迷宫求解C语言完整代码利用栈
时间: 2023-09-19 20:06:39 浏览: 89
### 回答1:
好的,下面是用 C 语言实现的迷宫求解代码。这里我们使用栈来存储路径。
首先,我们需要定义一些常量和结构体:
```
#define N 10 // 迷宫的大小
#define M 2 // 走到下一步所需的步数
#define S 2 // 两种走法:向左或向右
// 定义点的坐标结构体
struct point {
int x, y;
} st;
// 定义栈的结构体
struct stack {
point data[N * N]; // 栈内存储的点
int top; // 栈顶元素的下标
} stk;
```
然后,我们可以实现一个函数来判断某个点是否可以走:
```
// 判断点 p 是否可以走
int pd(point p) {
// 判断是否越界
if (p.x < 0 || p.x >= N || p.y < 0 || p.y >= N) return 0;
// 判断是否是障碍
if (maze[p.x][p.y] == 1) return 0;
// 判断是否已经走过
if (book[p.x][p.y] == 1) return 0;
return 1;
}
```
接下来,我们可以实现一个函数来搜索迷宫:
```
// 搜索迷宫
void dfs(point p) {
// 将当前点压入栈中
stk.data[stk.top++] = p;
// 标记当前点已经走过
book[p.x][p.y] = 1;
// 如果到达终点,则输出路径
if (p.x == N - 1 && p.y == N - 1) {
for (int i = 0; i < stk.top; i++) {
printf("(%d,
### 回答2:
以下是一个使用栈求解迷宫问题的C语言完整代码:
```c
#include <stdio.h>
#define MAX_SIZE 100
typedef struct {
int x; //位置的横坐标
int y; //位置的纵坐标
int direction; //当前位置的前进方向
} Position;
int main() {
int maze[5][5] = { //定义一个5x5的迷宫,1表示墙,0表示通路
{0, 1, 1, 0, 0},
{0, 0, 0, 1, 0},
{1, 1, 0, 1, 0},
{1, 1, 0, 0, 0},
{0, 0, 0, 1, 0}
};
int visited[5][5] = {0}; //定义一个5x5的数组记录已经访问过的位置
Position stack[MAX_SIZE]; //定义一个大小为MAX_SIZE的栈
int top = -1; //初始栈为空
Position startPos, endPos;
startPos.x = 0;
startPos.y = 0;
startPos.direction = 0;
endPos.x = 4;
endPos.y = 4;
stack[++top] = startPos; //将起始位置入栈
while (top != -1) { //当栈非空时,继续搜索
Position curPos = stack[top]; //获取栈顶位置
visited[curPos.x][curPos.y] = 1; //标记当前位置为已访问
if (curPos.x == endPos.x && curPos.y == endPos.y) { //找到终点,返回路径
for (int i = 0; i <= top; i++) {
printf("(%d, %d) ", stack[i].x, stack[i].y);
}
printf("\n");
return 0;
}
int i;
for (i = curPos.direction; i <= 3; i++) { //按照右、下、左、上的顺序依次尝试移动
Position nextPos;
nextPos.direction = i + 1; //更新下个位置的前进方向
switch (i) {
case 0: //向右
nextPos.x = curPos.x + 1;
nextPos.y = curPos.y;
break;
case 1: //向下
nextPos.x = curPos.x;
nextPos.y = curPos.y + 1;
break;
case 2: //向左
nextPos.x = curPos.x - 1;
nextPos.y = curPos.y;
break;
case 3: //向上
nextPos.x = curPos.x;
nextPos.y = curPos.y - 1;
break;
}
if (nextPos.x >= 0 && nextPos.x < 5 //判断是否越界
&& nextPos.y >= 0 && nextPos.y < 5
&& maze[nextPos.x][nextPos.y] == 0
&& visited[nextPos.x][nextPos.y] == 0) { //判断是否为通路且未访问过
stack[++top] = nextPos; //将下个位置入栈
break; //搜索下一个位置
