]
inserted = collection.insert_one(data)
return inserted.inserted_id
def update(self, collection_name, query, data):
collection = self.db[collection_name]
updated = collection.update_one(query, {'$set': data})
return updated.modified_count
def delete(self, collection_name, query):
collection = self.db[collection_name]
deleted = collection.delete_one(query)
return deleted.deleted_count
def find(self, collection_name, query={}, fields=None):
collection = self.db[collection_name]
if fields:
result = collection.find(query, fields)
else:
result = collection.find(query)
return list(result)
def paginate(self, collection_name, page=1, limit=10, query={}, fields=None):
collection = self.db[collection_name]
skip = (page - 1) * limit
if fields:
result = collection.find(query, fields).skip(skip).limit(limit)
else:
result = collection.find(query).skip(skip).limit(limit)
return list(result)
```
上述代码中,我们使用了pymongo库的MongoClient类来建立与MongoDB的连接,并使用db[]语法来获取指定的数据库。其中,insert()方法用于插入数据,update()方法用于更新数据,delete()方法用于删除数据,find()方法用于查询数据,paginate()方法用于分页查询数据。
使用时,可以先实例化一个MongoDB对象,然后通过该对象调用相应的方法来实现对MongoDB数据库的增删改查操作。例如:
```python
mongo = MongoDB(host='localhost', port=27017, db_name='mydb')
# 插入数据
data = {'name': 'Alice', 'age': 25}
inserted_id = mongo.insert('users', data)
print(f'Inserted id: {inserted_id}')
# 更新数据
query = {'name': 'Alice'}
data = {'age': 26}
modified_count = mongo.update('users', query, data)
print(f'Modified count: {modified_count}')
# 删除数据
query = {'name': 'Alice'}
deleted_count = mongo.delete('users', query)
print(f'Deleted count: {deleted_count}')
# 查询数据
query = {'age': { '$lt': 30 }}
result = mongo.find('users', query)
for user in result:
print(user)
# 分页查询数据
page = 2
limit = 5
result = mongo.paginate('users', page, limit)
for user in result:
print(user)
```
需要注意的是,上述代码只是一个简单的示例,实际使用中可能需要根据具体业务需求进行进一步封装和调整。
### 回答3:
使用Python编写一个可以进行MongoDB数据库的增删改查的类,包括分页查询。首先,我们需要导入pymongo库来连接MongoDB数据库。
```python
from pymongo import MongoClient
class MongoDB:
def __init__(self, host='localhost', port=27017, db_name='test', collection_name='data'):
# 连接MongoDB数据库
self.client = MongoClient(host, port)
self.db = self.client[db_name]
self.collection = self.db[collection_name]
def insert(self, data):
# 在集合中插入一条数据
result = self.collection.insert_one(data)
return result.inserted_id
def delete(self, query):
# 根据条件删除数据
result = self.collection.delete_many(query)
return result.deleted_count
def update(self, query, new_data):
# 根据条件更新数据
result = self.collection.update_many(query, {'$set': new_data})
return result.modified_count
def find(self, query, page_size=10, page_number=1):
# 根据条件查询数据,并进行分页
total_count = self.collection.count_documents(query)
total_page = int(total_count / page_size) + 1
if page_number > total_page:
page_number = total_page
if page_number < 1:
page_number = 1
skip_count = (page_number - 1) * page_size
result = self.collection.find(query).skip(skip_count).limit(page_size)
return result
db = MongoDB()
data = {'name': 'John', 'age': 30}
id = db.insert(data)
print('Inserted document ID:', id)
query = {'name': 'John'}
count = db.delete(query)
print('Deleted documents count:', count)
query = {'name': 'John'}
new_data = {'age': 35}
count = db.update(query, new_data)
print('Modified documents count:', count)
query = {'age': {'$gte': 30}}
result = db.find(query, page_size=5, page_number=1)
for doc in result:
print(doc)
```
这个类示例化时默认连接本地的MongoDB数据库,可以指定其他的主机地址、端口号、数据库名称和集合名称。insert方法用于插入一条数据,返回插入的文档ID。delete方法根据条件删除数据,返回删除的文档数。update方法根据条件更新数据,返回更新的文档数。find方法根据条件查询数据,返回满足条件的文档集合,并可以指定分页大小和页码。
以上是一个简单的MongoDB增删改查类的实现,根据需求可以对其进行扩展和优化。
相关推荐
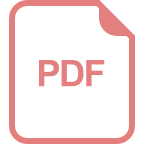



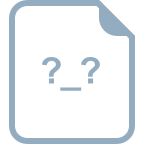
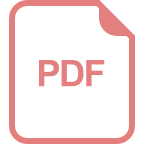
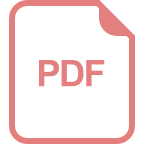
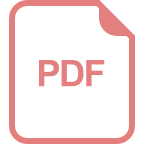
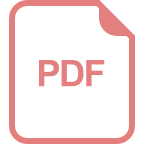
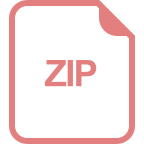
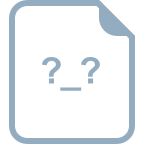