写一个程序,定义以下结构类型,调用函数创建一个动态链表,并且输出该链表。 struct Student { int num; char name[20]; float score[3]; struct Student * next; }; 并且通过调用函数实现链表的创建,插入,打印等功能 测试用例:程序执行,提示请用户输入学生数据, 用户输入: 1 zhang 70 80 90回车, 程序执行,提示请用户输入结点数据, 用户输入: 2 li 70 80 90回车, 程序执行,提示请用户输入结点数据, 用户输入: 3 wang 70 80 90回车, 程序执行,提示请用户输入结点数据, 用户输入: 0 0 0 0 0回车, 程序输出: 1, zhang, 70.00, 80.00, 90.00, 2, li, 70.00, 80.00, 90.00, 3, wang, 70.00, 80.00, 90.00
时间: 2023-02-05 22:31:14 浏览: 61
首先,需要定义一个结构体变量来表示动态链表的头结点,该结点不包含有效数据,只用于指向第一个有效结点:
struct Student * head = NULL;
接下来,可以定义一个函数来创建新的结点,并将其插入到链表的末尾:
void insertNode(int num, char * name, float score1, float score2, float score3) {
// 创建新结点
struct Student * newNode = (struct Student *) malloc(sizeof(struct Student));
newNode->num = num;
strcpy(newNode->name, name);
newNode->score[0] = score1;
newNode->score[1] = score2;
newNode->score[2] = score3;
newNode->next = NULL;
// 如果链表为空,将新结点设为头结点
if (head == NULL) {
head = newNode;
}
// 否则,找到链表的末尾并插入新结点
else {
struct Student * cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
}
最后,可以定义一个函数来遍历链表并打印每个结点的信息:
void printList() {
struct Student * cur = head;
while (cur != NULL) {
printf("%d, %s, %.2f, %.2f, %.2f, ", cur->num, cur->name, cur->score[0], cur->score[1], cur->score[2]);
cur = cur->next;
}
printf("\n");
}
综上,可以使用以下代码实现题目中给出的功能:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
int num;
char name[20];
float score[3];
struct Student * next;
};
struct Student * head = NULL;
void insertNode(int num, char * name,
阅读全文
相关推荐

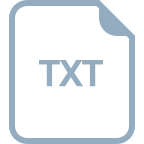





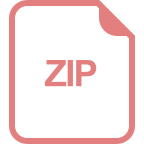
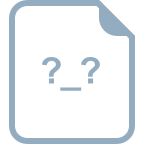
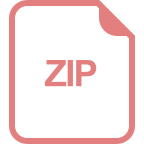