}
}
if (i > 3) { //上个位置的所有方向都无法继续移动,退栈继续搜索
top--;
}
}
printf("无法找到路径。\n");
return 0;
}
```
该代码通过使用栈来实现深度优先搜索(DFS)的思想,从起点开始不断尝试移动到相邻的可走位置,直到找到终点或无法继续移动为止。同时,利用visited数组来记录已经访问过的位置,避免重复访问。当找到终点时,将栈中的位置逐个输出,即为迷宫的求解路径。
### 回答3:
迷宫求解算法是一种常见的问题,可以使用深度优先搜索(DFS)结合栈的数据结构来解决。下面是一个C语言中使用栈来求解迷宫的完整代码。
```c
#include<stdio.h>
#include<stdlib.h>
#define ROW 8 //迷宫行数
#define COL 8 //迷宫列数
// 定义坐标结构体
typedef struct {
int x;
int y;
} Coordinate;
// 定义栈的结构体
typedef struct {
Coordinate position[ROW * COL];
int top;
} Stack;
// 初始化栈
void initStack(Stack* stack) {
stack->top = -1;
}
// 栈是否为空
int isStackEmpty(Stack* stack) {
return stack->top == -1;
}
// 入栈
void push(Stack* stack, Coordinate coord) {
stack->position[++stack->top] = coord;
}
// 出栈
Coordinate pop(Stack* stack) {
return stack->position[stack->top--];
}
// 迷宫求解函数
void mazeSolver(int maze[ROW][COL], Coordinate entry, Coordinate exit) {
Stack stack;
Coordinate current = entry;
initStack(&stack);
push(&stack, current);
while (!isStackEmpty(&stack)) {
current = pop(&stack);
int x = current.x;
int y = current.y;
// 如果到达出口,则退出循环
if (x == exit.x && y == exit.y) {
printf("Successfully solved the maze!\n");
return;
}
// 标记当前位置已被访问
maze[x][y] = 2;
// 下一步可能的方向
Coordinate next[4] = {
{x-1, y}, // 上
{x, y+1}, // 右
{x+1, y}, // 下
{x, y-1} // 左
};
// 将下一步可能的位置入栈
for (int i = 0; i < 4; i++) {
int nextX = next[i].x;
int nextY = next[i].y;
// 判断下一步是否为合法位置
if (nextX >= 0 && nextY >= 0 && nextX < ROW && nextY < COL && maze[nextX][nextY] == 0) {
Coordinate nextCoord = {nextX, nextY};
push(&stack, nextCoord);
}
}
}
printf("Failed to solve the maze!\n");
}
int main() {
int maze[ROW][COL] = {
{1, 1, 1, 1, 1, 1, 1, 1},
{1, 0, 0, 1, 0, 0, 0, 1},
{1, 0, 0, 1, 0, 0, 1, 1},
{1, 1, 0, 0, 1, 1, 1, 1},
{1, 1, 1, 0, 0, 0, 0, 1},
{1, 1, 1, 1, 1, 1, 0, 1},
{1, 0, 0, 0, 0, 0, 0, 1},
{1, 1, 1, 1, 1, 1, 1, 1}
};
Coordinate entry = {1, 1};
Coordinate exit = {6, 6};
mazeSolver(maze, entry, exit);
return 0;
}
```
这段代码首先定义了迷宫的行数和列数,以及坐标和栈的数据结构。迷宫求解函数中使用深度优先搜索算法来遍历迷宫并寻找路径,通过入栈和出栈操作来回溯遍历过程。在一个8x8的迷宫中,1代表墙壁,0代表通路,2代表已访问过的路径。程序通过栈来存储下一步可能的位置坐标,直到找到出口或者所有可能路径都已访问完。主函数中定义了一个迷宫数组,以及入口和出口的坐标,然后调用迷宫求解函数来解决迷宫问题。
相关推荐
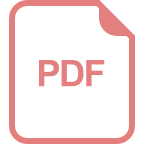
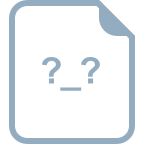












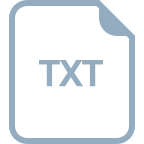