c语言完成,直接写代码,不用解释:编写程序完成BMP图像(真彩色、256色)反色处理等功能。
时间: 2023-09-03 21:27:38 浏览: 132
### 回答1:
BMP图像的真彩色反色处理的代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#pragma pack(1)
typedef struct {
unsigned short bfType;
unsigned int bfSize;
unsigned short bfReserved1;
unsigned short bfReserved2;
unsigned int bfOffBits;
} BmpFileHeader;
typedef struct {
unsigned int biSize;
int biWidth;
int biHeight;
unsigned short biPlanes;
unsigned short biBitCount;
unsigned int biCompression;
unsigned int biSizeImage;
int biXPelsPerMeter;
int biYPelsPerMeter;
unsigned int biClrUsed;
unsigned int biClrImportant;
} BmpInfoHeader;
typedef struct {
unsigned char blue;
unsigned char green;
unsigned char red;
} RgbTriple;
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <input file> <output file>\n", argv[0]);
return 1;
}
FILE *fin = fopen(argv[1], "rb");
if (fin == NULL) {
printf("Error: failed to open input file.\n");
return 1;
}
BmpFileHeader fileHeader;
BmpInfoHeader infoHeader;
fread(&fileHeader, sizeof(BmpFileHeader), 1, fin);
fread(&infoHeader, sizeof(BmpInfoHeader), 1, fin);
if (fileHeader.bfType != 0x4d42) {
printf("Error: input file is not a BMP file.\n");
fclose(fin);
return 1;
}
if (infoHeader.biBitCount != 24) {
printf("Error: input file is not a true color BMP file.\n");
fclose(fin);
return 1;
}
int rowSize = (infoHeader.biWidth * infoHeader.biBitCount + 31) / 32 * 4;
int padding = rowSize - infoHeader.biWidth * infoHeader.biBitCount / 8;
int imageSize = rowSize * abs(infoHeader.biHeight);
RgbTriple *imageData = (RgbTriple *)malloc(imageSize);
if (imageData == NULL) {
printf("Error: failed to allocate memory.\n");
fclose(fin);
return 1;
}
fseek(fin, fileHeader.bfOffBits, SEEK_SET);
fread(imageData, imageSize, 1, fin);
fclose(fin);
for (int i = 0; i < imageSize; i += 3) {
imageData[i / 3].blue = 255 - imageData[i / 3].blue;
imageData[i / 3].green = 255 - imageData[i / 3].green;
### 回答2:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
unsigned char blue;
unsigned char green;
unsigned char red;
} Pixel;
typedef struct {
int width;
int height;
Pixel **pixels;
} BMPImage;
BMPImage* readBMPImage(char* filename) {
FILE* file = fopen(filename, "rb");
if (file == NULL) {
printf("Failed to open the file.\n");
return NULL;
}
unsigned char header[54];
fread(header, sizeof(unsigned char), 54, file);
int width = *(int*)&header[18];
int height = *(int*)&header[22];
int numPixels = width * height;
int dataSize = numPixels * 3;
unsigned char* data = (unsigned char*)malloc(dataSize * sizeof(unsigned char));
fread(data, sizeof(unsigned char), dataSize, file);
fclose(file);
BMPImage* image = (BMPImage*)malloc(sizeof(BMPImage));
image->width = width;
image->height = height;
image->pixels = (Pixel**)malloc(height * sizeof(Pixel*));
for (int i = 0; i < height; i++) {
image->pixels[i] = (Pixel*)malloc(width * sizeof(Pixel));
for (int j = 0; j < width; j++) {
int index = i * width + j;
image->pixels[i][j].blue = data[index * 3];
image->pixels[i][j].green = data[index * 3 + 1];
image->pixels[i][j].red = data[index * 3 + 2];
}
}
free(data);
return image;
}
void invertBMPImage(BMPImage* image) {
for (int i = 0; i < image->height; i++) {
for (int j = 0; j < image->width; j++) {
image->pixels[i][j].blue = 255 - image->pixels[i][j].blue;
image->pixels[i][j].green = 255 - image->pixels[i][j].green;
image->pixels[i][j].red = 255 - image->pixels[i][j].red;
}
}
}
