请用C语言编写一个迷宫求解的程序,利用栈
时间: 2023-02-21 11:35:36 浏览: 39
好的,我可以帮助你写一个 C 语言的迷宫求解程序。首先,你需要建立一个存储迷宫信息的二维数组,并且给出起点和终点的坐标。然后,你可以用栈来记录当前位置的坐标以及上一个位置的坐标,并用 DFS 算法来搜索迷宫的路径。
具体来说,你可以先从起点开始,将起点坐标压入栈中,然后分别尝试向上下左右四个方向移动,如果当前位置不是墙壁且没有走过,就将该位置的坐标压入栈中并标记为已经走过。如果到达了终点,则找到了一条从起点到终点的路径;如果搜索完了所有的方向都无法到达终点,就弹出栈顶元素,返回上一个位置,继续搜索。
下面是一个示例代码,希望对你有帮助:
```
#include <stdio.h>
#include <stdlib.h>
#define ROW 5
#define COL 5
int maze[ROW][COL] = {
{0, 0, 1, 0, 0},
{0, 0, 0, 0, 0},
{0, 0, 1, 1, 0},
{0, 1, 0, 0, 0},
{0, 0, 0, 1, 0}
};
typedef struct {
int x;
int y;
} Pos;
void push(Pos *stack, int *top, int x, int y) {
stack[*top].x = x;
stack[*top].y = y;
(*top)++;
}
相关问题
用1表示死路,用0表示通路,使用C语言编写一个简单的用栈求解迷宫问题所有路径及最短路径程序
以下是一个基于栈的简单迷宫问题求解程序,包括所有路径和最短路径的求解:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAXROW 5 // 迷宫的行数
#define MAXCOL 5 // 迷宫的列数
// 迷宫地图
int maze[MAXROW][MAXCOL] = {
{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 1, 0}
};
// 定义坐标结构体
typedef struct {
int row;
int col;
} Position;
// 定义栈结构体
typedef struct {
Position pos;
int dir;
} Element;
Element stack[MAXROW * MAXCOL]; // 栈的最大容量
int top = -1; // 栈顶指针
// 入栈操作
void push(Position pos, int dir) {
top++;
stack[top].pos = pos;
stack[top].dir = dir;
}
// 出栈操作
void pop() {
top--;
}
// 判断栈是否为空
bool isEmpty() {
return top == -1;
}
// 判断当前位置是否为出口位置
bool isExit(Position pos) {
return pos.row == MAXROW - 1 && pos.col == MAXCOL - 1;
}
// 判断当前位置是否为通路
bool isPath(Position pos) {
if (pos.row < 0 || pos.row >= MAXROW || pos.col < 0 || pos.col >= MAXCOL) {
return false;
}
return maze[pos.row][pos.col] == 0;
}
// 查找迷宫所有路径
void findAllPaths(Position start) {
push(start, -1); // 将起点入栈,-1表示无方向可走
while (!isEmpty()) {
Element element = stack[top]; // 获取栈顶元素
Position pos = element.pos;
int dir = element.dir;
if (isExit(pos)) { // 到达终点,打印路径
printf("Path: ");
for (int i = 0; i <= top; i++) {
printf("(%d,%d) ", stack[i].pos.row, stack[i].pos.col);
}
printf("\n");
pop(); // 弹出栈顶元素,继续寻找其他路径
continue;
}
if (dir < 3) { // 方向控制变量dir表示当前位置能够继续走到的方向,0表示东,1表示南,2表示西,3表示北,-1表示无方向可走
Position nextPos;
switch (dir) {
case 0: // 向东
nextPos.row = pos.row;
nextPos.col = pos.col + 1;
break;
case 1: // 向南
nextPos.row = pos.row + 1;
nextPos.col = pos.col;
break;
case 2: // 向西
nextPos.row = pos.row;
nextPos.col = pos.col - 1;
break;
case 3: // 向北
nextPos.row = pos.row - 1;
nextPos.col = pos.col;
break;
}
if (isPath(nextPos)) { // 下一个位置为通路,入栈
push(nextPos, -1);
maze[nextPos.row][nextPos.col] = 1; // 标记为已访问
element.dir++; // 当前位置的方向控制变量加1
stack[top] = element; // 更新栈顶元素
continue;
}
}
pop(); // 当前位置无法继续走,弹出栈顶元素
}
}
// 查找迷宫最短路径
void findShortestPath(Position start) {
push(start, -1);
while (!isEmpty()) {
Element element = stack[top];
Position pos = element.pos;
int dir = element.dir;
if (isExit(pos)) {
printf("Shortest Path: ");
for (int i = 0; i <= top; i++) {
