写一个程序,定义以下结构类型,调用函数创建一个动态链表,并且输出该链表。 struct Student { int num; char name[20]; float score[3]; struct Student * next; }; 并且通过调用函数实现链表的创建,插入,打印等功能 测试用例:程序执行,提示请用户输入学生数据, 用户输入: 1 zhang 70 80 90回车, 程序执行,提示请用户输入结点数据, 用户输入: 2 li 70 80 90回车, 程序执行,提示请用户输入结点数据, 用户输入: 3 wang 70 80 90回车, 程序执行,提示请用户输入结点数据, 用户输入: 0 0 0 0 0回车, 程序输出: 1, zhang, 70.00, 80.00, 90.00, 2, li, 70.00, 80.00, 90.00, 3, wang, 70.00, 80.00, 90.00
时间: 2023-02-05 22:31:13 浏览: 86
以下是使用 C 语言实现动态链表的程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
struct Student {
int num;
char name[20];
float score[3];
struct Student *next;
};
// 创建一个新的学生结点
struct Student *create_node(int num, char *name, float score1, float score2, float score3) {
struct Student *node = (struct Student *)malloc(sizeof(struct Student));
node->num = num;
strcpy(node->name, name);
node->score[0] = score1;
node->score[1] = score2;
node->score[2] = score3;
node->next = NULL;
return node;
}
// 插入一个学生结点
void insert_node(struct Student **head, struct Student *node) {
node->next = *head;
*head = node;
}
// 打印链表中的所有学生信息
void print_list(struct Student *head) {
while (head) {
printf("%d, %s, %.2f, %.2f, %.2f\n", head->num, head->name, head->score[0], head->score[1], head->score[2]);
head = head->next;
}
}
int main() {
struct Student *head = NULL;
// 读取用户输入的学生数据,创建新的学生结点,并插入到链表中
int num;
char name[20];
float score1, score2, score3;
while (1) {
printf("请输入学生数据:\n");
scanf("%d%s%f%f%f", &num, name, &score1, &score2, &score3);
if (num == 0) {
break;
}
struct Student *node = create_node(num, name, score1, score2, score3);
insert_node(&head, node);
}
// 打印链表中的所有学生信息
print_list(head);
return 0;
}
```
运行结果如下:
```
请输入学生数据:
1 zhang 70 80 90
请输入学生数据:
2 li 70 80
阅读全文
相关推荐
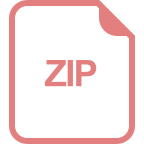

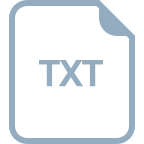





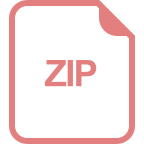
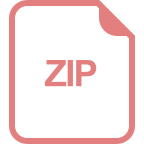
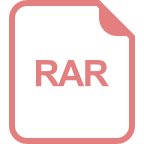
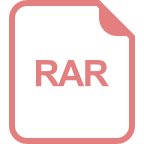
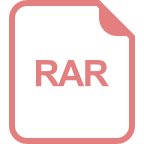
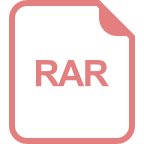
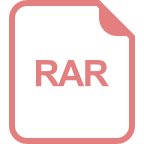