用PYTHON 写一个类,可以进行MONGO数据库的增删改查,包含分页查询,请直接展示代码和示例
时间: 2023-09-22 18:06:21 浏览: 71
### 回答1:
好的,以下是一个使用 Python 连接 MongoDB 数据库进行增删改查的类的示例代码:
```python
import pymongo
class MongoDB:
def __init__(self, host, port, database):
self.client = pymongo.MongoClient(host, port)
self.db = self.client[database]
def insert_one(self, collection, document):
"""插入一条记录"""
self.db[collection].insert_one(document)
def insert_many(self, collection, documents):
"""插入多条记录"""
self.db[collection].insert_many(documents)
def find_one(self, collection, query):
"""查询一条记录"""
return self.db[collection].find_one(query)
def find(self, collection, query, page_size=10, page_num=1):
"""分页查询多条记录"""
skip = (page_num - 1) * page_size
return self.db[collection].find(query).skip(skip).limit(page_size)
def update_one(self, collection, query, update):
"""更新一条记录"""
self.db[collection].update_one(query, update)
def update_many(self, collection, query, update):
"""更新多条记录"""
self.db[collection].update_many(query, update)
def delete_one(self, collection, query):
"""删除一条记录"""
self.db[collection].delete_one(query)
def delete_many(self, collection, query):
"""删除多条记录"""
self.db[collection].delete_many(query)
```
以下是使用这个类的示例:
```python
# 实例化类
mongodb = MongoDB('localhost', 27017, 'test')
# 插入一条记录
mongodb.insert_one('users', {'name': 'Alice', 'age': 20})
# 插入多条记录
mongodb.insert_many('users', [{'name': 'Bob', 'age': 21}, {'name': 'Charlie', 'age': 22}])
# 查询一条记录
print(mongodb.find_one('users', {'name': 'Alice'}))
# 分页查询多条记录
### 回答2:
下面是一个用 Python 写的类,可以进行 MONGO 数据库的增删改查,并包含分页查询的示例代码:
```python
from pymongo import MongoClient
class MongoHandler:
def __init__(self, host='localhost', port=27017):
self.client = MongoClient(host, port)
self.db = self.client['mydatabase']
self.collection = self.db['mycollection']
def insert(self, data):
result = self.collection.insert_one(data)
return result.inserted_id
def find(self, query):
results = self.collection.find(query)
return [result for result in results]
def update(self, filter_query, update_query):
result = self.collection.update_many(filter_query, update_query)
return result.modified_count
def delete(self, query):
result = self.collection.delete_many(query)
return result.deleted_count
def find_with_pagination(self, query, page_size, page_number):
skip_count = page_size * (page_number - 1)
results = self.collection.find(query).skip(skip_count).limit(page_size)
return [result for result in results]
# 示例代码
if __name__ == '__main__':
mongo_handler = MongoHandler()
# 插入数据
data = {
'name': 'John',
'age': 25,
'country': 'USA'
}
inserted_id = mongo_handler.insert(data)
print(f'Inserted ID: {inserted_id}')
# 查询数据
query = {'age': {'$gte': 20}}
results = mongo_handler.find(query)
for result in results:
print(result)
# 更新数据
filter_query = {'country': 'USA'}
update_query = {'$set': {'age': 30}}
modified_count = mongo_handler.update(filter_query, update_query)
print(f'Modified count: {modified_count}')
# 删除数据
delete_query = {'age': {'$lt': 30}}
deleted_count = mongo_handler.delete(delete_query)
print(f'Deleted count: {deleted_count}')
# 分页查询数据
query = {}
page_size = 2
page_number = 2
results = mongo_handler.find_with_pagination(query, page_size, page_number)
for result in results:
print(result)
```
这段代码定义了一个 `MongoHandler` 类,初始化时连接到本地的 MongoDB 数据库,并操作 `mydatabase` 数据库中的 `mycollection` 集合。类中的 `insert` 方法用于插入数据,`find` 方法用于查询数据,`update` 方法用于更新数据,`delete` 方法用于删除数据。另外还定义了一个 `find_with_pagination` 方法,用于分页查询数据。
在示例代码中,首先使用 `insert` 方法插入了一条数据,然后使用 `find` 方法查询了年龄大于等于 20 的数据,使用 `update` 方法将所有国家为 USA 的数据的年龄更新为 30,使用 `delete` 方法删除了年龄小于 30 的数据。最后使用 `find_with_pagination` 方法进行了分页查询,每页显示 2 条数据,查找第 2 页的数据。以上操作均打印出了相应的结果。
### 回答3:
下面是一个用Python写的可以进行Mongo数据库增删改查的类,包含分页查询的示例代码:
```python
from pymongo import MongoClient
class MongoDB:
def __init__(self, db_name, collection_name):
self.client = MongoClient()
self.db = self.client[db_name]
self.collection = self.db[collection_name]
def insert(self, data):
self.collection.insert_one(data)
def delete(self, query):
self.collection.delete_many(query)
def update(self, query, update_data):
self.collection.update_many(query, {"$set": update_data})
def find(self, query, projection=None, limit=None, skip=None):
cursor = self.collection.find(query, projection)
if limit:
cursor = cursor.limit(limit)
if skip:
cursor = cursor.skip(skip)
results = []
for doc in cursor:
results.append(doc)
return results
# 示例代码
# 创建MongoDB类的实例
mongo = MongoDB("test_database", "test_collection")
# 插入数据
data1 = {"name": "Alice", "age": 25}
mongo.insert(data1)
data2 = {"name": "Bob", "age": 27}
mongo.insert(data2)
data3 = {"name": "Charlie", "age": 30}
mongo.insert(data3)
# 查询所有数据
all_data = mongo.find({})
for data in all_data:
print(data)
# 查询年龄大于等于26的数据,只返回name字段
query = {"age": {"$gte": 26}}
projection = {"name": 1, "_id": 0}
data_with_condition = mongo.find(query, projection)
for data in data_with_condition:
print(data)
# 删除年龄大于等于26的数据
mongo.delete(query)
# 更新年龄小于30的数据,将age字段加1
update_query = {"age": {"$lt": 30}}
update_data = {"age": {"$inc": 1}}
mongo.update(update_query, update_data)
```
上述代码示例了如何使用MongoDB类进行增删改查操作。更具体的使用方式和参数说明可以参考PyMongo的官方文档:https://pymongo.readthedocs.io/
相关推荐
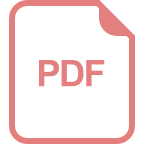
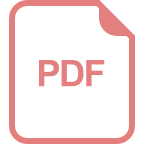













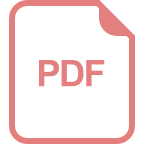
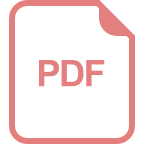