c语言完成,直接写代码,不用解释:编写单向线性链表程序用于连接多个字符型数据,以#作为结束输入的标志,例如ABCD。(1)根据题意定义合适的结构 体;(2)写出建立一个单项链表的函数;(3)写出输出链表中各节点值的函数
时间: 2023-02-06 14:37:41 浏览: 146
下面的方式进行链接:
定义链表结构体:
```
struct Node {
char data[100];
struct Node* next;
};
```
创建链表:
```
struct Node* createLinkedList(char** data, int num) {
if (num <= 0) return NULL;
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
strcpy(head->data, data[0]);
head->next = NULL;
struct Node* cur = head;
for (int i = 1; i < num; i++) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
strcpy(newNode->data, data[i]);
newNode->next = NULL;
cur->next = newNode;
cur = newNode;
}
return head;
}
```
在链表末尾插入新结点:
```
void insertNode(struct Node* head, char* data) {
struct Node* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
strcpy(newNode->data, data);
newNode->next = NULL;
cur->next = newNode;
}
```
在链表中间插入新结点:
```
void insertNodeAfter(struct Node* node, char* data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
strcpy(newNode->data, data);
newNode->next = node->next;
node->next = newNode;
}
```
删除链表中的结点:
```
void deleteNode(struct Node* head, char* data) {
struct Node* cur = head;
struct Node* prev = NULL;
while (cur != NULL) {
if (strcmp(cur->data, data) == 0) {
if (prev == NULL) {
head = cur->next;
} else {
prev->next = cur->next;
}
free(cur);
return;
}
prev = cur;
cur = cur->next;
}
}
```
遍历链表:
```
void traverseLinkedList(struct Node* head) {
struct Node* cur = head;
while (cur != NULL) {
printf("%s ", cur->data);
cur = cur->next;
}
printf("\n");
}
```
销毁链表:
```
void destroyLinkedList
相关推荐
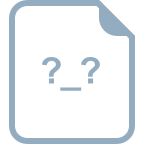
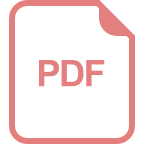















