根据Excel表中提供的网址信息,打开对应的网页,抽取标题和文本内容,并存储到TXT中,python代码示例
时间: 2023-09-16 09:05:27 浏览: 170
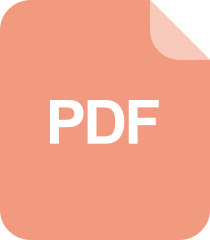
用Python程序抓取网页的HTML信息的一个小实例
### 回答1:
这是一个使用 Python 实现的示例代码:
```
import openpyxl
import requests
from bs4 import BeautifulSoup
# 读取 Excel 表中的网址
wb = openpyxl.load_workbook('urls.xlsx')
sheet = wb['Sheet1']
urls = sheet['A']
# 抓取网页信息
for url in urls:
response = requests.get(url.value)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
content = soup.get_text()
# 存储到 TXT 中
with open('content.txt', 'w', encoding='utf-8') as f:
f.write(title + '\n\n' + content)
```
在这段代码中,我们使用了 openpyxl 模块来读取 Excel 表中的网址信息,使用 requests 模块来访问网页,使用 BeautifulSoup 模块来提取网页的标题和文本内容,最后使用 Python 的文件操作来存储到 TXT 中。
注意:在运行这段代码之前,需要确保已经安装了 openpyxl、requests 和 BeautifulSoup 这三个 Python 模块。
### 回答2:
Python代码示例:
```python
import requests
from bs4 import BeautifulSoup
# 读取Excel表格中的网址信息,可以使用pandas库
# url_list = pd.read_excel('文件路径')
# 遍历网址列表
for url in url_list:
try:
# 使用requests库发送GET请求,获取网页内容
response = requests.get(url)
response.encoding = 'utf-8' # 设置编码方式
# 使用BeautifulSoup库解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 提取标题文本
title = soup.title.string
# 提取文本内容
content = ''
for p in soup.find_all('p'):
content += p.text + '\n'
# 存储到TXT文件
with open('result.txt', 'a', encoding='utf-8') as f:
f.write('标题:' + title + '\n')
f.write('内容:' + content + '\n\n')
except Exception as e:
print('抓取网页出现异常:', e)
```
注意事项:
1. 上述代码需要引入`requests`库和`BeautifulSoup`库。可以使用pip命令安装这两个库。
2. 需要将Excel文件中的网址信息读取到`url_list`变量中,可以使用pandas库的`read_excel`方法实现。
3. 根据网页的具体结构,使用合适的方法获取标题和文本内容。上述示例中使用的是BeautifulSoup库的方法,在解析时会根据标签类型提取相应的内容,可以根据实际情况进行调整。
4. 最终结果会存储到result.txt文件中,代码示例中每次写入结果时都以追加模式打开文件,如果需要覆盖文件内容,可以使用写模式打开。
### 回答3:
import requests
from bs4 import BeautifulSoup
def get_page_text(url):
# 发送请求获取网页内容
response = requests.get(url)
response.encoding = response.apparent_encoding
html = response.text
# 解析HTML并提取标题和文本内容
soup = BeautifulSoup(html, 'html.parser')
title = soup.title.string.strip()
content = soup.get_text().strip()
# 返回标题和内容
return title, content
def save_to_txt(title, content):
# 打开文件,如果文件不存在会自动创建
with open('result.txt', 'a', encoding='utf-8') as f:
# 写入标题和内容
f.write(f'Title: {title}\n')
f.write(f'Content: {content}\n\n')
if __name__ == '__main__':
# 读取Excel表,并提取网址信息
with open('excel.csv', 'r', encoding='utf-8') as f:
lines = f.readlines()
urls = [line.strip().split(',')[1] for line in lines[1:]]
# 遍历网址列表,处理每个网页
for url in urls:
try:
title, content = get_page_text(url)
save_to_txt(title, content)
except Exception as e:
print(f'Error occurred when extracting data from "{url}": {e}')
阅读全文
相关推荐
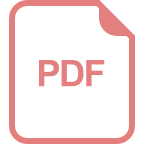
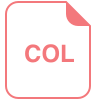
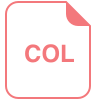
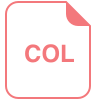
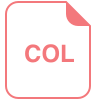
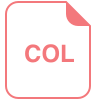
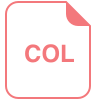
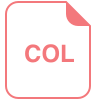
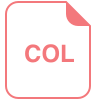
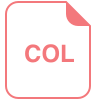
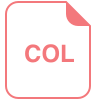
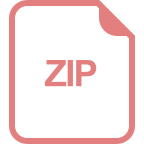