pandas获取某个值
时间: 2023-08-03 18:05:44 浏览: 41
可以使用 `loc` 或 `iloc` 方法来获取 DataFrame 中的某个值。其中,`loc` 方法是基于标签的索引,而 `iloc` 方法是基于位置的索引。例如,假设有以下 DataFrame:
```python
import pandas as pd
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'gender': ['F', 'M', 'M']})
```
我们可以使用以下方法获取某个值:
- 使用标签索引:使用 `loc` 方法,并指定行标签和列标签。例如,获取第一行第二列的元素:
```python
df.loc[0, 'age']
```
- 使用位置索引:使用 `iloc` 方法,并指定行索引和列索引。例如,获取第二行第三列的元素:
```python
df.iloc[1, 2]
```
注意,标签索引和位置索引都是从 0 开始的。
相关问题
pandas获取 某个值
在 pandas 中获取某个值可以使用 loc 或 iloc 函数。
使用 loc 函数获取某个值的语法为:
```
df.loc[row_label, column_label]
```
其中,row_label 表示行标签,column_label 表示列标签。例如:
```
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 32, 18, 47],
'gender': ['F', 'M', 'M', 'M']}
df = pd.DataFrame(data)
print(df.loc[1, 'name'])
```
输出结果为:
```
Bob
```
使用 iloc 函数获取某个值的语法为:
```
df.iloc[row_index, column_index]
```
其中,row_index 表示行索引,column_index 表示列索引。例如:
```
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 32, 18, 47],
'gender': ['F', 'M', 'M', 'M']}
df = pd.DataFrame(data)
print(df.iloc[1, 0])
```
输出结果为:
```
Bob
```
pandas获取值的索引
您好!感谢您的提问。
如果您要获取某个值在 DataFrame 或 Series 中的索引,可以使用 `loc` 属性或 `index` 属性。具体操作如下:
```python
import pandas as pd
# 创建一个 Series
s = pd.Series([1, 2, 3, 4], index=['a', 'b', 'c', 'd'])
# 使用 loc 属性获取值的索引
print(s.loc[s == 3].index[0]) # 输出 'c'
# 获取 DataFrame 中某一列的值的索引
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df['B'].loc[df['B'] == 5].index[0]) # 输出 1
```
在以上代码中,`loc` 属性用于定位符合条件的数据,然后使用 `index` 属性获取该数据的索引。对于 DataFrame,需要先使用列名定位到某一列,然后再使用 `loc` 属性和 `index` 属性获取索引。
希望这个回答能够帮到您,如果您还有任何问题,请随时问我。
相关推荐
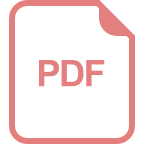












