vue实现完整雪花特效代码
时间: 2023-09-09 12:04:25 浏览: 130
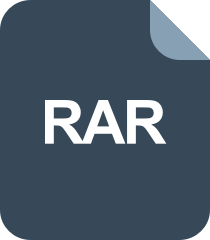
雪花效果源码
### 回答1:
这是一个可以在 Vue.js 中实现完整雪花特效的代码示例:
```
<template>
<div id="app">
<div class="snowflakes" v-bind:style="snowflakesStyle">
<span v-for="i in snowflakesCount" v-bind:key="i" class="snowflake"></span>
</div>
</div>
</template>
<style>
.snowflake {
position: absolute;
top: -50px;
left: -50px;
width: 50px;
height: 50px;
background: white;
border-radius: 50%;
box-shadow: 0 0 10px white;
animation: falling 10s linear infinite;
}
@keyframes falling {
from {
transform: rotate(0deg) translateY(-50px);
}
to {
transform: rotate(360deg) translateY(100vh);
}
}
</style>
<script>
export default {
name: "App",
data() {
return {
snowflakesCount: 100,
snowflakesStyle: {
width: "100vw",
height: "100vh",
position: "fixed",
top: 0,
left: 0,
overflow: "hidden"
}
};
}
};
</script>
```
在这段代码中,我们使用了 Vue.js 的 `v-for` 指令来循环渲染 `snowflake` 组件,并为每个雪花设置了绝对定位,使用了 CSS 动画来让雪花旋转并下落。
希望这个示例能帮助你。
### 回答2:
以下是一个用Vue实现完整雪花特效的代码示例:
``` html
<template>
<div>
<canvas ref="canvas" :width="width" :height="height"></canvas>
</div>
</template>
<script>
export default {
data() {
return {
width: 800,
height: 600,
ctx: null,
flakes: [],
numFlakes: 100,
};
},
mounted() {
this.initCanvas();
this.generateFlakes();
this.animate();
},
methods: {
initCanvas() {
this.ctx = this.$refs.canvas.getContext('2d');
},
generateFlakes() {
for (let i = 0; i < this.numFlakes; i++) {
const x = Math.random() * this.width;
const y = Math.random() * this.height;
const size = Math.random() * 3 + 2;
const speed = Math.random() * 1 + 0.5;
const opacity = Math.random() * 0.5 + 0.3;
const flake = { x, y, size, speed, opacity };
this.flakes.push(flake);
}
},
animate() {
requestAnimationFrame(this.animate);
this.ctx.clearRect(0, 0, this.width, this.height);
for (let i = 0; i < this.numFlakes; i++) {
const flake = this.flakes[i];
flake.y += flake.speed;
if (flake.y > this.height) {
flake.y = 0 - flake.size;
}
this.ctx.beginPath();
this.ctx.arc(flake.x, flake.y, flake.size, 0, Math.PI * 2);
this.ctx.closePath();
this.ctx.fillStyle = `rgba(255, 255, 255, ${flake.opacity})`;
this.ctx.fill();
}
},
},
};
</script>
```
上述代码实现了一个简单的雪花特效。首先,我们在模板中通过canvas元素创建了一个画布。在`mounted`生命周期钩子函数中,我们初始化了画布并生成了一定数量的雪花。然后,在`animate`方法中,我们使用循环遍历所有的雪花并更新它们的位置,根据需要循环播放雪花下落的动画效果。最后,我们使用`requestAnimationFrame`方法递归地调用`animate`方法,以实现平滑的动画效果。
这段代码的关键是使用`requestAnimationFrame`方法不断地重绘画布以更新雪花的位置和透明度。在循环中,我们使用`arc`方法绘制雪花的圆形,并使用`fillStyle`属性设置雪花的颜色和透明度,最后调用`fill`方法将雪花渲染到画布上。
