提供下C代码的 SHA算法
时间: 2023-09-09 10:04:29 浏览: 175
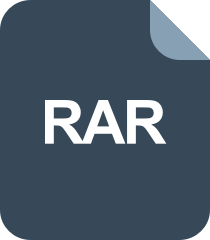
sha-1.rar_SHA_sha 明文_sha-1_sha源代码
### 回答1:
SHA(Secure Hash Algorithm)是一种数据加密算法,用于确保数据的完整性和安全性。在C语言中,可以使用 OpenSSL 库来实现 SHA 算法。
下面是一个使用 OpenSSL 库计算字符串的 SHA 哈希值的 C 程序示例:
```
#include <stdio.h>
#include <string.h>
#include <openssl/sha.h>
int main(int argc, char *argv[])
{
// 定义需要计算哈希值的字符串
char *str = "hello, world";
// 定义用于存储哈希值的数组
unsigned char hash[SHA_DIGEST_LENGTH];
// 计算字符串的 SHA 哈希值
SHA1(str, strlen(str), hash);
// 打印哈希值
for (int i = 0; i < SHA_DIGEST_LENGTH; i++)
printf("%02x", hash[i]);
printf("\n");
return 0;
}
```
在编译这段代码时,需要添加 `-lcrypto` 选项,例如:
```
gcc sha.c -o sha -lcrypto
```
运行后,程序会输出字符串 "hello, world" 的 SHA 哈希值。
注意:SHA 算法有多种版本,如 SHA1、SHA2 和 SHA3,其中 SHA1 是最常用的版本。上述代码使用的是 SHA1 算法。
### 回答2:
SHA(Secure Hash Algorithm,安全散列算法)是一种广泛使用的密码学哈希函数,常用于数据完整性验证、数字签名等安全应用中。以下是一个简化的C代码示例,用于计算SHA-256哈希值:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/sha.h>
void sha256(const char* str, char* output) {
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
SHA256_Update(&sha256, str, strlen(str));
SHA256_Final(hash, &sha256);
for (int i = 0; i < SHA256_DIGEST_LENGTH; i++) {
sprintf(output + (i * 2), "%02x", hash[i]);
}
output[64] = '\0'; // SHA-256哈希值长度为64,需手动添加终止符
}
int main() {
char input[] = "Hello, World!";
char output[65];
sha256(input, output);
printf("SHA-256哈希值:%s\n", output);
return 0;
}
```
以上示例使用了OpenSSL库中的SHA256函数来计算SHA-256哈希值。在使用时,可以调用`sha256`函数并传入要计算哈希值的字符串,结果会存储在`output`数组中。最后,我们打印出计算得到的SHA-256哈希值。
请注意,以上示例代码仅用于方便理解SHA-256算法的实现过程。在实际的应用中,应该使用经过严格验证和测试的可靠库或实现来计算SHA哈希值,以确保安全性和正确性。
### 回答3:
SHA算法是一种常用的密码散列函数。下面是一个用C语言实现的SHA-256算法的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <stdint.h>
#define ROTRIGHT(word, bits) (((word) >> (bits)) | ((word) << (32-(bits))))
#define CH(x, y, z) (((x) & (y)) ^ (~(x) & (z)))
#define MAJ(x, y, z) (((x) & (y)) ^ ((x) & (z)) ^ ((y) & (z)))
#define EP0(x) (ROTRIGHT(x, 2) ^ ROTRIGHT(x, 13) ^ ROTRIGHT(x, 22))
#define EP1(x) (ROTRIGHT(x, 6) ^ ROTRIGHT(x, 11) ^ ROTRIGHT(x, 25))
#define SIG0(x) (ROTRIGHT(x, 7) ^ ROTRIGHT(x, 18) ^ ((x) >> 3))
#define SIG1(x) (ROTRIGHT(x, 17) ^ ROTRIGHT(x, 19) ^ ((x) >> 10))
const uint32_t K[64] = {
0x428a2f98, 0x71374491, 0xb5c0fbcf, 0xe9b5dba5, 0x3956c25b, 0x59f111f1, 0x923f82a4, 0xab1c5ed5,
0xd807aa98, 0x12835b01, 0x243185be, 0x550c7dc3, 0x72be5d74, 0x80deb1fe, 0x9bdc06a7, 0xc19bf174,
0xe49b69c1, 0xefbe4786, 0x0fc19dc6, 0x240ca1cc, 0x2de92c6f, 0x4a7484aa, 0x5cb0a9dc, 0x76f988da,
0x983e5152, 0xa831c66d, 0xb00327c8, 0xbf597fc7, 0xc6e00bf3, 0xd5a79147, 0x06ca6351, 0x14292967,