printf("(%d,%d) ", stack[i].pos.row, stack[i].pos.col);
}
printf("\n");
return;
}
if (dir < 3) {
Position nextPos;
switch (dir) {
case 0:
nextPos.row = pos.row;
nextPos.col = pos.col + 1;
break;
case 1:
nextPos.row = pos.row + 1;
nextPos.col = pos.col;
break;
case 2:
nextPos.row = pos.row;
nextPos.col = pos.col - 1;
break;
case 3:
nextPos.row = pos.row - 1;
nextPos.col = pos.col;
break;
}
if (isPath(nextPos)) {
push(nextPos, -1);
maze[nextPos.row][nextPos.col] = 1;
element.dir++;
stack[top] = element;
continue;
}
}
pop();
}
}
int main() {
Position start = {0, 0}; // 起点位置
findAllPaths(start); // 查找所有路径
printf("\n");
findShortestPath(start); // 查找最短路径
return 0;
}
```
上述程序中,我们使用了一个栈来保存每个已访问的位置及其能够继续走到的方向,从而实现了迷宫所有路径和最短路径的查找。在程序中,我们使用-1表示无方向可走,0表示向东,1表示向南,2表示向西,3表示向北。当一个位置的方向控制变量为3时,表示已经尝试了所有方向但是都无法继续走下去,此时需要弹出栈顶元素。
用C语言代码编写程序实现“栈求解迷宫”
在C语言中,我们可以使用栈数据结构来解决迷宫问题,因为栈的特性符合回溯搜索(Backtracking)的思想,通常用于遍历和寻找路径。下面是一个简单的迷宫求解算法的C语言代码示例,使用深度优先搜索(DFS):
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int row;
int col;
} Position;
// 定义迷宫数组,0表示墙,1表示可以通行的空格
int maze[5][5] = {
{1, 1, 1, 1, 0},
{0, 1, 0, 0, 1},
{1, 0, 1, 1, 1},
{1, 1, 0, 0, 0},
{0, 0, 0, 1, 1}
};
// 检查当前位置是否越界,是否是墙壁,或者已经访问过
int is_valid(int row, int col) {
return row >= 0 && row < 5 && col >= 0 && col < 5 && maze[row][col] == 1;
}
// 动态分配栈空间,存储位置信息
Position* create_position_stack() {
int stack_size = 0;
Position* stack = (Position*)malloc(sizeof(Position) * stack_size);
return stack;
}
// 将位置压入栈
void push_stack(Position* stack, int row, int col) {
stack[stack_size++] = (Position){row, col};
}
// 弹出栈顶元素并返回
Position pop_stack(Position* stack) {
if(stack_size > 0) {
Position temp = stack[stack_size - 1];
stack_size--;
return temp;
}
return (Position){-1, -1}; // 如果栈为空则返回无效位置
}
// 深度优先搜索,从起点开始
void dfs(Position start) {
Position stack[5]; // 假设最大步数不超过5x5迷宫
Position current = start;
while(1) {
push_stack(stack, current.row, current.col); // 入栈当前节点
if(current.row == 4 && current.col == 4) { // 到达终点
printf("Path found: %d %d -> %d %d\n",
start.row, start.col, current.row, current.col);
break;
}
// 四个相邻方向检查
for(int dir = 0; dir < 4; dir++) {
int newRow = current.row + directions[dir][0];
int newCol = current.col + directions[dir][1];
if(is_valid(newRow, newCol)) {
current.row = newRow;
current.col = newCol;
break;
}
}
if(stack_size > 0) { // 栈非空,取出上一步
current = pop_stack(stack);
} else { // 栈为空,无路可走
printf("No path found.\n");
return;
}
}
}
int main() {
dfs((Position){0, 0}); // 从起点(0,0)开始搜索
return 0;
}
阅读全文
相关推荐
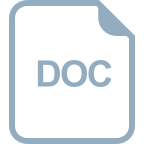
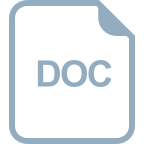
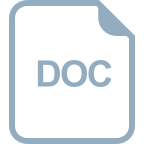
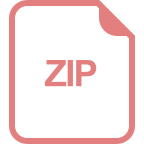
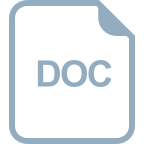


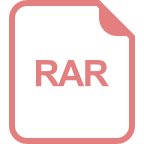
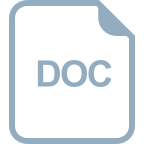
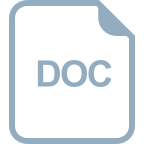




