1. 设计汽车类,实现如下要求: (1) 在该类中定义汽车品牌、价格和颜色等私有成员变量,并对成员变量定义set和get方法; (2) 定义汽车类3个构造方法,分别为不带参数的构造方法、带3个参数实现初始化其成员变量的构造方法、带一个参数实现初始化其品牌的构造方法; (3) 定义汽车类printInfo()方法实现输出汽车基本信息; (4) 定义汽车类的3个对象,分别以“红旗”“五菱宏光”“蔚来”作为汽车品牌,每一个对象调用不同构造方法,实现调用其成员方法。
时间: 2023-02-09 17:46:34 浏览: 291
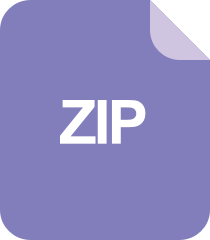
可以用bmp文件构造该类,不须在设计时将bmp文件加入到资源中去。
以下是使用 Java 语言实现汽车类的代码示例:
```
class Car {
// 定义私有成员变量
private String brand;
private double price;
private String color;
// 定义 set 方法
public void setBrand(String brand) {
this.brand = brand;
}
public void setPrice(double price) {
this.price = price;
}
public void setColor(String color) {
this.color = color;
}
// 定义 get 方法
public String getBrand() {
return this.brand;
}
public double getPrice() {
return this.price;
}
public String getColor() {
return this.color;
}
// 定义不带参数的构造方法
public Car() {
// 默认初始化成员变量
this.brand = "";
this.price = 0.0;
this.color = "";
}
// 定义带 3 个参数的构造方法
public Car(String brand, double price, String color) {
this.brand = brand;
this.price = price;
this.color = color;
}
// 定义带 1 个参数的构造方法
public Car(String brand) {
this.brand = brand;
this.price = 0.0;
this.color = "";
}
// 定义 printInfo 方法
public void printInfo() {
System.out.println("汽车品牌:" + this.brand);
System.out.println("汽车价格:" + this.price);
System.out.println("汽车颜色:" + this.color);
}
}
// 创建 3 个 Car 对象
Car car1 = new Car();
Car car2 = new Car("五菱宏光", 50000, "红色");
Car car3 = new Car("蔚来");
// 调用 Car 类的 printInfo 方法
car1.printInfo();
car2.printInfo();
car3.printInfo();
```
上面的代码定义了一个 Car 类,其中包含了汽车品牌、价格和颜色等私有成员变量。它还定义了
阅读全文
相关推荐
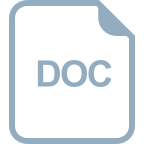
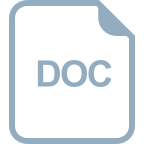
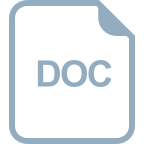
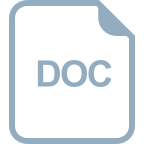
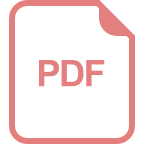
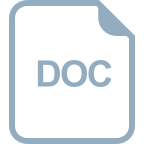
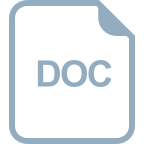
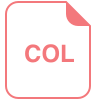
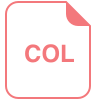
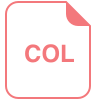
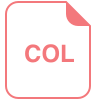
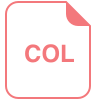
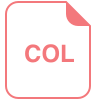
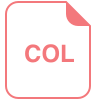
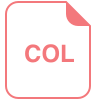
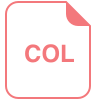