用java实现五子棋
时间: 2023-08-31 08:24:28 浏览: 151
### 回答1:
好的,这是一个五子棋的简单实现方式。
首先,我们需要准备一个二维数组来存储棋盘信息,0表示这个位置没有棋子,1表示黑棋,2表示白棋。
然后,我们可以使用一个变量来记录当前是哪一方在下棋,每次可以让用户输入棋子的位置,并在二维数组中放置棋子。
接着,我们需要检查每一方是否获胜,可以使用循环来检查每一行、每一列和每一条对角线是否有五个连续的棋子。
最后,我们可以使用双重循环来打印出棋盘的当前状态。
这是一个简单的实现方式,你可以按照这个思路来编写五子棋的代码。
示例代码:
```java
public class Gobang {
// 定义棋盘大小
private static final int BOARD_SIZE = 15;
// 定义一个二维数组来充当棋盘
private String[][] board;
// 定义当前是哪一方在下棋
private String currentPlayer;
public void initBoard() {
// 初始化棋盘数组
board = new String[BOARD_SIZE][BOARD_SIZE];
// 把棋盘数组全部赋为"+"
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = "+";
}
}
// 初始时,玩家1先手
currentPlayer
### 回答2:
五子棋是一种非常经典的策略性棋类游戏,在Java中实现五子棋可以通过面向对象的方式来完成。
首先,我们需要创建一个棋盘类Chessboard,用来表示五子棋的游戏场景。棋盘类中需要包含一个二维数组来表示棋盘上的棋子位置,以及相关的方法用于下子、判断胜负等操作。
接下来,我们需要创建一个棋子类Piece,用来表示棋盘上的每个棋子。棋子类中可以包含一个枚举类型的成员变量,表示棋子的类型(黑子或白子),以及其在棋盘中的位置。
然后,我们可以创建一个游戏类Game,用来控制整个游戏的流程。游戏类中需要有一个实例化棋盘类和棋子类的对象,并实现下子、判断胜负、打印棋盘等方法。
在游戏开始时,我们可以通过用户输入来决定先手还是后手。然后,程序会提示玩家输入下子的位置,将棋子放在对应的位置上。接着,程序会调用判断胜负的方法来判断当前局势是否已经分出胜负。如果胜负已分,则游戏结束;否则,双方继续轮流下子,直到出现胜负或者棋盘已满。
最后,我们可以在游戏类中实现一个打印棋盘的方法,将当前棋盘的状态以图形化的方式展示出来,以方便玩家观察和下子。
总的来说,用Java实现五子棋的过程就是通过面向对象的方式创建棋盘类、棋子类和游戏类,并实现相应的方法来控制游戏的流程和判断胜负。这样,我们就可以用Java来实现一个简单的五子棋游戏了。
### 回答3:
使用Java实现五子棋可以分为以下几个步骤。
1. 创建游戏界面:可以使用Java Swing或者JavaFX创建一个窗口,设置好棋盘大小和线条,以及棋子的大小和颜色等等。
2. 实现游戏逻辑:定义一个二维数组来表示棋盘,每个数组元素用于表示一个格子的状态,比如空白、黑子或白子。通过鼠标事件监听器来获取玩家下棋的位置,并将棋子的状态更新到数组中。
3. 判断胜负条件:每次有玩家下棋后,需要判断是否有五连珠的情况出现,可以通过遍历棋盘数组来检查每个格子的横向、纵向、斜向是否有五颗相同的棋子。
4. 实现人机对战:可以通过编写简单的AI算法来实现人机对战,比如使用最简单的随机算法来让电脑下棋。
5. 添加游戏结束的提示:当有玩家或者电脑获胜时,弹出相应的对话框来提示游戏结束。
6. 完善其他功能:可以添加悔棋、重新开始游戏等功能,使游戏更加友好。
使用Java来实现五子棋可以使用面向对象的思想,将棋盘、棋子、玩家等抽象成类,便于维护和扩展。同时,可以使用图形界面来提升用户的交互体验,增加游戏的可玩性。
阅读全文
相关推荐
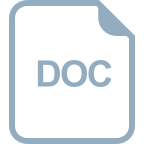
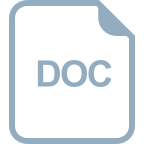
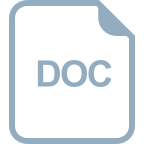
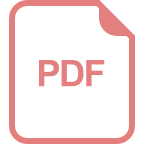
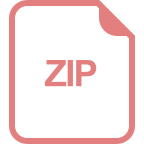
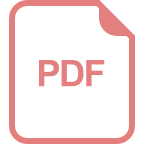

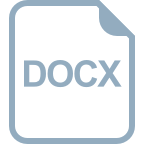
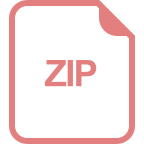
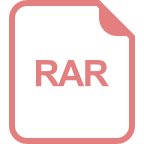
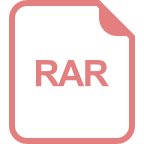
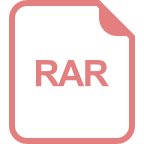
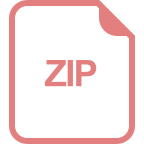


