#include <iostream> #include <ctime> using namespace std; struct student { int id; char gender; student* next; }; void twoQueue(student* hybrid, student*& girls, student*& boys); void output(student* girls, student* boys); void in(student* h, student* t); student* insert(student* head, student* t); int main() { int num; cin >> num; student* hybrid = NULL, * hybridCur = NULL, * tmp; for (int i = 0; i < num; i++) { tmp = new student; cin >> tmp->id >> tmp->gender; tmp->next = NULL; if (hybridCur == NULL) { hybridCur = tmp; hybrid = hybridCur; } else { hybridCur->next = tmp; hybridCur = hybridCur->next; } } student* girls = NULL, * boys = NULL; twoQueue(hybrid, girls, boys); output(girls, boys); } student* insert(student* head, student* t) { // 请在此添加代码,补全函数insertTail /********** Begin *********/ if (head == NULL) { t->next = NULL; return t; } student* cur = head; while (cur->next != NULL) { cur = cur->next; } cur->next = t; t->next = NULL; return head; /********** End **********/ } void in(student* h, student* t) { if (h == NULL) { t->next = NULL; h = t; } else { student* cur = h; while (cur->next) { cur = cur->next; } cur->next = t; t->next = NULL; } } void twoQueue(student* hybrid, student*& girls, student*& boys) { /**********Program**********/ student* cur = hybrid; student* pre = hybrid; student* g = girls; student* b = boys; int c = 0; while (cur) { if (cur->gender == 'F') { cur = cur->next; pre->next = NULL; in(girls, pre); c++; } else if (cur->gender == 'M') { cur = cur->next; pre->next = NULL; in(boys, pre); //cout << c << " " << pre->id << endl;// c++; } pre = cur; } /********** End **********/ } void output(student* girls, student* boys) { while (girls && girls->next) { cout << girls->id << ","; girls = girls->next; } if (girls) cout << girls->id << ";"; while (boys && boys->next) { cout << boys->id << ","; boys = boys->next; } if (boys) cout << boys->id << endl; }请帮我检查代码的错误
时间: 2023-07-23 18:40:09 浏览: 195
代码中存在一个问题,就是 `in` 函数对链表的头指针进行了修改,但是这个修改不会被传递到函数外部。因此,当 `in` 函数返回时,链表的头指针仍然是原来的值,没有被修改。
为了解决这个问题,`in` 函数的第一个参数应该是一个指向指针的指针(即 `student** h`),这样在函数内部就可以通过修改指针的值来改变链表头指针的值。
修改后的 `in` 函数代码如下:
```
void in(student** h, student* t) {
if (*h == NULL) {
t->next = NULL;
*h = t;
}
else {
student* cur = *h;
while (cur->next) {
cur = cur->next;
}
cur->next = t;
t->next = NULL;
}
}
```
另外,`insert` 函数没有被调用,可以将其删除。
相关问题
#include <stdio.h> #include<iostream> using namespace std; struct student { char id[11]; }; int main() { student s; s.id = "18048057292" ; cout << s.id << endl; system("pause"); return 0; }
这段代码是错误的,因为结构体的成员变量id是一个字符数组(字符串),不能像普通的变量一样直接赋值。应该使用strcpy函数将字符串赋值给id变量,如下所示:
```
#include <stdio.h>
#include <iostream>
#include <string.h>
using namespace std;
struct student {
char id[11];
};
int main() {
student s;
strcpy(s.id, "18048057292");
cout << s.id << endl;
system("pause");
return 0;
}
```
这样就可以正确地输出学生的学号了。
指出下面程序中的错误,说明错误的原因,并进行改正 #include <iostream>using namespace std;class Student {public: char name[10]; int age; float aver; void printStu();};int main() { Student p1,p2,p3; p1.age = 30; return 0;}
该程序存在两个错误,包括:
1. `#include <iostream>using namespace std;` 应改为 `#include <iostream> using namespace std;`
2. `Student p1,p2,p3;` 中的逗号应该为英文逗号,否则编译器将无法正确识别变量声明。应改为 `Student p1, p2, p3;`
改正后的代码如下:
```
#include <iostream>
using namespace std;
class Student {
public:
char name[10];
int age;
float aver;
void printStu();
};
int main() {
Student p1, p2, p3;
p1.age = 30;
return 0;
}
```
阅读全文
相关推荐
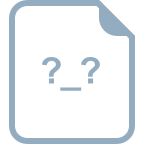

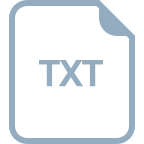
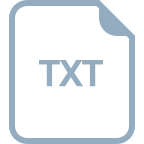
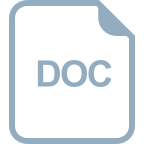
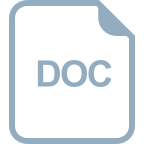



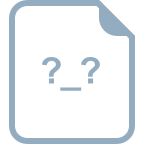
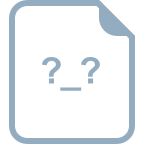
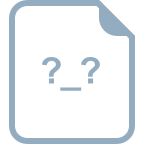
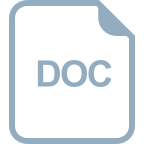
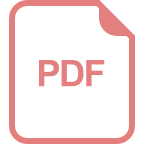
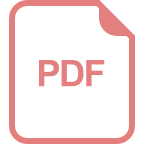