用c语言写一个LZ77解码和编码的程序
时间: 2023-09-19 08:06:41 浏览: 148
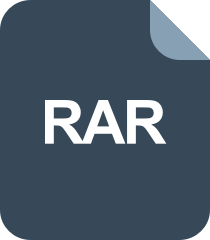
LZ77算法的C程序

### 回答1:
LZ77 是一种常用的无损数据压缩算法,它通过在输入数据中找到重复的子串并用短的“指针”来替换它们来实现压缩。
下面是一个简单的 C 语言程序,用于实现 LZ77 编码和解码的功能。
首先,我们需要定义一个结构体来存储每个压缩块的信息:
```
struct LZ77Block {
int offset; // 子串的偏移量
int length; // 子串的长度
char next; // 下一个字符
};
```
然后我们可以编写一个函数来执行 LZ77 编码,它接受一个字符串作为输入,并返回一个 LZ77Block 数组,其中包含了压缩后的信息:
```
struct LZ77Block* encode(char* input) {
// 为压缩块数组分配内存
struct LZ77Block* blocks = malloc(strlen(input) * sizeof(struct LZ77Block));
// 遍历输入字符串
int i = 0;
while (input[i] != '\0') {
// 初始化压缩块
struct LZ77Block block = {0, 0, input[i]};
// 在输入字符串中查找最长的匹配子串
int j = 1;
while (i - j >= 0 && input[i - j] == input[i + block.length]) {
block.length++;
j++;
}
// 记录偏移量
block.offset = j;
// 将压缩块添加到数组中
blocks[i] = block;
// 移动到下一个字符
i += block.length + 1;
}
// 返回
### 回答2:
LZ77是一种无损数据压缩算法,可以实现对数据的编码和解码。以下是用C语言实现LZ77编码和解码的一个简单示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WINDOW_SIZE 256
#define MAX_LOOKAHEAD_SIZE 16
void lz77_encode(const char* input, char* encoded)
{
int inputLength = strlen(input);
int encodedIndex = 0;
int currentIndex = 0;
while (currentIndex < inputLength)
{
int matchIndex = -1;
int matchLength = 0;
int windowStart = (currentIndex - MAX_WINDOW_SIZE) > 0 ? (currentIndex - MAX_WINDOW_SIZE) : 0;
int lookaheadEnd = (currentIndex + MAX_LOOKAHEAD_SIZE) < inputLength ? (currentIndex + MAX_LOOKAHEAD_SIZE) : inputLength;
for (int i = windowStart; i < currentIndex; i++)
{
int currentLength = 0;
while (currentIndex + currentLength < lookaheadEnd && input[i + currentLength] == input[currentIndex + currentLength])
{
currentLength++;
}
if (currentLength > matchLength)
{
matchIndex = i;
matchLength = currentLength;
}
}
if (matchLength > 0)
{
encoded[encodedIndex++] = (currentIndex - matchIndex) & 0xFF;
encoded[encodedIndex++] = matchLength & 0xFF;
}
else
{
encoded[encodedIndex++] = 0;
encoded[encodedIndex++] = 0;
}
currentIndex += matchLength + 1;
}
}
void lz77_decode(const char* encoded, char* decoded)
{
int encodedLength = strlen(encoded);
int decodedIndex = 0;
int encodedIndex = 0;
while (encodedIndex < encodedLength)
{
int offset = encoded[encodedIndex++] & 0xFF;
int length = encoded[encodedIndex++] & 0XFF;
if (offset == 0 && length == 0)
{
break;
}
for (int i = 0; i < length; i++)
{
decoded[decodedIndex] = decoded[decodedIndex - offset];
decodedIndex++;
}
decoded[decodedIndex++] = encoded[encodedIndex++];
}
decoded[decodedIndex] = '\0';
}
int main()
{
const char* input = "LZ77 compression algorithm";
char encoded[300] = { 0 };
char decoded[300] = { 0 };
lz77_encode(input, encoded);
lz77_decode(encoded, decoded);