0x27b70a85, 0x2e1b2138, 0x4d2c6dfc, 0x53380d13, 0x650a7354, 0x766a0abb, 0x81c2c92e, 0x92722c85,
0xa2bfe8a1, 0xa81a664b, 0xc24b8b70, 0xc76c51a3, 0xd192e819, 0xd6990624, 0xf40e3585, 0x106aa070,
0x19a4c116, 0x1e376c08, 0x2748774c, 0x34b0bcb5, 0x391c0cb3, 0x4ed8aa4a, 0x5b9cca4f, 0x682e6ff3,
0x748f82ee, 0x78a5636f, 0x84c87814, 0x8cc70208, 0x90befffa, 0xa4506ceb, 0xbef9a3f7, 0xc67178f2
};
void sha256(const uint8_t *message, size_t message_len, uint32_t hash[8]) {
uint32_t h[8] = {
0x6a09e667, 0xbb67ae85, 0x3c6ef372, 0xa54ff53a, 0x510e527f, 0x9b05688c, 0x1f83d9ab, 0x5be0cd19
};
size_t new_len = ((((message_len + 8) / 64) + 1) * 64) - 8;
uint8_t *new_message = calloc(new_len + 64, 1);
memcpy(new_message, message, message_len);
new_message[message_len] = 0x80;
uint32_t bits_len = message_len * 8;
memcpy(new_message + new_len, &bits_len, 4);
size_t chunk_count = new_len / 64;
for (size_t i = 0; i < chunk_count; i++) {
uint32_t w[64];
const uint8_t *chunk = new_message + (i * 64);
for (size_t j = 0; j < 16; j++) {
w[j] = (chunk[j*4] << 24) | (chunk[j*4+1] << 16) | (chunk[j*4+2] << 8) | chunk[j*4+3];
}
for (size_t j = 16; j < 64; j++) {
w[j] = SIG1(w[j-2]) + w[j-7] + SIG0(w[j-15]) + w[j-16];
}
uint32_t a = h[0];
uint32_t b = h[1];
uint32_t c = h[2];
uint32_t d = h[3];
uint32_t e = h[4];
uint32_t f = h[5];
uint32_t g = h[6];
uint32_t k = h[7];
for (size_t j = 0; j < 64; j++) {
uint32_t t1 = g + EP1(e) + CH(e, f, g) + K[j] + w[j];
uint32_t t2 = EP0(a) + MAJ(a, b, c);
g = f;
f = e;
e = d + t1;
d = c;
c = b;
b = a;
a = t1 + t2;
}
h[0] += a;
h[1] += b;
h[2] += c;
h[3] += d;
h[4] += e;
h[5] += f;
h[6] += g;
h[7] += k;
}
for (size_t i = 0; i < 8; i++) {
hash[i] = h[i];
}
free(new_message);
}
int main() {
const char *message = "Hello, world!";
uint32_t hash[8];
sha256((const uint8_t *)message, strlen(message), hash);
for (size_t i = 0; i < 8; i++) {
printf("%08x ", hash[i]);
}
printf("\n");
return 0;
}
```
以上是一个简单的SHA-256算法的C代码实现。它首先会将输入的消息进行填充和整理,然后将消息划分为多个512位的分块,针对每个分块进行一系列的运算,最终生成一个256位的哈希值。这里的示例代码只是对字符串"Hello, world!"进行SHA-256计算,并打印出最终结果。实际应用中,可以根据需要将此算法应用于具体的数据处理任务。
阅读全文
相关推荐
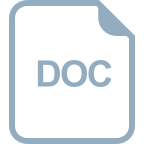
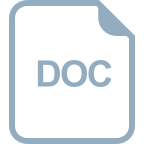
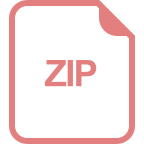

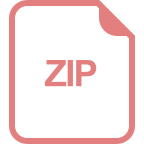
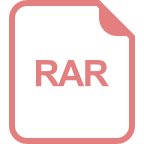
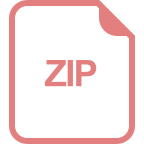
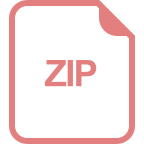
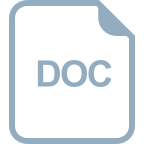





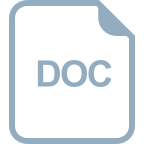
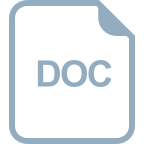