lz77压缩算法 c语言
时间: 2023-11-22 21:02:52 浏览: 120
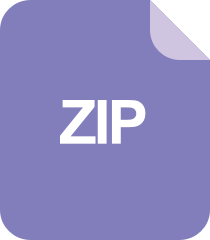
lz77压缩算法c语言实现
LZ77压缩算法是一种用于数据压缩的算法,它可以在一定程度上减小数据的存储空间。该算法以C语言实现时,通常需要使用两个主要的数据结构:窗口和缓冲区。窗口是用于存储已经编码的数据的缓存区域,而缓冲区则是用于暂存待编码的数据。
在LZ77算法中,编码过程主要分为两个步骤:查找和匹配。查找步骤是通过在窗口中寻找与缓冲区中待编码数据匹配的最长字符串,然后记录该字符串在窗口中的位置和长度。匹配步骤是将查找到的匹配字符串的位置和长度编码,并将下一个待编码的字符串加入到窗口中,重复以上步骤直至所有数据编码完毕。
解码过程则是通过根据编码得到的位置和长度信息,在窗口中找到对应的字符串,然后将其输出到解压缩后的数据中,最终得到原始的数据。
在C语言中实现LZ77压缩算法时,需要注意对于窗口和缓冲区的管理,以及正确地实现查找和匹配等算法逻辑。同时,还需要处理一些边界情况和特殊数据,以确保算法的正确性和稳定性。通过合理优化算法实现,并结合一些高效的数据结构和编程技巧,可以更好地实现LZ77压缩算法的性能和效果。
阅读全文
相关推荐
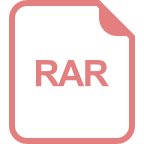
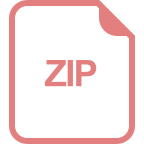














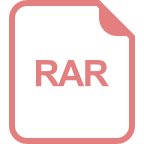