你可以将以上代码复制到Vue项目中的组件中使用,然后引入这个组件并将其放置到你的网页中即可看到雪花特效的效果。
### 回答3:
Vue实现完整雪花特效的代码如下:
```vue
<template>
<div id="snowflakes">
<img
v-for="(flake, index) in snowflakes"
:key="index"
class="snowflake"
:src="flake.url"
:style="flake.style"
/>
</div>
</template>
<script>
export default {
data() {
return {
snowflakes: []
};
},
methods: {
createSnowflake() {
const screenWidth = document.documentElement.clientWidth;
const screenHeight = document.documentElement.clientHeight;
const flakeSize = this.getRandomSize();
const flakeSpeed = this.getRandomSpeed();
return {
url: 'snowflake.png', // 雪花图片的路径
style: {
left: `${this.getRandomPosition(screenWidth)}px`,
top: '-50px',
width: `${flakeSize}px`,
height: `${flakeSize}px`,
animation: `fall ${flakeSpeed}s linear infinite`
}
};
},
getRandomPosition(max) {
return Math.floor(Math.random() * max);
},
getRandomSize() {
return Math.floor(Math.random() * 20) + 10;
},
getRandomSpeed() {
return Math.floor(Math.random() * 5) + 3;
},
startSnowfall() {
setInterval(() => {
this.snowflakes.push(this.createSnowflake());
}, 500);
}
},
mounted() {
this.startSnowfall();
}
};
</script>
<style>
#snowflakes {
position: fixed;
top: 0;
left: 0;
z-index: -1;
pointer-events: none;
}
.snowflake {
position: absolute;
}
@keyframes fall {
0% {
transform: translateY(-50px) rotate(0deg);
}
100% {
transform: translateY(100vh) rotate(360deg);
}
}
</style>
```
上面的代码实现了一个简单的雪花特效。首先,在`mounted`生命周期钩子中调用`startSnowfall`方法来开始雪花的飘落。`startSnowfall`方法使用`setInterval`函数来定时添加雪花对象到`snowflakes`数组中,每500毫秒添加一个。
在`createSnowflake`方法中,根据屏幕的宽度和高度计算雪花的位置、尺寸和速度。生成的每个雪花对象都有一个`url`属性,以指定雪花图片的路径,并使用动态绑定的方式将雪花对象的样式应用到`img`标签上。
在样式中,为`img`标签设置`position: absolute`,使其脱离文档流,并在`#snowflakes`容器内创建一个层级低于其他元素的效果。使用`@keyframes`和`animation`属性来定义雪花下落的动画效果,其中`fall`为动画的名称,`transform`属性为雪花的位移和旋转效果。
每个雪花对象都会根据其定义的动画属性在页面中飘落。
阅读全文
相关推荐
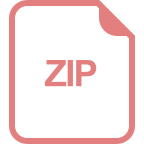


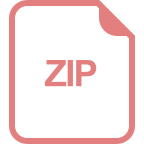
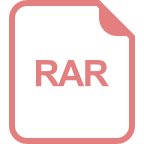
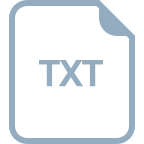
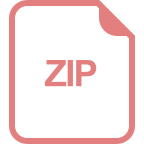
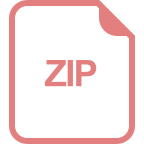
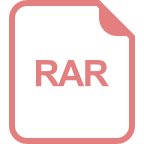
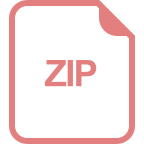
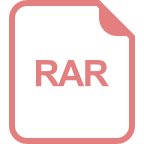
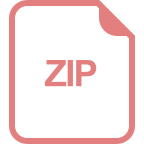
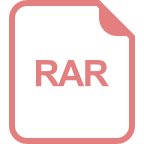
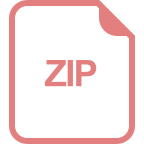
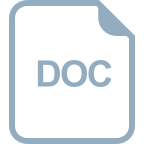
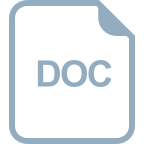
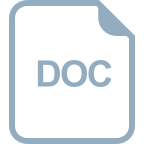