用html+css+js编写一个音乐网站
时间: 2023-09-18 18:11:41 浏览: 120
很好,以下是简单的步骤:
1. 首先创建一个 HTML 文件,命名为 index.html。在文件中添加一个 head 和 body 标签,以及一个导航栏和一个 footer。
2. 在 head 标签中添加一个标题和一个链接到你喜欢的字体库。你可以使用 Google 字体库,如下所示:
```html
<head>
<title>My Music Site</title>
<link href="https://fonts.googleapis.com/css?family=Open+Sans" rel="stylesheet">
</head>
```
3. 在 body 标签中创建一个 div,用于包含所有的内容。在这个 div 中添加一个标题和一个搜索栏。
```html
<body>
<div class="container">
<h1>My Music Site</h1>
<form>
<input type="text" placeholder="Search for music...">
<button type="submit">Search</button>
</form>
</div>
</body>
```
4. 接下来,添加一些样式到你的网页中。在 head 标签中添加一个样式标签,并为你的元素添加样式:
```html
<style>
body {
margin: 0;
padding: 0;
font-family: 'Open Sans', sans-serif;
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
h1 {
text-align: center;
}
form {
display: flex;
margin-top: 20px;
}
input[type="text"] {
flex: 1;
border: none;
padding: 10px;
font-size: 16px;
}
button[type="submit"] {
background-color: #F44336;
border: none;
color: white;
padding: 12px 20px;
font-size: 16px;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: #FFC107;
}
</style>
```
5. 现在,我们需要添加一些音乐到我们的网站中。你可以使用 HTML5 的音频标签来嵌入音频。
```html
<audio src="music.mp3" controls></audio>
```
6. 在你的容器 div 中添加一些音乐。你可以使用一个表格来列出你的音乐,并为每一行添加一个音频标签。
```html
<table>
<thead>
<tr>
<th>Title</th>
<th>Artist</th>
<th>Album</th>
<th>Length</th>
</tr>
</thead>
<tbody>
<tr>
<td><audio src="music1.mp3" controls></audio></td>
<td>Artist 1</td>
<td>Album 1</td>
<td>3:45</td>
</tr>
<tr>
<td><audio src="music2.mp3" controls></audio></td>
<td>Artist 2</td>
<td>Album 2</td>
<td>4:23</td>
</tr>
<tr>
<td><audio src="music3.mp3" controls></audio></td>
<td>Artist 3</td>
<td>Album 3</td>
<td>3:12</td>
</tr>
</tbody>
</table>
```
7. 最后,我们需要添加一些 JavaScript 代码来使我们的搜索栏工作。当用户提交表单时,我们将使用 JavaScript 过滤我们的音乐列表。
```html
<script>
const form = document.querySelector('form');
const input = document.querySelector('input[type="text"]');
const table = document.querySelector('table');
form.addEventListener('submit', (event) => {
event.preventDefault();
const searchTerm = input.value.toLowerCase();
for (let i = 1; i < table.rows.length; i++) {
const title = table.rows[i].cells[0].innerText.toLowerCase();
const artist = table.rows[i].cells[1].innerText.toLowerCase();
const album = table.rows[i].cells[2].innerText.toLowerCase();
if (title.indexOf(searchTerm) > -1 || artist.indexOf(searchTerm) > -1 || album.indexOf(searchTerm) > -1) {
table.rows[i].style.display = '';
} else {
table.rows[i].style.display = 'none';
}
}
});
</script>
```
现在你就可以打开你的网站并享受听歌的乐趣了!
阅读全文
相关推荐
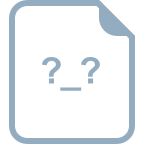

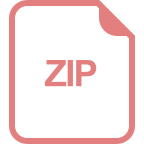
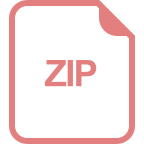
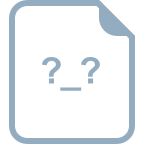
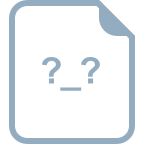
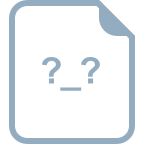
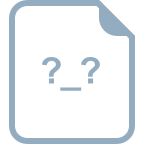
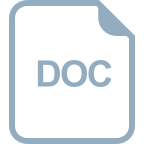
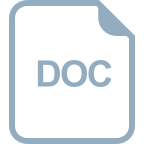
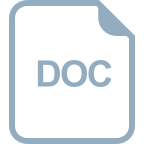
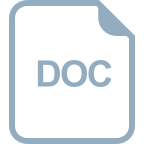
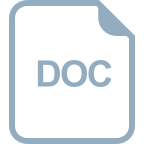
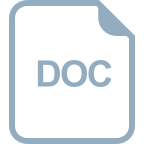
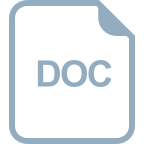
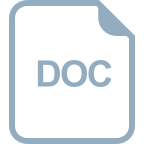
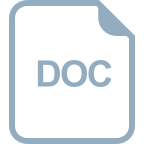