immatrix1 = array([array(Image.open(imname1)).flatten() for imname1 in imlist1], 'f')
时间: 2024-06-01 07:11:03 浏览: 82
This code creates a 2D array called "immatrix1" where each row corresponds to an image and each column corresponds to a pixel value. It does this by iterating through a list of image names "imlist1", opening each image with the PIL (Python Imaging Library) "Image.open" function, flattening the image into a 1D array with the "flatten" method, and converting each element to a float datatype ('f'). The resulting array can be used for image processing and analysis.
相关问题
# Save PointMap to pm_path. def SavePlyFile(self,pm_path, pm, image): width = pm.GetSize().width height = pm.GetSize().height pm_num = width * height pm_ptr = np.array(pm, copy=False).reshape((-1, 3)) image_ptr = np.array(image, copy=False) if image.GetType() == RVC.ImageTypeEnum.Mono8: tmp = image_ptr.copy().flatten() image_ptr = np.zeros((height * width, 3)) image_ptr[:, 0] = tmp image_ptr[:, 1] = tmp image_ptr[:, 2] = tmp else: image_ptr = image_ptr.reshape(-1, 3) if image.GetType() == RVC.ImageTypeEnum.BGR8: image_ptr[:, [0, 2]] = image_ptr[:, [2, 0]] data = np.concatenate((pm_ptr, image_ptr), axis=1) np.savetxt(pm_path, data, fmt = '%f %f %f %d %d %d') with open(pm_path, 'r+') as f: old = f.read() f.seek(0) f.write("{}".format("ply")) f.write("\n{}".format("format ascii 1.0")) f.write("\n{}".format("comment Created by Rvbust, Inc")) f.write("\nelement vertex {}".format(pm_num)) f.write("\n{}".format("property float x")) f.write("\n{}".format("property float y")) f.write("\n{}".format("property float z")) f.write("\n{}".format("property uchar red")) f.write("\n{}".format("property uchar green")) f.write("\n{}".format("property uchar blue")) f.write("\n{}\n".format("end_header")) f.write(old) f.close() return
这段代码是用来将一个 PointMap 和一张图片保存为一个 .ply 文件的函数。具体来说,它接受三个参数:pm_path 表示保存的路径,pm 表示 PointMap 对象,image 表示图片对象。
首先,它通过 pm.GetSize() 获取 PointMap 的宽高信息,计算出 PointMap 中点的数量。然后,它将 PointMap 转换为一个二维 numpy 数组 pm_ptr,将图片转换为一个三维 numpy 数组 image_ptr。
接下来,它根据图片的类型进行判断。如果是 Mono8 类型,表示图片是单通道灰度图像,那么它将其转换为一个三通道数组,其中三个通道的值都相等。如果是其他类型,那么就将其转换为一个二维的三通道数组,并将 BGR 通道顺序转换为 RGB。
最后,它将 pm_ptr 和 image_ptr 按列连接成一个大的 numpy 数组 data,并将其写入到 pm_path 指定的文件中。在写入之前,它先写入了一个 .ply 文件的头部信息,包括文件格式、版本、注释、点的数量和属性等。最后,它将原文件中的内容追加到新写入的内容之后,完成文件的保存。
import numpy as np import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Flatten from tensorflow.keras.callbacks import EarlyStopping from PIL import Image # 加载数据集 X = [] y = [] for i in range(1000): img = Image.open(f"train/cat.{i}.jpg") img = img.resize((150, 150)) X.append(np.array(img)) y.append(0) for i in range(1000): img = Image.open(f"train/dog.{i}.jpg") img = img.resize((150, 150)) X.append(np.array(img)) y.append(1) X = np.array(X) y = np.array(y) # 划分训练集和测试集 X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # 构建模型 model = Sequential([ Flatten(input_shape=(150, 150, 3)), Dense(128, activation='relu'), Dense(1, activation='sigmoid') ]) # 编译模型 model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # 训练模型 early_stopping = EarlyStopping(monitor='val_loss', patience=3) model.fit(X_train, y_train, epochs=10, validation_data=(X_test, y_test), callbacks=[early_stopping]) # 计算准确率 loss, acc = model.evaluate(X_test, y_test) print(f"Accuracy: {acc}")
这段代码是一个使用卷积神经网络做猫狗分类的例子。
首先,代码通过PIL库加载了一千张猫和一千张狗的图片,并将它们缩放成150x150像素的大小。然后将图片数据存储到数组X中,将标签(0表示猫,1表示狗)存储到数组y中。
接下来,使用train_test_split函数将数据集划分为训练集和测试集。
然后,使用Sequential模型构建了一个简单的卷积神经网络模型。这个模型包含了一个Flatten层(将图片数据展开成一维向量)、一个Dense层、和一个输出层。其中Dense层使用了ReLU激活函数,输出层使用了sigmoid激活函数,用于输出分类结果(猫或狗)的概率。
模型中使用了Adam优化器和二元交叉熵损失函数,并用accuracy评估了模型的性能。
在训练模型时,使用了EarlyStopping回调函数,以防止过拟合。
最后,代码计算并打印了模型在测试集上的准确率。
阅读全文
相关推荐
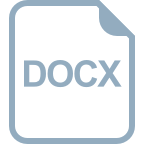
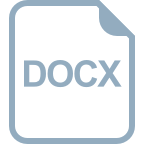
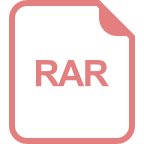
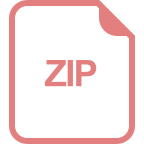
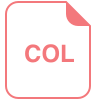
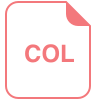










