用PYTHON 写一个类,可以进行MONGO数据库的增删改查。
时间: 2023-08-31 11:41:24 浏览: 210
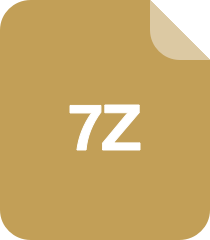
python界面实现数据库增删改查

### 回答1:
你好! 这是一个简单的类,可以连接到 MongoDB 数据库并进行增删改查操作。这需要使用 PyMongo 库,该库提供了用于连接和操作 MongoDB 数据库的功能。
首先,你需要在终端或命令行中使用以下命令安装 PyMongo 库:
```
pip install pymongo
```
然后,你可以使用以下代码创建一个类,该类具有连接到 MongoDB 数据库的方法,并具有四个方法,用于增加、删除、修改和查询数据库中的数据。
```python
import pymongo
class MongoDB:
def __init__(self, host, port, db_name):
self.client = pymongo.MongoClient(host, port)
self.db = self.client[db_name]
def insert_one(self, collection, data):
self.db[collection].insert_one(data)
def insert_many(self, collection, data_list):
self.db[collection].insert_many(data_list)
def delete_one(self, collection, query):
self.db[collection].delete_one(query)
def delete_many(self, collection, query):
self.db[collection].delete_many(query)
def update_one(self, collection, query, update):
self.db[collection].update_one(query, update)
def update_many(self, collection, query, update):
self.db[collection].update_many(query, update)
def find_one(self, collection, query):
return self.db[collection].find_one(query)
def find(self, collection, query):
return self.db[collection].find(query)
```
使用这个类的方法如下:
```python
# 创建 MongoDB 对象
mongodb = MongoDB('localhost', 27017, 'mydb')
# 插入一条数据
data = {'name': 'John', 'age': 30}
mongodb.insert_one('users', data)
# 插入多条数据
data_list = [{'name': 'Alice', 'age': 25}, {'name':
### 回答2:
下面是一个使用Python编写的类,可以进行MongoDB数据库的增删改查操作:
```python
from pymongo import MongoClient
class MongoDBConnector:
def __init__(self, host, port, database):
self.client = MongoClient(host, port)
self.db = self.client[database]
def insert(self, collection, data):
self.db[collection].insert_one(data)
def find(self, collection, query):
return self.db[collection].find(query)
def update(self, collection, query, update_data):
self.db[collection].update(query, {"$set": update_data})
def delete(self, collection, query):
self.db[collection].delete_one(query)
# 使用示例:
connector = MongoDBConnector('localhost', 27017, 'myDB')
collection = 'myCollection'
# 添加数据
data = {'name': 'John', 'age': 30}
connector.insert(collection, data)
# 查询数据
query = {'name': 'John'}
results = connector.find(collection, query)
for result in results:
print(result)
# 更新数据
query = {'name': 'John'}
update_data = {'age': 31}
connector.update(collection, query, update_data)
# 删除数据
query = {'name': 'John'}
connector.delete(collection, query)
```
这个类通过使用pymongo库来连接MongoDB数据库,并提供了插入、查询、更新和删除操作的方法。你可以根据自己的需要调用这些方法来对数据库进行操作。在使用时,你需要提供MongoDB的主机地址、端口和数据库名称,并将数据集合的名称作为参数传递给方法。
阅读全文
相关推荐
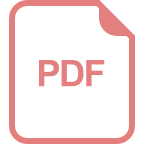




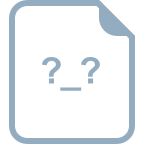
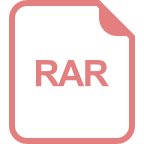





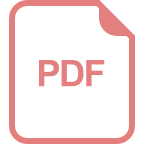
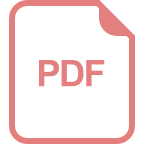
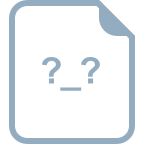