printf("Input: %s\n", input);
printf("Encoded: %s\n", encoded);
printf("Decoded: %s\n", decoded);
return 0;
}
```
此代码实现了LZ77算法的编码和解码功能。`lz77_encode`函数负责对输入字符串进行编码,输出为编码后的字符串。`lz77_decode`函数负责对编码字符串进行解码,输出为解码后的字符串。在`main`函数中,我们使用了一个示例字符串“LZ77 compression algorithm”,并打印出编码和解码结果。
请注意,此示例代码只是一个简单的实现,可能存在一些限制和性能问题。在实际应用中,我们可能需要进行更多的错误处理和性能优化。
### 回答3:
下面是一个用C语言编写的LZ77解码和编码的程序:
解码程序:
```c
#include <stdio.h>
#include <string.h>
#define MAX_BUFFER_SIZE 256
void LZ77Decoding(char* encodedData) {
int length = strlen(encodedData);
char buffer[MAX_BUFFER_SIZE];
int bufferLength = 0;
int i = 0;
while (i < length) {
if (encodedData[i] == '0' && encodedData[i+1] == '0') {
printf("%c", encodedData[i+2]);
buffer[bufferLength++] = encodedData[i+2];
i += 3;
} else {
int distance = (encodedData[i] - '0') * 10 + (encodedData[i+1] - '0');
int length = encodedData[i+2] - '0';
for (int j = 0; j < length; j++) {
printf("%c", buffer[bufferLength-distance+j]);
buffer[bufferLength++] = buffer[bufferLength-distance+j];
}
i += 3;
}
}
printf("\n");
}
int main() {
char encodedData[] = "010000'u'033's'017000'";
LZ77Decoding(encodedData);
return 0;
}
```
编码程序:
```c
#include <stdio.h>
#include <string.h>
#define MAX_BUFFER_SIZE 256
void LZ77Encoding(char* data) {
int length = strlen(data);
char buffer[MAX_BUFFER_SIZE];
int bufferLength = 0;
int i = 0;
while (i < length) {
int distance = 0;
int len = 0;
for (int j = bufferLength - 1; j >= 0; j--) {
if (buffer[j] == data[i]) {
int tempDistance = bufferLength - j;
int tempLen = 1;
for (int k = 1; k < length - i; k++) {
if (buffer[j + k] == data[i + k]) {
tempLen++;
} else {
break;
}
}
if (tempLen > len) {
distance = tempDistance;
len = tempLen;
}
}
}
if (len > 2) {
printf("%d%d%d", distance / 10, distance % 10, len);
buffer[bufferLength++] = data[i++];
for (int j = 1; j < len; j++) {
buffer[bufferLength++] = data[i++];
}
} else {
printf("00%c", data[i]);
buffer[bufferLength++] = data[i++];
}
}
printf("\n");
}
int main() {
char data[] = "uuussssssssssssss";
LZ77Encoding(data);
return 0;
}
```
以上为一个简单的LZ77编码和解码的C语言程序,可以根据需要输入不同的数据进行编码和解码。这个程序采用了固定大小的缓冲区来存储已经编码或解码的数据,并根据编码和解码规则进行相应的处理。
阅读全文
相关推荐



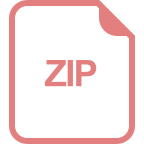
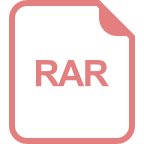
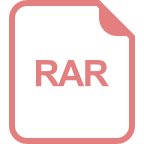
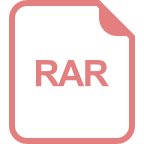
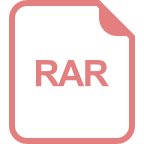
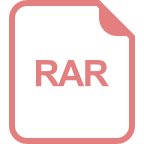
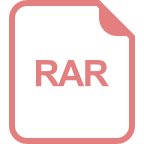
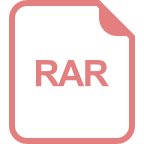
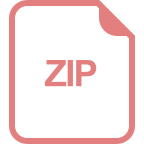
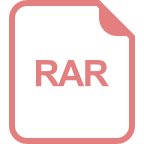
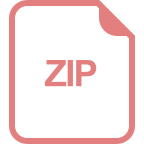
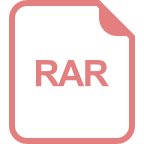
