从doc格式文件中提出含有多个关键词的句子,并将句子存入sqlserver数据库,使用python代码
时间: 2023-01-28 07:25:59 浏览: 135
这是一个可以使用 Python 代码实现的方案:
1. 使用 Python 的文档处理库,例如 python-docx,读取 doc 文件并解析其中的文本。
2. 对文本进行遍历,使用正则表达式或其他方法提取出含有多个关键词的句子。
3. 使用 Python 的数据库驱动程序,例如 pyodbc,连接到 SQL Server 数据库。
4. 创建一个数据表,用于存储提取出的句子。
5. 将提取出的句子插入到数据表中。
以下是一个示例代码,假设已经安装了 python-docx 和 pyodbc 库:
```python
import docx
import pyodbc
import re
# 连接到 SQL Server 数据库
cnxn = pyodbc.connect('DRIVER={ODBC Driver 17 for SQL Server};'
'SERVER=localhost;'
'DATABASE=testdb;'
'UID=user;'
'PWD=password')
cursor = cnxn.cursor()
# 创建数据表
cursor.execute("""
CREATE TABLE Sentences (
id INT IDENTITY PRIMARY KEY,
sentence NVARCHAR(MAX)
)
""")
cnxn.commit()
# 读取 doc 文件并解析文本
document = docx.Document('document.docx')
text = ""
for paragraph in document.paragraphs:
text += paragraph.text
# 提取含有多个关键词的句子
keywords = ['keyword1', 'keyword2']
pattern = r'\b(' + '|'.join(keywords) + r')\b'
sentences = re.findall(pattern, text)
# 将句子插入到数据表中
for sentence in sentences:
cursor.execute("INSERT INTO Sentences (sentence) VALUES (?)", sentence)
cnxn.commit()
# 关闭数据库连接
cursor.close()
cnxn.close()
```
阅读全文
相关推荐
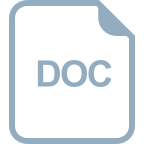
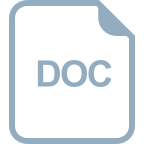
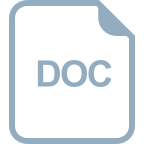




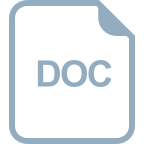
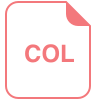
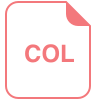
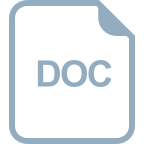
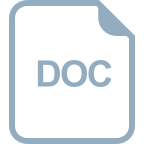
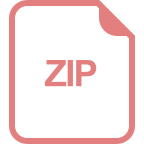
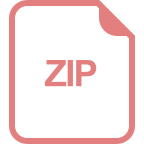