c语言完成,直接写代码,不用解释:编写单向线性链表程序用于连接多个字符型数据,以当用户输入‘#’作为结束输入的标志,例如A B C D #输入结束。(1)根据题意定义合适的结构 体;(2)写出建立一个单项链表的函数;(3)写出输出链表中各节点值的函数
时间: 2023-08-31 19:24:32 浏览: 57
### 回答1:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体表示单向线性链表的节点
struct node {
char data[10]; // 存储字符型数据
struct node *next; // 指向下一个节点的指针
};
// 定义一个头节点,方便操作
struct node *head = NULL;
// 插入节点
void insert(char data[10]) {
struct node *new_node = (struct node*) malloc(sizeof(struct node)); // 创建新节点
strcpy(new_node->data, data); // 将字符串存入新节点的数据域
new_node->next = NULL; // 将新节点的指针赋为空
if (head == NULL) { // 如果链表为空
head = new_node; // 将头节点指向新节点
} else { // 如果链表不为空
struct node *temp = head; // 创建一个临时指针变量,指向头节点
while (temp->next != NULL) { // 循环找到链表的末尾
temp = temp->next; // 将临时指针变量指向下一个节点
}
temp->next = new_node; // 将末尾节点的指针指向新节点
}
}
// 删除节点
void delete(char data[10]) {
struct node *temp = head; // 创建一个临时指针变量,指向头节点
if (strcmp(temp->data, data) == 0) { // 如果头节点的数据与要删除的数据相同
head = temp->next; // 将头节点指向下一个节点
free(temp); // 释放节点空间
return;
}
while
### 回答2:
```c
#include<stdio.h>
#include<stdlib.h>
//定义链表结构体
struct Node{
char data;
struct Node* next;
};
//函数声明
void insert(struct Node** head_ref, char new_data);
void display(struct Node* node);
//主函数
int main(){
struct Node* head = NULL;
char input;
//获取用户输入
printf("请输入字符型数据,输入'0'结束输入:\n");
do{
scanf(" %c", &input);
if(input != '0'){
insert(&head, input);
}
}while(input != '0');
//显示链表内容
printf("链表内容为:");
display(head);
return 0;
}
//在链表末尾插入新节点
void insert(struct Node** head_ref, char new_data){
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
struct Node *last = *head_ref;
new_node->data = new_data;
new_node->next = NULL;
if (*head_ref == NULL) {
*head_ref = new_node;
return;
}
while (last->next != NULL)
last = last->next;
last->next = new_node;
return;
}
//显示链表内容
void display(struct Node* node){
while (node != NULL) {
printf("%c ", node->data);
node = node->next;
}
printf("\n");
}
```
使用该代码,用户可以输入多个字符型数据,直至输入'0'为止。代码会将这些字符型数据连接成一个单向线性链表,并显示链表的内容。
### 回答3:
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
char data; // 数据域
struct Node* next; // 指针域,指向下一个节点
} Node;
// 创建节点
Node* createNode(char data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 连接多个字符型数据到单向线性链表中
Node* connect(char* str) {
Node *head = NULL, *curr = NULL;
// 遍历字符数组
for (int i = 0; str[i] != '\0'; i++) {
if (head == NULL) {
// 如果链表为空,创建头节点
head = createNode(str[i]);
curr = head;
} else {
// 否则创建新的节点,并将其连接到链表的末尾
curr->next = createNode(str[i]);
curr = curr->next;
}
}
return head; // 返回链表的头节点
}
// 打印链表
void printList(Node* head) {
Node* curr = head;
while (curr != NULL) {
printf("%c ", curr->data);
curr = curr->next;
}
printf("\n");
}
int main() {
char str[100];
printf("请输入字符型数据:");
scanf("%s", str);
Node* head = connect(str); // 连接字符型数据到链表中
printList(head); // 打印链表
return 0;
}
相关推荐
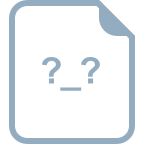
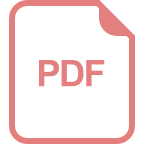















