将以下代码从C++转换到C代码:#include<iostream> #include<string> using namespace std; //使用string容器需要加上这一句 const int maxn=1000; struct student{ string ID; //准考证 int shiji,kaoshi; //试机座位号 考试座位号 }k[maxn]; //所有的考生 int main() { int n,m,cha; //考试人数 待查次数 待查座位号 cin>>n; for(int i=0;i<n;i++){ cin>>k[i].ID>>k[i].shiji>>k[i].kaoshi; //录入考生信息 } cin>>m; for(int i=0;i<m;i++){ cin>>cha; for(int i=0;i<n;i++){ if(cha==k[i].shiji ){ cout<<k[i].ID<<' '<<k[i].kaoshi<<endl;//如果查到则按照格式输出相应信息。 break; //已经查到则推出此循环。以加快速度。 } } } }
时间: 2024-02-05 12:03:23 浏览: 24
以下是将该代码从C++转换到C的结果:
```
#include <stdio.h>
#include <string.h>
#define MAXN 1000
struct student {
char ID[20];
int shiji;
int kaoshi;
} k[MAXN];
int main() {
int n, m, cha;
scanf("%d", &n);
for (int i = 0; i < n; i++) {
scanf("%s %d %d", k[i].ID, &k[i].shiji, &k[i].kaoshi);
}
scanf("%d", &m);
for (int i = 0; i < m; i++) {
scanf("%d", &cha);
for (int j = 0; j < n; j++) {
if (cha == k[j].shiji) {
printf("%s %d\n", k[j].ID, k[j].kaoshi);
break;
}
}
}
return 0;
}
```
请注意,由于C语言中没有string类型,因此我们使用了字符数组来代替。另外,C语言中不能像C++那样使用cin和cout进行输入输出,而是使用了scanf和printf函数。
相关问题
#include <iostream> #include <queue> #include <string> using namespace std;对代码进行注释
代码注释如下:
```c++
#include <iostream> // 引入输入输出流库
#include <queue> // 引入队列库
#include <string> // 引入字符串库
using namespace std; // 使用 std 命名空间
// 主函数
int main() {
queue<string> q; // 定义一个字符串队列 q
// 往队列中添加元素
q.push("apple");
q.push("banana");
q.push("orange");
// 输出队列头部元素
cout << q.front() << endl; // 输出:apple
// 弹出队列头部元素
q.pop();
// 输出队列头部元素
cout << q.front() << endl; // 输出:banana
// 输出队列中元素的个数
cout << "The size of the queue is " << q.size() << endl; // 输出:The size of the queue is 2
return 0; // 返回 0 表示程序正常结束
}
```
解释代码# include < iostream > # include < string > # include < vector > # include < iomanip > using namespace std ;
这段代码是 C++ 中的预处理指令,用于引入头文件并进行命名空间声明。
- `#include <iostream>` 引入输入输出流库,用于控制台输入输出。
- `#include <string>` 引入字符串库,用于处理字符串操作。
- `#include <vector>` 引入向量库,用于动态数组操作。
- `#include <iomanip>` 引入输入输出流格式化库,用于格式化控制台输出。
`using namespace std;` 是命名空间声明,表示使用标准命名空间(std)中的所有函数和类,避免了每次使用时都要写 std:: 的麻烦。
相关推荐
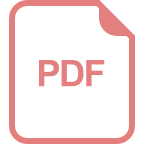
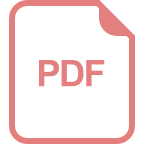
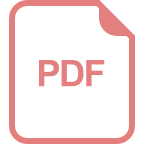