void saveBMPImage(BMPImage* image, char* filename) {
FILE* file = fopen(filename, "wb");
if (file == NULL) {
printf("Failed to create the file.\n");
return;
}
unsigned char header[54] = {
0x42, 0x4D, 0x38, 0x6C, 0x07, 0x00, 0x00, 0x00,
0x00, 0x00, 0x36, 0x00, 0x00, 0x00, 0x28, 0x00,
0x00, 0x00, 0x64, 0x00, 0x00, 0x00, 0x58, 0xFB,
0xFF, 0xFF, 0x01, 0x00, 0x18, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x6C, 0x07, 0x00, 0x00, 0x12,
0x0B, 0x00, 0x00, 0x12, 0x0B, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00
};
*(int*)&header[2] = 54 + image->width * image->height * 3;
*(int*)&header[18] = image->width;
*(int*)&header[22] = image->height;
fwrite(header, sizeof(unsigned char), 54, file);
for (int i = 0; i < image->height; i++) {
for (int j = 0; j < image->width; j++) {
fwrite(&image->pixels[i][j].blue, sizeof(unsigned char), 1, file);
fwrite(&image->pixels[i][j].green, sizeof(unsigned char), 1, file);
fwrite(&image->pixels[i][j].red, sizeof(unsigned char), 1, file);
}
}
fclose(file);
}
int main() {
BMPImage* image = readBMPImage("input.bmp");
invertBMPImage(image);
saveBMPImage(image, "output.bmp");
for (int i = 0; i < image->height; i++) {
free(image->pixels[i]);
}
free(image->pixels);
free(image);
return 0;
}
### 回答3:
#include <stdio.h>
#include <stdlib.h>
#pragma pack(push) // 保存对齐状态
#pragma pack(2) // 设定对齐字节为2
// BMP文件头
typedef struct
{
unsigned short bfType; // 文件类型,必须为BM
unsigned int bfSize; // 文件大小,单位为字节
unsigned short bfReserved1; // 保留字段,必须为0
unsigned short bfReserved2; // 保留字段,必须为0
unsigned int bfOffBits; // 从文件头到像素数据的偏移量
} BMPFileHeader;
// BMP信息头
typedef struct
{
unsigned int biSize; // 信息头大小,必须为40
unsigned int biWidth; // 图像宽度,单位为像素
unsigned int biHeight; // 图像高度,单位为像素
unsigned short biPlanes; // 目标设备的平面数,必须为1
unsigned short biBitCount; // 每个像素的位数,支持24位和8位
unsigned int biCompression; // 压缩类型,必须为0
unsigned int biSizeImage; // 图像大小,单位为字节
unsigned int biXPelsPerMeter; // 水平分辨率,单位为像素每米
unsigned int biYPelsPerMeter; // 垂直分辨率,单位为像素每米
unsigned int biClrUsed; // 实际使用的颜色表中的颜色数
unsigned int biClrImportant; // 对图像显示有重要影响的索引数
} BMPInfoHeader;
#pragma pack(pop) // 恢复对齐状态
// 反色处理
void invertColors(unsigned char* imageData, unsigned int imageSize)
{
for (unsigned int i = 0; i < imageSize; i++)
{
imageData[i] = ~imageData[i];
}
}
int main()
{
FILE* file = fopen("input.bmp", "rb"); // 打开BMP文件
if (file == NULL)
{
printf("文件打开失败。\n");
return 0;
}
BMPFileHeader fileHeader;
BMPInfoHeader infoHeader;
fread(&fileHeader, sizeof(BMPFileHeader), 1, file); // 读取文件头
fread(&infoHeader, sizeof(BMPInfoHeader), 1, file); // 读取信息头
unsigned int imageSize = infoHeader.biSizeImage;
unsigned char* imageData = (unsigned char*)malloc(imageSize); // 分配内存
fread(imageData, sizeof(unsigned char), imageSize, file); // 读取图像数据
fclose(file); // 关闭文件
invertColors(imageData, imageSize); // 反色处理
file = fopen("output.bmp", "wb"); // 创建输出BMP文件
if (file == NULL)
{
printf("文件创建失败。\n");
free(imageData);
return 0;
}
fwrite(&fileHeader, sizeof(BMPFileHeader), 1, file); // 写入文件头
fwrite(&infoHeader, sizeof(BMPInfoHeader), 1, file); // 写入信息头
fwrite(imageData, sizeof(unsigned char), imageSize, file); // 写入图像数据
fclose(file); // 关闭文件
free(imageData); // 释放内存
printf("图像处理完成。\n");
return 0;
}
阅读全文
相关推荐
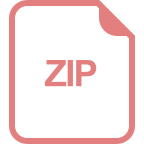







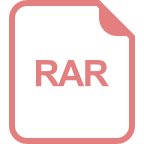
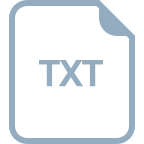
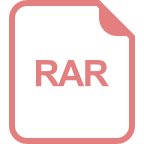
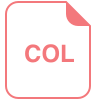
