#include <stdio.h> #include<iostream> using namespace std; struct student { char id[11]; }; int main() { student s; s.id = "18048057292" ; cout << s.id << endl; system("pause"); return 0; }
时间: 2024-04-04 19:32:36 浏览: 16
这段代码是错误的,因为结构体的成员变量id是一个字符数组(字符串),不能像普通的变量一样直接赋值。应该使用strcpy函数将字符串赋值给id变量,如下所示:
```
#include <stdio.h>
#include <iostream>
#include <string.h>
using namespace std;
struct student {
char id[11];
};
int main() {
student s;
strcpy(s.id, "18048057292");
cout << s.id << endl;
system("pause");
return 0;
}
```
这样就可以正确地输出学生的学号了。
相关问题
优化以下代码,要求:班级成绩管理系统 (1)利用结构体和简单的链表,实现一个班学生成绩的管理,至少可以输入20个学生的成绩。 (2)成绩管理功能包括:输入、输出,利用new和delete运算符添加和删除班级成绩 (1)利用结构体和简单的链表(关于链表的知识,自己查阅资料学习),实现一个班学生成绩的管理。#include<stdio.h> #include<iostream> #include<windows.h> using namespace std; typedef struct student { char name[20]; int score; student* next; }stu; stu* head; void input() { stu* p1, * p2; p1 = (stu*)new stu; head = p1; while (p1 != NULL) { cout << "输入姓名" << endl; cin >> p1->name; cout << "输入成绩" << endl; cin >> p1->score; cout << "是否继续输入?(1.是 2.否)"; int a; cin >> a; if (a == 1) { p2 = p1; p1 = (stu*)new stu; p2->next = p1; continue; } else if (a == 2) { p1->next = NULL; break; } } } void listprint() { stu* p1 = head; while (p1 != NULL) { cout << 1; cout << "姓名: " << p1->name << " 成绩:" << p1->score << endl; p1 = p1->next; } } void shanchu() { char c[20]; cout << "请输入删除的学生姓名" << endl; cin >> c; stu* p1 = head, * p2; while (p1 != NULL) { p2 = p1->next; if (strcmp(p2->name, c) == 0) { p1->next = p2->next; break; }p1 = p1->next; } } int main() { int a = 0; while (1) { cout << "1.输入成绩 2.输出成绩 3.删除" << endl;cin >> a; switch (a) { case 1: { input(); system ("cls"); continue; } case 2: { listprint(); continue; } case 3: { shanchu(); continue; } default: { break; } } break; } }
以下是优化后的代码:
#include <iostream>
#include <string>
using namespace std;
struct Student {
string name;
int score;
Student* next;
};
void addStudent(Student*& head, string name, int score) {
Student* newStudent = new Student;
newStudent->name = name;
newStudent->score = score;
newStudent->next = head;
head = newStudent;
}
void deleteStudent(Student*& head, string name) {
if (head == NULL) {
return;
}
if (head->name == name) {
Student* temp = head;
head = head->next;
delete temp;
return;
}
Student* current = head;
while (current->next != NULL && current->next->name != name) {
current = current->next;
}
if (current->next == NULL) {
return;
}
Student* temp = current->next;
current->next = current->next->next;
delete temp;
}
void printStudents(Student* head) {
while (head != NULL) {
cout << head->name << " " << head->score << endl;
head = head->next;
}
}
int main() {
Student* head = NULL;
int choice;
string name;
int score;
while (true) {
cout << "1. Add student" << endl;
cout << "2. Delete student" << endl;
cout << "3. Print students" << endl;
cout << "4. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "Enter student name: ";
cin >> name;
cout << "Enter student score: ";
cin >> score;
addStudent(head, name, score);
break;
case 2:
cout << "Enter student name: ";
cin >> name;
deleteStudent(head, name);
break;
case 3:
printStudents(head);
break;
case 4:
return 0;
default:
cout << "Invalid choice" << endl;
}
}
return 0;
}
优化后的代码使用了指针和动态内存分配,可以更好地管理学生信息。同时,使用了switch语句来实现菜单功能,代码更加简洁易懂。
#include <iostream>#include <iomanip> // 用于输出保留小数位数using namespace std;const int MAXN = 50;struct Student { char id[11]; double score;} stu[MAXN];int main() { int n; cin >> n; for (int i = 0; i < n; i++) { cin >> stu[i].id >> stu[i].score; } for (int i = 0; i < n; i++) { cout << stu[i].id << " " << fixed << setprecision(1) << stu[i].score << endl; // 输出保留1位小数 } return 0;}用c语言实现程序
抱歉,你给出的是 C++ 的代码,不是 C 语言的代码。以下是将其转换为 C 语言的代码:
```c
#include <stdio.h>
#define MAXN 50
struct Student {
char id[11];
double score;
};
int main() {
int n, i;
struct Student stu[MAXN];
scanf("%d", &n);
for (i = 0; i < n; i++) {
scanf("%s %lf", stu[i].id, &stu[i].score);
}
for (i = 0; i < n; i++) {
printf("%s %.1lf\n", stu[i].id, stu[i].score);
}
return 0;
}
```
注意,C 语言中没有 `cin` 和 `cout`,需要使用 `scanf` 和 `printf` 进行输入输出。另外,C 语言中的结构体需要在前面加上 `struct` 关键字,而 C++ 中则不需要。
相关推荐
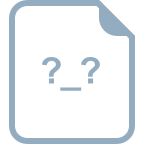
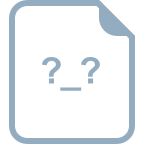












